There are two types of devs in the world right now…
Those still debugging manually, trying to decipher someone else’s spaghetti code while Googling StackOverflow answers written in 2013…
And those using Cursor.
Cursor isn’t just another VSCode fork. It’s a full-blown assistant that thinks in code. An AI-native editor that writes for you, explains logic instantly, and helps you debug in real time, with surgical accuracy.
No fluff. No lag.
Just clean, intelligent code collaboration between you… and an AI that actually knows what you’re trying to do.
Here’s the truth…
If you’re still coding without Cursor, you’re playing the game on hard mode.
Because the devs using Cursor? They’re shipping faster. Fixing bugs in seconds. And rewriting legacy codebases without touching a single line themselves.
So the question isn’t if you’ll switch.
It’s when.
And the longer you wait… the further behind you fall.
Cursor is a powerful code editor built on VSCode that integrates AI capabilities to help you write, understand, and debug code more efficiently.
Getting Started with Cursor
First, download and install Cursor from cursor.so. After installation, you’ll find an interface similar to VSCode but with additional AI features.
Basic Code Generation
Generating Simple Functions
To generate a Python function, you can describe what you want in a comment and use the /generate
command:
# Create a function that calculates the factorial of a number
def factorial(n):
if n == 0 or n == 1:
return 1
else:
return n * factorial(n-1)
Generating Classes
You can generate entire classes by describing their purpose:
# Create a Student class that tracks name, ID, and grades
class Student:
def __init__(self, name, student_id):
self.name = name
self.student_id = student_id
self.grades = []
def add_grade(self, grade):
self.grades.append(grade)
def get_average(self):
if not self.grades:
return 0
return sum(self.grades) / len(self.grades)
def __str__(self):
return f"Student: {self.name} (ID: {self.student_id})"
Code Generation Techniques
Data Processing Scripts
Cursor excels at generating data processing code. For example:
# Generate a function to read a CSV file, filter rows where age > 30, and save to a new file
import pandas as pd
def filter_csv_by_age(input_file, output_file, min_age=30):
# Read the CSV file
df = pd.read_csv(input_file)
# Filter rows where age > min_age
filtered_df = df[df['age'] > min_age]
# Save to a new file
filtered_df.to_csv(output_file, index=False)
return f"Filtered {len(df) - len(filtered_df)} rows. Saved {len(filtered_df)} rows to {output_file}"
API Integrations
Cursor can help you generate code for API integrations:
# Create a function to fetch weather data from OpenWeatherMap API
import requests
def get_weather(city, api_key):
base_url = "https://api.openweathermap.org/data/2.5/weather"
params = {
"q": city,
"appid": api_key,
"units": "metric"
}
response = requests.get(base_url, params=params)
if response.status_code == 200:
data = response.json()
return {
"city": city,
"temperature": data["main"]["temp"],
"description": data["weather"][0]["description"],
"humidity": data["main"]["humidity"],
"wind_speed": data["wind"]["speed"]
}
else:
return {"error": f"Error fetching weather data: {response.status_code}"}
Using Chat for Code Generation
Cursor has a chat interface that you can use to generate code interactively. Press Ctrl+L
(or Cmd+L
on Mac) to open the chat panel.
Example chat prompt: “Generate a Python function that takes a list of dictionaries containing product information (name, price, quantity) and returns the total inventory value.”
def calculate_inventory_value(products):
"""
Calculate the total value of inventory from a list of product dictionaries.
Args:
products (list): List of dictionaries with keys 'name', 'price', and 'quantity'
Returns:
float: Total inventory value
"""
total_value = 0
for product in products:
product_value = product['price'] * product['quantity']
total_value += product_value
return total_value
# Example usage
inventory = [
{"name": "Laptop", "price": 1200, "quantity": 5},
{"name": "Mouse", "price": 25, "quantity": 20},
{"name": "Keyboard", "price": 45, "quantity": 15}
]
total = calculate_inventory_value(inventory)
print(f"Total inventory value: ${total}")
Generating Complex Algorithms
Cursor can help with more complex algorithms too:
# Generate a function to implement the Dijkstra algorithm for finding shortest paths in a graph
import heapq
def dijkstra(graph, start):
"""
Implements Dijkstra’s algorithm to find shortest paths from start node to all other nodes.
