What are the Advanced Python Skills Some Developers Have? Embrace advanced OOP principles, apply intricate design patterns, and continuously evolve to stay atop the tech innovation ladder.
In today’s fast-paced digital landscape, developers need to possess a wide range of skills to stay ahead. While basic Python knowledge is essential, advanced Python skills are what set certain developers apart from others.
Developers with advanced Python skills possess an in-depth understanding of the language and its features, enabling them to create innovative solutions and tackle complex coding challenges.
Key Takeaways:
- Developers with advanced Python skills can create innovative solutions and tackle complex coding challenges.
- Advanced Python skills include object-oriented programming, data structures and algorithms, concurrency and multithreading, decorators and metaclasses, generators and iterators, web development frameworks, testing, and debugging techniques.
- Mastering these skills can elevate a developer’s Python proficiency and deliver high-quality software applications.
Object-Oriented Programming (OOP)
Object-oriented programming (OOP) is a fundamental skill for developers aiming to take their Python proficiency to the next level. OOP is a programming paradigm based on the concept of “objects,” which can contain data and code that operates on that data. By dividing code into chunks of objects and classes, developers can write more organized and modular code that is easier to understand and maintain.
Python’s support for OOP makes it a powerful language for building complex software applications. The following concepts are key to mastering OOP in Python:
Concept | Description |
---|---|
Classes | Classes are templates that define the properties and behaviors of objects. |
Objects | Objects are instances of classes that contain specific data and behaviors. |
Inheritance | Inheritance allows classes to inherit properties and behaviors from other classes. |
Polymorphism | Polymorphism enables objects to take on multiple forms or types, allowing for more generic and flexible code. |
Developers can leverage these principles to design more efficient and scalable software. For instance, using classes and objects to represent different elements of a program can make the code more readable and easier to maintain.
Additionally, inheritance and polymorphism can help reduce duplicate code and increase code reuse, allowing developers to write software faster and with fewer errors.
Data Structures and Algorithms
Python is a popular programming language that is used to develop a wide range of applications, from simple scripts to complex web applications. One of the key factors that contribute to Python’s success is its rich set of data structures and algorithms that enable developers to write efficient and scalable code.
Advanced Python developers are well-versed in data structures like arrays, linked lists, stacks, queues, and trees, and have a strong understanding of various algorithmic techniques like searching, sorting, and graph traversal. These skills allow developers to optimize their code for performance and scalability, resulting in faster and more efficient programs.
One of the most important data structures in Python is the dictionary, which is a collection of key-value pairs. Dictionaries are incredibly versatile and can be used to store and retrieve data in a variety of ways. Another important data structure is the list, which is a collection of items that can be of any data type. Lists are widely used in Python programming, and many algorithms and data processing tasks can be implemented using them.
Algorithms are a set of instructions that are used to perform a specific task. Advanced Python developers are skilled in various algorithmic techniques like searching, sorting, and graph traversal, which are essential for optimizing the performance of Python programs. For example, binary search is a popular algorithm used to search for an element in a sorted list of items. Merge sort is another algorithm used to sort a list of items in ascending or descending order.
In conclusion, a solid understanding of advanced data structures and algorithms is essential for optimizing Python programs. By mastering data structures and algorithms, developers can design more efficient and scalable solutions for a wide range of applications.
Concurrency and Multithreading
Concurrency and multithreading are advanced Python skills that enable developers to write code that can execute multiple tasks simultaneously, improving performance and responsiveness. By dividing a program into smaller parts that can run independently, developers can design efficient, parallelized code.
Python offers built-in support for threads and processes, allowing developers to create multi-tasking applications. A thread is a lightweight flow of execution that can run concurrently with other threads within the same process, while a process is a separate instance of a program that runs independently from other programs.
However, writing concurrent code can be challenging, as multiple threads or processes may try to access the same resources, leading to race conditions, deadlocks, and other synchronization issues. To avoid such problems, developers must use locks, semaphores, and other synchronization mechanisms.
Concept | Description |
---|---|
Thread | A lightweight flow of execution that can run concurrently with other threads within the same process. |
Process | A separate instance of a program that runs independently from other programs. |
Lock | A synchronization mechanism that prevents multiple threads from accessing the same resource simultaneously. |
Semaphore | A synchronization mechanism that limits the number of threads that can access a resource simultaneously. |
Developers who master concurrency and multithreading can create programs that leverage the full potential of modern CPUs and improve user experience by providing faster and more responsive applications.
Decorators and Metaclasses
Decorators and metaclasses are advanced Python features that allow developers to modify the behavior of functions, classes, and instances dynamically. These techniques are based on the idea that functions and classes are objects in Python, which means they can be passed as arguments, returned from functions, and modified on-the-fly.
