As data becomes an increasingly valuable commodity in today’s digital age, it’s important for businesses to implement efficient and effective solutions for managing their data. Python, a versatile and popular programming language, has emerged as a powerful tool for building database solutions that are both robust and flexible.
Whether you’re looking to build a simple database management system or a complex data analytics platform, Python offers a wide range of tools and frameworks that can help you achieve your goals. With its intuitive syntax, dynamic type system, and extensive library support, Python has become a go-to choice for many data professionals.
What are Python Database Solutions?
Python is a popular programming language used in a variety of domains, including data management. Python database solutions are applications that leverage Python to manage, manipulate, and store data efficiently.
Python database solutions have become increasingly popular in recent years due to the numerous advantages they offer.
One of the benefits of using Python database solutions is their ability to build efficient databases with Python.
Efficient databases are necessary for managing large volumes of data. Python database solutions allow users to interact with databases and perform database operations such as create, read, update, and delete (CRUD) operations. These operations can be performed efficiently using Python, which is known for its simplicity and flexibility.
Popular Python Database Frameworks
In addition to the standard Python library for database management, there are several popular frameworks that can be used to build efficient databases with Python. These frameworks provide higher-level abstractions and features for managing databases, allowing developers to focus on building robust applications instead of low-level details of database management.
SQLAlchemy
SQLAlchemy is a popular Python SQL toolkit and Object-Relational Mapping (ORM) library. It provides a high-level SQL abstraction layer and supports multiple database backends, such as MySQL, PostgreSQL, and SQLite. With SQLAlchemy, developers can create and manage database schemas, execute queries, and manipulate data using Python objects and syntax.
For example, to create a new table in a MySQL database using SQLAlchemy, developers can use the following code:
# Import required modules
from sqlalchemy import create_engine, Column, Integer, String
from sqlalchemy.ext.declarative import declarative_base
# Create an engine for the database
engine = create_engine('mysql+pymysql://user:password@host/db_name')
# Define a new table class
Base = declarative_base()
class User(Base):
__tablename__ = 'users'
id = Column(Integer, primary_key=True)
name = Column(String(50))
email = Column(String(50))
# Create the table
Base.metadata.create_all(engine)
With this code, a new table named “users” with three columns (id, name, and email) will be created in the specified MySQL database. Developers can then use Python objects to add or retrieve data from this table.
Django ORM
Django ORM is the default Object-Relational Mapping (ORM) tool provided by the Django web framework. It provides an intuitive and easy-to-use interface for managing database operations using Python. With Django ORM, developers can define database models and manipulate data using Python objects and syntax, similar to SQLAlchemy.
For example, to define a new model in Django ORM for a database table, developers can use the following code:
# Import required modules
from django.db import models
# Define a new model
class User(models.Model):
name = models.CharField(max_length=50)
email = models.EmailField(max_length=50)
In this code, a new model “User” is defined with two fields – name and email. Developers can then use this model to create or retrieve records from the corresponding database table.
Both SQLAlchemy and Django ORM are powerful frameworks for managing databases with Python. Developers can choose the framework that best suits their needs and preferences based on the features and capabilities provided by each framework.
Code examples with SQLAlchemy
SQLAlchemy is a popular Python framework for working with databases. Its powerful object-relational mapping (ORM) features make it easier to work with databases, especially in complex projects.
Let’s look at some code examples to understand how SQLAlchemy can be used for building efficient databases with Python.
Creating a database connection
First, we need to create a database connection using the create_engine method.
Code:
from sqlalchemy import create_engine engine = create_engine ('postgresql://username:password@localhost:5432/ mydatabase')
In the above example, we are creating a connection to a PostgreSQL database named ‘mydatabase’. We also specify the username and password required to access the database.
Creating a table
Next, we can use the declarative_base method to define a table schema and create a new table in the database.
Code:
from sqlalchemy.ext.declarative import declarative_base from sqlalchemy import Column, Integer, String Base = declarative_base() class User(Base): __tablename__ = 'users' id = Column(Integer, primary_key=True) name = Column(String) age = Column(Integer)
In the above example, we create a new table called ‘users’ with three columns: id, name, and age. The primary_key argument specifies that the id column is the primary key for the table. We can now create this table using the create_all method.
Inserting data into a table
We can use the session object to insert data into a table.
Code:
from sqlalchemy.orm import sessionmaker Session =
sessionmaker(bind=engine) session = Session() new_user = User
(name='John Doe', age=30) session.add(new_user) session.commit()
In the above example, we create a new User instance with name ‘John Doe’ and age 30. We then add this instance to the session object and commit the changes. This will insert the new user data into the ‘users’ table.
