One crucial aspect of data science is selecting the right programming language to build data solutions. This is where Python comes in – a versatile and powerful tool that can deliver expert data science solutions efficiently.
Python offers a range of benefits for building data solutions, including flexible syntax, scalability, and extensive libraries and frameworks for data analysis. Python enables developers and data scientists alike to leverage data and gain intelligent insights for efficient decision-making.
Whether you are an experienced developer or a newcomer, building data solutions with Python requires the right approach.
Python: A Powerhouse for Data Science Solutions
Python is a high-level programming language that has gained immense popularity in data science due to its simplicity, ease of use, and powerful libraries and frameworks. With Python, developers can build intelligent data solutions that enable businesses to make data-driven decisions.
The readability of Python code and its dynamic nature make it accessible to professionals from various backgrounds. Its extensive libraries and frameworks, including Pandas, NumPy, and SciPy, provide powerful tools for data manipulation, numerical computations, and scientific computing. Python’s flexibility and scalability mean that it can handle large datasets and complex computations efficiently.
Let’s take a look at some examples that illustrate Python’s capabilities in handling data science tasks:
Data Manipulation:
# import Pandas library
import pandas as pd
# read a CSV file
data = pd.read_csv('data.csv')
# select specific columns
subset = data[['column1', 'column2']]
# filter rows based on a condition
filtered_data = data[data['column1'] > 100]
The example code above shows how Python’s Pandas library can be used to manipulate data by reading a CSV file, selecting specific columns, and filtering rows based on a condition.
Statistical Analysis:
# import NumPy library
import numpy as np
# create a NumPy array
data = np.array([1, 2, 3, 4, 5])
# calculate mean and standard deviation
mean = np.mean(data)
std_dev = np.std(data)
The example code above demonstrates how Python’s NumPy library can be used to perform statistical analysis by calculating the mean and standard deviation of a dataset.
Machine Learning:
# import Scikit-Learn library
from sklearn.linear_model import LinearRegression
# create a linear regression model
model = LinearRegression()
# train the model
model.fit(X_train, y_train)
# make predictions on test data
predictions = model.predict(X_test)
The example code above showcases how Python’s Scikit-Learn library can be used for machine learning tasks by creating a linear regression model, training it on data, and making predictions on test data.
Python’s strength in data science is evident, but how does Python compare with other programming languages commonly used in this field, such as R or Julia? Let’s explore that in the next section.
Python Data Science Libraries and Frameworks
Python provides a vast range of libraries and frameworks for data analysis, manipulation, and visualization. These libraries and frameworks are designed to simplify data processing tasks, enabling professionals to focus more on the analytical aspects of their projects. Here are some of the most popular Python data science libraries and frameworks:
Library/Framework | Functionality |
---|---|
Pandas | Provides flexible data structures to handle large and complex datasets, including support for data import/export, data cleaning, and data transformation. |
NumPy | Provides efficient numerical operations and mathematical functions for scientific computing, including support for linear algebra, Fourier analysis, and random number generation. |
SciPy | Provides a comprehensive set of algorithms for optimization, integration, interpolation, and signal processing, among other scientific computing tasks. |
Matplotlib | Provides a wide range of visualization tools for creating static, animated, or interactive plots and charts, including support for 2D and 3D plotting, subplots, and annotations. |
Seaborn | Provides additional visualization tools for statistical data analysis and modeling, including support for heatmaps, pair plots, and distribution plots. |
Each library or framework has its strengths and weaknesses, depending on the specific data science task at hand. For instance, Pandas is usually preferred for working with tabular datasets and time-series data due to its powerful data manipulation capabilities.
On the other hand, NumPy is ideal for handling large arrays and matrices, providing fast and memory-efficient operations on these data structures. Meanwhile, Matplotlib and Seaborn are widely used for creating high-quality visualizations that can help reveal patterns and trends in data.
While there are several other Python data science libraries and frameworks available, mastering these essential tools will go a long way in improving one’s data science skills and capabilities.
Building Intelligent Data Solutions with Python
Python’s versatility and extensive libraries make it a valuable tool for developing intelligent data solutions. Whether it’s developing predictive models or data-driven applications, Python provides a flexible and scalable environment for implementing data science solutions.
One important aspect of building intelligent data solutions with Python is data preprocessing. This involves transforming raw data into a clean, structured format that can be analyzed and used to train machine learning models. Fortunately, Python offers various libraries such as Pandas and NumPy, which provide powerful functionalities for data manipulation and numerical computations.