Decorators
A decorator is a function that takes another function as input, adds some functionality to it, and returns it as output. Decorators are typically used to modify the behavior of functions or methods, without changing their original code.
For example, a decorator can be used to add logging, caching, timing, validation, or authorization to a function, as shown below:
# Define a decorator function
def log_calls(func):
def wrapper(*args, **kwargs):
print("Calling function", func.__name__)
result = func(*args, **kwargs)
print("Function", func.__name__, "returned", result)
return wrapper
# Use the decorator to modify a function
@log_calls
def add(a, b):
return a + b
# Call the modified function
add(1, 2)
In this example, the decorator function “log_calls” takes a function “func” as input and defines a nested function “wrapper” that logs the name of “func” when it is called, and the result of “func” when it returns. The “wrapper” function then calls “func” with the same arguments using the syntax “*args, **kwargs”, which means that any number of positional and keyword arguments can be passed. Finally, the “wrapper” function returns the result of “func”.
The “@log_calls” syntax before the function “add” is a shorthand for applying the decorator to “add”, which is equivalent to writing “add = log_calls(add)”. This means that every time “add” is called, it will also call “log_calls” and perform the logging before and after the original code of “add”.
Metaclasses
A metaclass is a class that defines the behavior of other classes. Metaclasses are used to customize the creation, initialization, and behavior of classes at runtime. For example, a metaclass can be used to enforce certain rules on a class hierarchy, to add or remove attributes or methods from a class, or to generate classes automatically based on some pattern.
# Define a metaclass
class AutoInit(type):
def __new__(cls, name, bases, attrs):
if '__init__' not in attrs:
def init(self, **kwargs):
for key, value in kwargs.items():
setattr(self, key, value)
attrs['__init__'] = init
return super().__new__(cls, name, bases, attrs)
# Use the metaclass to generate a class
class Person(metaclass=AutoInit):
pass
# Create an instance of the generated class
person = Person(name="John", age=30)
In this example, the metaclass “AutoInit” defines a special method “__new__” that intercepts the creation of a new class, and inspects its attributes. If the attribute “__init__” is not defined, the metaclass generates a default initializer “init” that takes any number of keyword arguments, and sets them as attributes of the class instance using the syntax “setattr(self, key, value)”. The metaclass then assigns the “init” function to the “__init__” attribute of the class attributes, effectively modifying the behavior of the class.
The “class Person(metaclass=AutoInit)” syntax defines a new class “Person” that uses the metaclass “AutoInit” to customize its behavior. The generated class has a default initializer that takes the arguments “name” and “age”, and sets them as attributes of the “Person” instance. The syntax “person = Person(name=”John”, age=30)” creates an instance of the “Person” class with the name “John” and age 30.
Generators and Iterators
Generators and iterators provide developers with powerful techniques for working with large amounts of data efficiently. These concepts revolve around the concept of lazy evaluation, which means only computing a value when it is actually needed. This approach can save significant amounts of memory and processing time, especially when working with large datasets.
In Python, generators are special functions that use the yield keyword to return a series of values, one at a time, rather than constructing and returning a large data structure all at once. This allows for more memory-efficient code that can process data as it is generated, rather than loading all of it into memory at once.
Iterators are objects that enable looping over sequences, such as lists or tuples, and computing values on the fly. By using an iterator, developers can avoid creating unnecessary lists, saving both memory and processing time. Instead of storing the entire sequence in memory, the iterator computes the next value on the fly, iterating through the sequence one element at a time.
Python provides a number of built-in functions for working with generators and iterators, including the next() function for retrieving the next value generated by a generator, and the iter() function for creating an iterator from a sequence. Developers can also create custom iterators and generators to suit their specific needs.
Web Development Frameworks
Python has gained popularity for web development due to its versatile and comprehensive web frameworks. Python frameworks provide developers with tools and libraries to simplify web development, making it faster and more efficient than ever before.
Django
One of the most popular Python web frameworks is Django. It provides a full-stack solution for web development that includes an ORM system, templating engine, and routing system. Django’s powerful and flexible tools make it possible to build complex web applications in a short amount of time.
Pros: | Cons: |
---|---|
Admin interface for database management | Steep learning curve for beginners |
High-level of security features | Heavy resource usage |
Flask
Flask is a lightweight and flexible Python web framework that provides developers with the necessary tools to build web applications quickly. It has a minimalistic design and is very easy to use, making it a great choice for beginners.
Pros: | Cons: |
---|---|
Easy to learn and use | Not suitable for complex web applications |
Flexible and extensible | Minimalistic design can lead to less secure web applications |
Pyramid
Pyramid is a Python web framework that offers high flexibility and scalability. It allows developers to build web applications using a variety of web servers, databases, and templating languages. Pyramid’s architecture makes it easy to integrate a wide range of third-party tools and libraries, enabling developers to customize their web applications to their own specific needs.