Querying data from a table
We can use the session object to query data from a table.
Code:
users = session.query(User).all() for user in users: print
(user.name, user.age)
In the above example, we query all the user data from the ‘users’ table and store it in a Python list. We can then iterate over the list to print the name and age of each user.
SQLAlchemy offers many other features and capabilities for working with databases in Python. By leveraging these features, we can build efficient and robust data solutions using Python.
Code examples with Django ORM
Similar to SQLAlchemy, Django also provides an Object-Relational Mapping (ORM) interface for interacting with databases in Python. Let’s take a look at some code examples to see how we can use Django ORM to build efficient databases with Python.
Creating a model
In Django, a model is an abstraction of a database table. We can use the model class to create a new table or interact with an existing table. Here’s an example of defining a model for a simple blog post:
from django.db import models class Post(models.Model): title = models.CharField(max_length=200) content = models.TextField() pub_date = models.DateTimeField(auto_now_add=True)
Here we define a model called Post, which has three fields: title, content, and pub_date. The title and content fields are CharField and TextField types respectively, and pub_date is a DateTimeField type with auto_now_add=True, meaning it will automatically set the date and time of creation.
Creating a new record
Now that we have defined our model, we can create a new record in the database by creating a new instance of the model and calling the save() method:
post = Post(title='My First Post', content='Hello World!') post.save()
This will create a new record in the Post table with the title “My First Post” and the content “Hello World!”.
Retrieving records
We can retrieve records from the database using the QuerySet API provided by Django. Here’s an example of retrieving all posts from the database:
posts = Post.objects.all()
This will return a QuerySet object containing all posts in the database. We can also filter the QuerySet to retrieve specific records:
post = Post.objects.get(title='My First Post')
This will return the post with the title “My First Post”. We can also use other filter methods like filter(), exclude(), and order_by() to further refine our QuerySet.
Django ORM provides a powerful interface for interacting with databases in Python. Its intuitive syntax and extensive documentation make it a popular choice for building robust data solutions with Python.
Performance considerations in Python database solutions
When building database solutions with Python, it’s essential to consider performance optimization. The following tips and techniques can help ensure the efficiency of your Python database solutions:
- Indexing: Adding indexes to frequently used columns can significantly improve query performance.
- Batch operations: Instead of executing a large number of individual SQL statements, batching them can reduce overhead and enhance performance.
- Memory usage: Be mindful of memory usage when working with large datasets, as excessive memory consumption can impact performance.
Additionally, understanding the inner workings of the Python database frameworks you’re using can help optimize performance. Let’s take a look at some code examples using SQLAlchemy and Django ORM to demonstrate their performance optimization features.
Code examples with SQLAlchemy
SQLAlchemy provides various features for optimizing performance in Python database solutions. For instance, it allows for the use of bulk operations, reducing the number of SQL statements executed and improving overall efficiency. Here’s an example:
import csv
from sqlalchemy import create_engine, Column, Integer, String
from sqlalchemy.orm import sessionmaker
from sqlalchemy.ext.declarative import declarative_baseBase = declarative_base()
class Customer(Base):
__tablename__ = ‘customers’
id = Column(Integer, primary_key=True)
name = Column(String)
age = Column(Integer)engine = create_engine(‘sqlite:///customers.db’)
Session = sessionmaker(bind=engine)
session = Session()with open(‘customers.csv’) as csvfile:
datareader = csv.reader(csvfile)
for row in datareader:
customer = Customer(name=row[0], age=row[1])
session.add(customer)
if datareader.line_num % 1000 == 0:
session.flush()
session.commit()
In the above example, the data from a CSV file is being added to a SQLite database using SQLAlchemy. By utilizing the session.flush()
method, the changes are committed to the database in batches of 1000, rather than individually. This significantly reduces the overhead involved in committing changes for large datasets.
Code examples with Django ORM
Django ORM also provides several performance optimization features that can be used in Python database solutions. For instance, the select_related()
method allows for efficient querying of related objects, reducing the number of SQL queries required. Here’s an example:
from django.db import models
class Author(models.Model):
name = models.CharField(max_length=100)
age = models.IntegerField()class Book(models.Model):
title = models.CharField(max_length=100)
author = models.ForeignKey(Author, on_delete=models.CASCADE)books = Book.objects.select_related(‘author’)
for book in books:
print(book.title, book.author.name)
In the above example, the select_related()
method is used to fetch the related author objects along with the books, rather than fetching them separately. This reduces the number of SQL queries executed and enhances performance.