Another important concept in building intelligent data solutions is feature engineering. This involves selecting, extracting, and transforming the relevant features of the data to optimize the performance of the predictive models. Python libraries such as Scikit-learn offer easy-to-use tools for feature engineering, simplifying the process of selecting the appropriate features for the model.
Pandas Example: | Scikit-learn Example: |
---|---|
import pandas as pd | from sklearn.feature_extraction.text import CountVectorizer |
Finally, Python makes it easy to select the appropriate model for the data and optimize its performance. Whether it’s a simple linear regression or a complex deep learning model, Python libraries such as TensorFlow and PyTorch provide an extensive range of tools for building and training machine learning models.
Overall, building intelligent data solutions with Python involves leveraging the programming language’s extensive libraries and frameworks, and using best practices for data preprocessing, feature engineering, and model selection.
Industry Examples of Python Data Science Solutions
Python has been widely applied across various industries to develop intelligent data solutions that facilitate decision making and optimization. Here are some examples of industries that have successfully leveraged Python data science solutions:
- Finance: Financial institutions use Python for risk management, portfolio optimization, fraud detection, and trading strategies. Goldman Sachs, for instance, has its own open-source platform for quantitative finance, called Quantlib-Python, which is based on Python.
- Healthcare: Python is used for medical analysis and research, drug discovery, and patient monitoring. For instance, the National Institute of Health (NIH) uses Python for genome analysis and visualization.
- Retail: Retail companies use Python for customer analytics, inventory management, and supply chain optimization. Walmart, for example, has a Python-based platform called Databricks, which enables real-time data streaming and analysis.
- Manufacturing: Python is used for production planning, quality control, and predictive maintenance. General Electric (GE) has developed a Python-based platform called Predix, which uses machine learning for industrial analytics.
These are just a few examples; in reality, Python data science solutions are applicable to nearly any industry or sector, depending on the specific needs and challenges.
Python vs. Other Languages for Data Science
When it comes to data science, Python has become the go-to programming language for many professionals. But how does it compare to other popular languages like R or Julia?
Python vs. R
Python and R are both popular languages for data science, but they have some key differences. One advantage of Python is its versatility; it can be used for a wide range of tasks beyond data analysis. Additionally, Python has a larger community and more extensive libraries and frameworks for data science. On the other hand, R is designed specifically for statistical analysis and has better built-in capabilities for data visualization.
Let’s compare the syntax of Python and R for performing a common data manipulation task:
Python | R |
---|---|
df.dropna() | na.omit(df) |
As you can see, Python’s syntax is more intuitive and readable, making it accessible for professionals from various backgrounds.
Python vs. Julia
Julia is a newer language in the data science scene that has gained popularity for its speed and performance. However, Python still has some advantages in terms of community support and versatility. Additionally, Python has more mature and developed libraries for machine learning and deep learning.
Let’s compare the syntax of Python and Julia for implementing a simple machine learning algorithm:
Python | Julia |
---|---|
from sklearn.ensemble import RandomForestClassifier | using ScikitLearn |
Again, Python’s syntax is more intuitive and easier to read, making it more accessible for professionals with different backgrounds.
While each language has its own strengths and weaknesses, Python’s versatility and large community make it a powerhouse for data science solutions, especially for those looking to leverage Python for intelligent data solutions.
Best Practices for Expert Python Data Science Solutions
Developing data science solutions with Python can be challenging without a set of best practices to follow. Here are some expert tips for achieving efficient, maintainable, and reliable Python data science projects:
- Start with Data Cleaning: Cleaning and preprocessing data is a crucial step for accurate analysis and modeling. Use libraries like Pandas to handle missing values, outliers, or duplicates, and ensure data consistency and quality.
- Explore Data with EDA: Exploratory Data Analysis (EDA) helps to understand data distributions, relationships, and patterns. Use visualizations and statistical methods in libraries like Matplotlib or Seaborn to gain insights and generate hypotheses.
- Choose the Right Model: Selecting the right machine learning model for your data is key for achieving good performance. Experiment with different algorithms and techniques in libraries like Scikit-Learn or TensorFlow, and validate their accuracy and generalizability.
- Validate Models with Cross-Validation: Testing model accuracy on the same data used for training can lead to overfitting. Use cross-validation techniques like K-fold or Stratified sampling to estimate model performance on unseen data.
- Document Your Code: Writing clear and concise documentation for your code is important for future maintenance and collaboration. Use comments and docstrings to describe the purpose, inputs, and outputs of your functions or modules.
- Optimize Your Code: Data science solutions can involve processing large amounts of data, and therefore, require efficient algorithms and code structures. Use libraries like NumPy or Cython to speed up computations and reduce memory usage.