Pros: | Cons: |
---|---|
Flexible and scalable | Lacks integrated ORM system |
Highly customizable | Requires deeper knowledge of Python web development |
Testing and Debugging Techniques
One hallmark of an advanced Python developer is their ability to thoroughly test and debug their code. By ensuring the reliability and stability of their applications, developers can deliver quality software products that meet user needs.
Python offers a range of testing frameworks that developers can use to automate the testing process and catch errors early on. Two popular testing frameworks are unittest and pytest.
Unittest is a built-in Python testing framework that provides a set of tools for constructing test cases and suites. Developers can use unittest to create comprehensive test suites for functions, modules, and even entire applications. This framework also includes a set of assertion methods that enable developers to test for specific conditions and values.
Pytest, on the other hand, is a third-party testing framework that provides a more concise and intuitive syntax for writing tests. This framework is particularly useful for developers working with complex applications and test scenarios. Pytest supports fixture-based testing, which allows developers to define and reuse setup and teardown actions across multiple tests.
In addition to testing frameworks, skilled Python developers also leverage various debugging techniques to identify and resolve issues in their code. One effective debugging technique is logging, which involves adding code to log messages that capture the state of an application during runtime. Developers can use logging messages to track the flow of their code, identify where exceptions occur, and monitor system behavior.
Another valuable debugging technique is exception handling, which involves writing code to handle errors and exceptions that occur during program execution. Developers can use try-except blocks to gracefully handle exceptions and prevent their code from crashing. By leveraging exception handling, developers can create more robust and resilient applications.
Common Problems and Solutions – What are the Advanced Python Skills Some Developers Have?
1. Problem: Overengineering Solutions
Consequences: This can result in more complex code than necessary, making it harder to maintain, debug, and causing potential performance issues.
Solution:
Stick to the Basics First: Before diving into advanced techniques, always question if a simpler solution can achieve the same goal.
Example: Before using a complex design pattern, check if a simpler OOP principle can suffice.
Quote: “Perfection is achieved, not when there is nothing more to add, but when there is nothing left to take away.” – Antoine de Saint-Exupéry
Key Takeaway: Complexity doesn’t always equate to efficiency.
2. Problem: Neglecting Pythonic Ways in Favor of Speed
Consequences: Writing non-Pythonic code can make it less readable and maintainable for other Python developers.
Solution:
Balance is Key: While performance is crucial, maintaining Pythonic ways ensures code clarity.
Example: List comprehensions can be both Pythonic and efficient, as opposed to using raw loops with appended elements.
Key Takeaway: Prioritize writing clean, Pythonic code without compromising on performance.
3. Problem: Not Keeping Up with Updates and Changes
Consequences: Falling behind in the latest best practices, updates, or security patches.
Solution:
Consistent Learning: Regularly visit official documentation, attend conferences, or engage in online Python communities.
List of Resources:
Python.org’s official documentation
PyCon conferences
Forums like Stack Overflow, and Reddit’s r/Python
Key Takeaway: Continual learning is essential in the rapidly evolving tech world.
4. Problem: Relying Excessively on External Libraries
Consequences: Can lead to bloated applications, security vulnerabilities, and dependency issues.
Solution:
Due Diligence: Before integrating a library, research its popularity, maintainability, and security aspects.
Table of Criteria:
Criteria | Description |
---|---|
Popularity | Number of downloads, stars on GitHub |
Maintainers | Active and responsive maintainers |
Last Update | Recently updated libraries are more likely to be secure and reliable |
Key Takeaway: Use external resources wisely; every added dependency is also a potential risk.
5. Problem: Not Optimizing Memory Management
Consequences: Leads to inefficient applications, memory leaks, and unexpected crashes.
Solution:
Understand Python’s Garbage Collection: Know when and how to influence garbage collection for memory optimization.
Example: Use Python’s gc
module to manually control garbage collection in memory-critical applications.
Pro Tip: Tools like objgraph
can help identify memory leaks in Python applications.
Code Sample:
import gc
gc.collect() # Manually run garbage collection
Key Takeaway: Proactive memory management can drastically improve application performance.
Having advanced Python skills is undeniably beneficial, but it’s also vital to navigate the challenges that come with it judiciously. By being aware of potential pitfalls, developers can write robust, efficient, and maintainable Python applications.
Wrapping up – What are the Advanced Python Skills Some Developers Have?
Acquiring foundational Python skills is just the beginning. Many developers strive to master advanced competencies to elevate their expertise and tackle more complex projects.
1. Deep Learning and AI Integration:
- Mastery in libraries like TensorFlow, Keras, and PyTorch allows developers to build and integrate sophisticated machine learning models.