By utilizing the performance optimization features provided by Python database frameworks such as SQLAlchemy and Django ORM, you can design robust and efficient database solutions with Python.
Real-world examples of Python database solutions
Python database solutions have been successfully implemented by many companies and projects to manage their data efficiently and robustly. Here are some real-world examples of organizations that have leveraged Python for their data management needs:
Reddit, the popular social news aggregator, uses Python as their primary programming language and leverages PostgreSQL as their database management system. They also use the SQLAlchemy framework to interact with their database efficiently. By using Python for their data management needs, Reddit is able to quickly process and analyze massive amounts of user-generated data, providing a personalized experience to its users.
Spotify
Spotify, the music streaming service, also utilizes Python for their data management needs. They use a combination of Python and Apache Cassandra for their database solution, which allows them to store large amounts of data efficiently and process user queries quickly. Additionally, they use the Django ORM to interact with their databases, which provides a high level of abstraction and ease of use.
City of Chicago
The City of Chicago leverages Python for their data analytics and management needs. They use a variety of tools and frameworks such as pandas, NumPy, and SQLAlchemy to process and analyze data related to public safety, transportation, and other municipal services. By using Python, the City of Chicago is able to quickly and efficiently analyze complex data, enabling them to make data-driven decisions that benefit the citizens of the city.
These real-world examples demonstrate the versatility and power of Python database solutions in various industries and applications. By leveraging Python for their data management needs, these organizations are able to improve their efficiency, scalability, and decision-making capabilities.
Advantages of Python for Database Solutions
Python is an excellent language for building robust database solutions due to its flexibility, scalability, and ease of development. Python’s minimalist syntax allows developers to write code more concisely, reducing the time and effort required to develop and maintain database applications.
One of the key advantages of leveraging Python for database solutions is its extensive support for database frameworks, including SQLAlchemy and Django ORM. These frameworks provide a set of tools and utilities for interacting with databases, offering developers a choice of tools and techniques for building efficient databases with Python.
Framework | Advantages |
---|---|
SQLAlchemy | Offers a high degree of customization and flexibility, with support for a wide range of databases and features such as ORM, schema generation, and introspection. |
Django ORM | Provides a high-level abstraction layer that simplifies database queries and management, with a strong focus on rapid development and code reusability. |
Python’s support for object-oriented programming (OOP) makes it an ideal choice for data modeling and abstraction, allowing developers to organize data into classes and objects that can be easily manipulated and queried. Python also offers extensive support for data visualization and analysis, with libraries such as NumPy, Pandas, and Matplotlib providing powerful tools for data processing and analysis.
In addition to these advantages, Python also has a strong and vibrant community of developers and users, who have contributed a wide range of libraries, tools, and frameworks for building and managing databases with Python.
Overall, the advantages of using Python for building database solutions are clear, with its flexibility, scalability, and ease of development making it an excellent choice for developers looking to build efficient and robust data solutions.
Challenges and Considerations in Python Database Solutions
While Python database solutions offer many benefits, they also come with some challenges and considerations that developers need to be aware of. These include:
- Security concerns: As with any database solution, security is a critical concern. Developers need to ensure that their Python database solutions are secure and that sensitive data is protected from unauthorized access.
- Scalability: Python is a popular language for prototyping and building solutions quickly, but it may not be the best option for large-scale applications that require high-performance and scalability. Developers may need to consider alternative solutions for these types of projects.
- Data integrity: Maintaining data integrity is a crucial aspect of database management. Developers need to ensure that their Python database solutions are designed to prevent data loss, corruption, or other issues that can compromise data quality.
However, with careful planning and development, these challenges can be overcome. Here are some tips to help developers ensure a successful Python database solution:
- Plan for security: Incorporate security features like encryption and access controls into your Python database solutions from the outset.
- Consider alternative solutions for scalability: If you anticipate scaling beyond what Python can handle, consider using a different language or technology that can better handle your needs.
- Test, test, test: To ensure data integrity, thoroughly test your Python database solutions to identify and fix any issues before deploying them.
Python database solutions are an excellent choice for many projects due to the language’s flexibility and ease of development. By understanding and addressing the challenges and considerations, developers can create robust and effective Python-based data solutions that meet their business needs.
Conclusion
Python database solutions have proven to be a powerful tool for building efficient and robust databases. As we have seen, Python frameworks such as SQLAlchemy and Django ORM offer a wide range of features and capabilities that enable developers to create data solutions that are flexible, scalable, and easy to maintain.
Expert Python database solutions can help businesses of all sizes leverage Python for their data management needs. Whether it’s building a custom application or managing a large-scale enterprise database, Python provides the flexibility and scalability needed to meet diverse needs and accommodate changing requirements.