Args:
graph (dict): Dictionary where keys are nodes and values are dictionaries of neighbors and distances
start: Starting node
Returns:
dict: Dictionary of shortest distances from start to each node
"""
# Initialize distances with infinity for all nodes except the start node
distances = {node: float('infinity') for node in graph}
distances[start] = 0
# Priority queue to keep track of nodes to visit
priority_queue = [(0, start)]
while priority_queue:
current_distance, current_node = heapq.heappop(priority_queue)
# If we've already found a shorter path, skip
if current_distance > distances[current_node]:
continue
# Check all neighbors of the current node
for neighbor, weight in graph[current_node].items():
distance = current_distance + weight
# If we found a shorter path to the neighbor
if distance < distances[neighbor]:
distances[neighbor] = distance
heapq.heappush(priority_queue, (distance, neighbor))
return distances
Generating Web Applications
Cursor can help you generate code for web applications using frameworks like Flask:
# Create a simple Flask API for a todo list
from flask import Flask, request, jsonify
app = Flask(__name__)
# In-memory storage for todos
todos = []
@app.route('/todos', methods=['GET'])
def get_todos():
return jsonify(todos)
@app.route('/todos', methods=['POST'])
def add_todo():
data = request.get_json()
if 'task' not in data:
return jsonify({"error": "Task is required"}), 400
todo = {
"id": len(todos) + 1,
"task": data['task'],
"completed": False
}
todos.append(todo)
return jsonify(todo), 201
@app.route('/todos/<int:todo_id>', methods=['PUT'])
def update_todo(todo_id):
data = request.get_json()
for todo in todos:
if todo['id'] == todo_id:
todo['task'] = data.get('task', todo['task'])
todo['completed'] = data.get('completed', todo['completed'])
return jsonify(todo)
return jsonify({"error": "Todo not found"}), 404
@app.route('/todos/<int:todo_id>', methods=['DELETE'])
def delete_todo(todo_id):
for i, todo in enumerate(todos):
if todo['id'] == todo_id:
del todos[i]
return jsonify({"message": "Todo deleted"})
return jsonify({"error": "Todo not found"}), 404
if __name__ == '__main__':
app.run(debug=True)
Data Analysis and Visualization
Cursor can generate data analysis and visualization code:
# Generate code to analyze and visualize a dataset of house prices
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
from sklearn.linear_model import LinearRegression
from sklearn.model_selection import train_test_split
def analyze_house_prices(csv_file):
# Load the dataset
df = pd.read_csv(csv_file)
# Display basic statistics
print("Dataset shape:", df.shape)
print("\nBasic statistics:")
print(df.describe())
# Check for missing values
print("\nMissing values:")
print(df.isnull().sum())
# Create visualizations
plt.figure(figsize=(12, 8))
# Price distribution
plt.subplot(2, 2, 1)
sns.histplot(df['price'])
plt.title('Price Distribution')
# Price vs. Square Footage
plt.subplot(2, 2, 2)
sns.scatterplot(x='sqft_living', y='price', data=df)
plt.title('Price vs. Square Footage')
# Price by number of bedrooms
plt.subplot(2, 2, 3)
sns.boxplot(x='bedrooms', y='price', data=df)
plt.title('Price by Bedrooms')
# Correlation heatmap
plt.subplot(2, 2, 4)
numeric_df = df.select_dtypes(include=['float64', 'int64'])
sns.heatmap(numeric_df.corr(), annot=True, cmap='coolwarm', fmt='.2f')
plt.title('Correlation Heatmap')
plt.tight_layout()
plt.savefig('house_price_analysis.png')
# Simple linear regression model
X = df[['sqft_living']]
y = df['price']
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
model = LinearRegression()
model.fit(X_train, y_train)
print(f"\nLinear Regression Results:")
print(f"Coefficient: {model.coef_[0]:.2f}")
print(f"Intercept: {model.intercept_:.2f}")
print(f"R² Score: {model.score(X_test, y_test):.4f}")
return df
Tips for Better Code Generation
Be specific in your prompts: The more details you provide, the better the generated code will match your needs.
Use incremental generation: Start with a basic structure and then ask Cursor to add specific features.
Provide examples: If you have specific formatting or style requirements, include examples in your prompt.
Edit and regenerate: Don’t hesitate to edit the generated code and ask Cursor to regenerate parts that need improvement.
Use the chat interface for complex requirements: The chat interface allows for more detailed conversations about your code needs.
Debugging Generated Code
Cursor can also help debug code it generates. If you encounter an error, highlight the problematic code and use the /fix
command:
# This function has a bug, let's fix it
def calculate_average(numbers):
total = 0
for num in numbers:
total += num
return total / len(numbers) # This will raise a ZeroDivisionError if numbers is empty
# Fixed version
def calculate_average(numbers):
if not numbers:
return 0
total = 0
for num in numbers:
total += num
return total / len(numbers)
Cursor is a powerful tool for generating Python code that can significantly speed up your development process. By providing clear prompts and iteratively refining the generated code, you can leverage Cursor’s AI capabilities to write everything from simple functions to complex applications. The key is to be specific in your requests and to review and refine the generated code to ensure it meets your requirements.
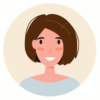
Lydia is a seasoned technical author, well-versed in the intricacies of software development and a dedicated practitioner of Python. With a career spanning 16 years, Lydia has made significant contributions as a programmer and scrum master at renowned companies such as Thompsons, Deloit, and The GAP, where they have been instrumental in delivering successful projects.
A proud alumnus of Duke University, Lydia pursued a degree in Computer Science, solidifying their academic foundation. At Duke, they gained a comprehensive understanding of computer systems, algorithms, and programming languages, which paved the way for their career in the ever-evolving field of software development.
As a technical author, Lydia remains committed to fostering knowledge sharing and promoting the growth of the computer science community. Their dedication to Python development, coupled with their expertise as a programmer and scrum master, positions them as a trusted source of guidance and insight. Through their publications and engagements, Lydia continues to inspire and empower fellow technologists, leaving an indelible mark on the world of scientific computer science.