2. Advanced Web Frameworks:
- Beyond Flask and Django, proficiency in asynchronous frameworks like FastAPI and Tornado equips developers for high-performance web applications.
3. Multi-threading and Multiprocessing:
- Implementing concurrent programming techniques ensures optimal performance by fully utilizing available system resources.
4. Cython and Python C Extensions:
- Using Cython or creating C extensions enables developers to write high-performance code sections in C, boosting the application’s speed.
5. Design Patterns and OOP Principles:
- Applying advanced Object-Oriented Programming (OOP) and design patterns ensures clean, reusable, and maintainable code structures.
6. Python Memory Management:
- Proficient memory management, including understanding Garbage Collection and reference cycles, optimizes application efficiency.
Key Takeaway: Advanced Python skills are often the result of continuous learning and hands-on experience. These competencies empower developers to craft superior applications and stay at the forefront of technological innovation.
FAQs – What are the Advanced Python Skills Some Developers Have?
1. FAQ: How do advanced developers utilize AI and machine learning in Python?
Answer: Experienced developers often leverage libraries like TensorFlow, Keras, and PyTorch to implement deep learning models and AI integrations.
Example: For image recognition tasks, a developer might use TensorFlow with Convolutional Neural Networks.
Pro Tip: Stay updated with AI research and consistently practice on platforms like Kaggle.
Code Sample:
import tensorflow as tf
model = tf.keras.Sequential([
tf.keras.layers.Conv2D(32, (3,3), activation='relu', input_shape=(150, 150, 3)),
#... (additional layers)
])
2. FAQ: What advanced web frameworks are Python pros using beyond Flask and Django?
Answer: Many seasoned developers are now using asynchronous frameworks such as FastAPI and Tornado for enhanced performance and scalability.
Example: For real-time applications or services with high concurrent requests, FastAPI can be a game-changer.
Pro Tip: Asynchronous frameworks shine with I/O bound operations. Ensure your use case benefits from them.
Code Sample:
from fastapi import FastAPI
app = FastAPI()
@app.get("/")
async def read_root():
return {"Hello": "World"}
3. FAQ: How do experts handle multitasking in Python for performance optimization?
Answer: By mastering multi-threading and multiprocessing, developers can achieve parallelism, maximizing resource usage.
Example: Using Python’s threading
module for concurrent I/O-bound tasks or multiprocessing
for CPU-bound tasks.
Pro Tip: Always be cautious of the Global Interpreter Lock (GIL) when threading in CPython.
Code Sample:
from multiprocessing import Process
def print_func(name):
print(f'Hello {name}')
if __name__ == '__main__':
process = Process(target=print_func, args=('Alice',))
process.start()
4. FAQ: How do Python gurus achieve speed boosts using C integration?
Answer: Through Cython and Python C extensions, developers can write performance-critical sections in C, achieving significant speedups.
Example: A computational-intensive operation can be written in C and called from Python to expedite the process.
Pro Tip: Ensure safety and compatibility when integrating C components. Improper integrations can lead to hard-to-trace issues.
Code Sample: (Using Cython)
def fib(int n):
cdef int a = 0, b = 1, i
for i in range(n):
a, b = b, a + b
return a
5. FAQ: What advanced OOP techniques do expert Python developers employ?
Answer: Skilled developers use intricate design patterns and principles to write modular, reusable, and maintainable code.
Example: Implementing the Singleton pattern to ensure a class has only one instance.
Pro Tip: Familiarize yourself with the SOLID principles to better structure your OOP designs in Python.
Code Sample:
class Singleton:
_instance = None
@staticmethod
def getInstance():
if Singleton._instance == None:
Singleton()
return Singleton._instance
def __init__(self):
if Singleton._instance != None:
raise Exception("Singleton class!")
else:
Singleton._instance = self
Harnessing these advanced Python skills can significantly enhance a developer’s capabilities, opening doors to more complex and innovative projects.
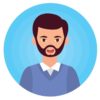
Matthew is a technical author with a passion for software development and a deep expertise in Python. With over 20 years of experience in the field, he has honed his skills as a software development manager at prominent companies such as eBay, Zappier, and GE Capital, where he led complex software projects to successful completion.
Matthew’s deep fascination with Python began two decades ago, and he has been at the forefront of its development ever since. His experience with the language has allowed him to develop a keen understanding of its inner workings, and he has become an expert at leveraging its unique features to build elegant and efficient software solutions.
Matthew’s academic background is rooted in the esteemed halls of Columbia University, where he pursued a Master’s degree in Computer Science.
As a technical author, Matthew is committed to sharing his knowledge with others and helping to advance the field of computer science. His contributions to the scientific computer science community are invaluable, and his expertise in Python development has made him a sought-after speaker and thought leader in the field.