Building efficient databases with Python requires a deep understanding of the language and its frameworks. As we have seen, there are a number of best practices and techniques that can be used to optimize performance and ensure data integrity.
While there are challenges and considerations to keep in mind when implementing Python database solutions, such as security and scalability, the advantages of using Python for data management are clear. Python is a powerful and versatile language that enables developers to build efficient, scalable, and robust data solutions quickly and easily.
If you’re looking to leverage Python for your own data solutions, now is the time to start exploring the power of this versatile language and its frameworks. With expert Python database solutions, you can build efficient and robust data solutions that meet the diverse needs of your business and help you stay ahead of the competition.
FAQ
Q: What are Python database solutions?
A: Python database solutions refer to the use of Python programming language to build and manage databases efficiently. Python provides various frameworks and libraries that enable developers to interact with databases and perform operations such as querying, inserting, and updating data.
Q: What are popular Python database frameworks?
A: Some popular Python database frameworks include SQLAlchemy and Django ORM. These frameworks provide a high-level interface for interacting with databases and offer features such as object-relational mapping (ORM) and query generation.
Q: Can you provide code examples for using SQLAlchemy?
A: Certainly! Here’s a code example that demonstrates how to connect to a database using SQLAlchemy:
“`python
from sqlalchemy import create_engine, MetaData
# Create an engine to connect to the database
engine = create_engine(‘postgresql://username:password@localhost/mydatabase’)
# Initialize a metadata object
metadata = MetaData(bind=engine)
# Perform database operations using the metadata object
# …
“`
This is just a basic example, and there are many more features and functionalities provided by SQLAlchemy that you can explore.
Q: How can Django ORM be used for database operations?
A: Django ORM is a powerful tool for working with databases in Python. Here’s an example of how you can use Django ORM to retrieve data from a database:
“`python
from django.db import models
class Customer(models.Model):
name = models.CharField(max_length=100)
email = models.EmailField()
# Retrieve all customers from the database
customers = Customer.objects.all()
# Access customer attributes
for customer in customers:
print(customer.name, customer.email)
“`
Django ORM provides a convenient and intuitive way to perform various database operations in Python.
Q: What are some performance considerations in Python database solutions?
A: When working with Python databases, it’s important to consider performance optimizations. Some tips for improving performance include using appropriate indexing, minimizing database queries, and optimizing data retrieval and processing logic.
Q: Can you provide real-world examples of Python database solutions?
A: Certainly! Many companies and projects have successfully utilized Python database solutions. One example is Instagram, which leverages Python and its database solutions for managing massive amounts of user data and content. Another example is Pinterest, which uses Python’s database capabilities to store and retrieve user-generated content efficiently.
Q: What are the advantages of Python for database solutions?
A: Python offers several advantages for building database solutions. It provides a flexible and easy-to-use syntax that simplifies development. Python also has a rich ecosystem of libraries and frameworks that enhance database management capabilities. Additionally, Python’s scalability allows for efficient handling of large datasets.
Q: What are the challenges in Python database solutions?
A: While Python offers many benefits for database solutions, there are also challenges to consider. Security is an important aspect to address, as databases may contain sensitive information. Scaling Python databases to handle increased workloads can also be challenging. Ensuring data integrity and preventing data corruption are other considerations to keep in mind.
Q: What are the future trends in Python database solutions?
A: The field of Python database solutions is continuously evolving. Some emerging trends include the integration of machine learning and artificial intelligence algorithms into database operations, the use of blockchain technology for secure data management, and advancements in cloud-based database solutions. These developments have the potential to revolutionize how databases are built and managed using Python.
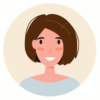
Lydia is a seasoned technical author, well-versed in the intricacies of software development and a dedicated practitioner of Python. With a career spanning 16 years, Lydia has made significant contributions as a programmer and scrum master at renowned companies such as Thompsons, Deloit, and The GAP, where they have been instrumental in delivering successful projects.
A proud alumnus of Duke University, Lydia pursued a degree in Computer Science, solidifying their academic foundation. At Duke, they gained a comprehensive understanding of computer systems, algorithms, and programming languages, which paved the way for their career in the ever-evolving field of software development.
As a technical author, Lydia remains committed to fostering knowledge sharing and promoting the growth of the computer science community. Their dedication to Python development, coupled with their expertise as a programmer and scrum master, positions them as a trusted source of guidance and insight. Through their publications and engagements, Lydia continues to inspire and empower fellow technologists, leaving an indelible mark on the world of scientific computer science.