- Collaborate with Git: Version control is essential for managing changes, tracking bugs, and collaborating with others. Use Git to store your code, create branches for experiments or features, and share your work with the community.
By following these best practices, you can ensure the quality and effectiveness of your Python data science solutions and become an expert in the field.
Challenges and Future Trends in Python Data Science
Python has established itself as a powerful tool for data science solutions. Yet, as with any technology, there are challenges to be aware of when working with data.
Challenges
One key challenge in Python data science is working with large datasets. Python’s memory footprint can be a limitation when dealing with datasets which are larger than your available memory. Memory optimization techniques like chunking, compression, and data streaming can be used to overcome this issue.
Another challenge involves scalability. While Python can be used to develop scalable data solutions, the language itself is limited by its interpretation and global interpreter lock. Choosing the right tools and frameworks, like PySpark or Dask, can help address these limitations.
Finally, deep learning techniques present a unique challenge in Python data science. TensorFlow, Keras, and PyTorch are popular frameworks for deep learning in Python, but they can also be computationally expensive and require specialized hardware such as GPUs to run effectively.
Conclusion: Unlock the Power of Python Data Science Solutions
Python has revolutionized the field of data science, providing professionals from diverse backgrounds with a powerful and versatile programming language to build intelligent data solutions. By leveraging Python’s extensive libraries and frameworks, businesses can gain valuable insights, optimize processes, and stay ahead of the curve in their respective industries.
When building Python data science solutions, it is important to follow best practices to ensure efficiency, maintainability, and reliability. This can include data cleaning, exploratory data analysis, and model validation, as well as proper documentation.
Despite its many strengths, Python does face challenges when working with large datasets or incorporating deep learning techniques. However, as the field of data science continues to evolve, Python is likely to remain at the forefront of innovation and drive the next generation of intelligent data solutions.
Unlock the power of Python data science solutions today by exploring further resources, courses, and certifications to enhance your skills and stay up to date with emerging trends.
FAQ
Q: What is data science?
A: Data science is the field that involves extracting insights and knowledge from large amounts of data using various scientific methods, algorithms, and processes.
Q: Why is Python considered a powerful tool for data science solutions?
A: Python is highly regarded in the data science community due to its simplicity, readability, and extensive libraries and frameworks specifically designed for data analysis, manipulation, and machine learning.
Q: What are some popular Python libraries and frameworks used in data science?
A: Some widely used Python libraries and frameworks in data science include Pandas, NumPy, and SciPy. These tools provide powerful functionalities for data manipulation, numerical computations, and scientific computing.
Q: How can Python be leveraged to build intelligent data solutions?
A: Python can be used to build intelligent data solutions by facilitating tasks such as data preprocessing, feature engineering, and model selection. It provides a versatile and flexible environment for developing predictive models and data-driven applications.
Q: How does Python compare to other languages commonly used in data science, like R or Julia?
A: Python offers advantages such as a large and active community, versatility, and easy integration with other technologies. However, each language has its own strengths and weaknesses, so the choice depends on the specific requirements and preferences of the project.
Q: What are some best practices for developing Python data science solutions?
A: Some best practices include thorough data cleaning, exploratory data analysis, proper model validation, and comprehensive documentation. Following these practices can improve efficiency, maintainability, and reliability in data science projects.
Q: What are the challenges and future trends in Python data science?
A: Challenges in Python data science include scalability issues with large datasets and incorporating deep learning techniques. Future trends include the integration of big data technologies and the use of Python in conjunction with cloud platforms.
Q: How can I unlock the power of Python data science solutions?
A: To unlock the power of Python data science solutions, it is essential to gain a strong understanding of Python’s libraries and frameworks for data analysis and machine learning. Exploring further resources, such as courses or certifications, can enhance your Python data science skills.
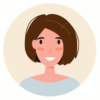
Lydia is a seasoned technical author, well-versed in the intricacies of software development and a dedicated practitioner of Python. With a career spanning 16 years, Lydia has made significant contributions as a programmer and scrum master at renowned companies such as Thompsons, Deloit, and The GAP, where they have been instrumental in delivering successful projects.
A proud alumnus of Duke University, Lydia pursued a degree in Computer Science, solidifying their academic foundation. At Duke, they gained a comprehensive understanding of computer systems, algorithms, and programming languages, which paved the way for their career in the ever-evolving field of software development.
As a technical author, Lydia remains committed to fostering knowledge sharing and promoting the growth of the computer science community. Their dedication to Python development, coupled with their expertise as a programmer and scrum master, positions them as a trusted source of guidance and insight. Through their publications and engagements, Lydia continues to inspire and empower fellow technologists, leaving an indelible mark on the world of scientific computer science.