As businesses and industries continue to rely on technology solutions, designing software solutions with Python has become increasingly important. At [company name], we provide tailored solutions and expertise in leveraging Python for robust architecture.
Python software architecture is crucial for building efficient, scalable, and maintainable software systems. With its flexibility and simplicity, Python has become a popular choice for a wide range of applications.
Understanding Python Software Architecture
Python software architecture is the process of designing and developing software solutions with Python. The architecture of a software solution determines how it functions, how components interact, and how data flows within the system. Python is a versatile and powerful language that offers numerous frameworks for building scalable and maintainable software solutions.
Designing software solutions with Python involves identifying the requirements, creating a blueprint of the solution, and implementing it using Python code. The architecture of a software solution can be modular, meaning that it comprises a set of independent modules that interact with each other. Python’s modularity allows developers to break down the solution into smaller, more manageable parts, making it easier to maintain and update.
Python frameworks provide developers with a set of pre-built tools and libraries that facilitate the development of software solutions. Some popular Python frameworks include Django, Flask, Pyramid, and Tornado. These frameworks provide customizable and scalable solutions for web development, database management, and more.
Code Example: Creating a Simple Web Application in Flask
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello_world():
return 'Hello, World!'
if __name__ == '__main__':
app.run()
The code above demonstrates how to create a basic web application using the Flask framework. The ‘@app.route’ decorator indicates the URL that triggers the ‘hello_world’ function. The function returns a simple greeting message. This code is just an example of how easy it is to implement basic functionality using Python frameworks.
Key Principles of Python Software Architecture
In designing software solutions with Python, it is crucial to adhere to key principles of Python software architecture. Adhering to these principles ensures that the software is modular, scalable, and maintainable, enabling developers to create robust applications. Below are the key principles of Python software architecture:
Modularity
Modularity is a critical principle of Python software architecture. A modular codebase breaks the code into independent, reusable modules that can be tested and deployed separately. This allows developers to focus on specific modules without affecting the rest of the application, making it easier to fix bugs and implement new features. Modularity also promotes code reusability, leading to faster development and easier maintenance.
Below is an example of how to import a module in Python:
# import module
import module_name
Scalability
Scalability is essential when designing software solutions with Python. As the software grows, it should be able to handle an increasing number of users, requests, and data. Applying scalability principles ensures the software can handle the increased demand without compromising performance or reliability. Some key principles for achieving scalability in Python software architecture include using caching, using message queues, and employing load balancing techniques.
Below is an example of how to cache data in Python using the Python caching library, cachetools:
# import caching library
from cachetools import cached, TTLCache
# cache data for 5 seconds
@cached(TTLCache(maxsize=100, ttl=5))
def get_data(key):
# get data from database
return db.get(key)
Maintainability
Maintainability is key when designing software solutions with Python. It involves creating code that is easy to understand, update, and maintain. Code maintainability is critical because, over time, code needs to be updated, debugged, and optimized. Writing maintainable code makes these tasks more manageable, reducing costs and improving the quality of the software.
Some key principles for achieving maintainability in Python software architecture include code readability, commenting, and adhering to best practices.
Below is an example of how to write readable code in Python:
# Example of readable code in Python
def calculate_average(lst):
"""Calculate the average of a list."""
total = sum(lst)
count = len(lst)
return total / count
Comparing Python Frameworks for Software Architecture
Python is an ideal language for software architecture, providing flexibility and ease of use. When it comes to choosing a framework for your project, it can be challenging to decide which one to use. In this section, we compare two popular Python frameworks for software architecture: Flask and Django.
Flask
Flask is a lightweight framework that offers a lot of flexibility for developers. It is suitable for small to medium-sized applications and allows you to start small and add features as needed. One of the key advantages of Flask is its simplicity, as it has a small codebase and minimal dependencies. This makes it easy to learn and use, especially for developers who are new to Python.
Here is an example of creating a simple Flask application:
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello_world():
return 'Hello, World!'
if __name__ == '__main__':
app.run()
This code creates a basic Flask web application that displays a “Hello, World!” message on the root URL.
Django
Django is a more robust framework that is designed for larger applications. It has a more extensive feature set than Flask and provides more out-of-the-box functionality. Django is suitable for complex web applications that require a lot of customization and integration with other systems. One of the strengths of Django is its ability to handle high traffic websites and complex database schemas.
Here is an example of creating a simple Django application:
from django.http import HttpResponse
def hello_world(request):
return HttpResponse("Hello, World!")
This code creates a Django view that displays a “Hello, World!” message.
Comparing Flask and Django
When it comes to choosing between Flask and Django, there are several factors to consider. Flask is more lightweight and flexible, making it an excellent choice for small to medium-sized projects where simplicity is key. Django is more robust and feature-packed, making it ideal for larger projects where scalability and complexity are a concern.
Another important consideration is the development team’s experience with each framework. Flask is more straightforward to learn and use, making it an excellent choice for teams that are new to Python or have limited experience with web development. Django, on the other hand, requires more significant experience and expertise to use effectively.
Building Scalable Applications with Python
Python is an incredibly flexible and powerful language, making it an excellent choice for building scalable applications. Scalability is the ability to handle increased workload or traffic without slowing down or breaking down. In this section, we will explore various architectural patterns and techniques that enable scalability in Python software architecture.
Horizontal and Vertical Scaling
Horizontal scaling involves adding more servers or nodes to a system to handle increased workload or traffic. It involves distributing the workload across multiple servers or nodes and creating a cluster. A cluster is a group of servers that work together to handle requests and provide high availability. This technique is useful for web applications that require high availability and load balancing.
Vertical scaling, on the other hand, involves increasing the resources available on a single server or node to handle increased workload or traffic. It involves adding more CPU, memory, or disk space to a single server. This approach is suitable for applications that require high performance and intensive computation.
Message Queuing
Message queuing is a technique used to decouple components in a system, allowing them to work independently and asynchronously. It involves sending messages between components using a message broker. This technique facilitates scaling by allowing components to handle messages at their own pace, rather than being tied to the speed of other components.
Python provides several message queuing frameworks, including RabbitMQ, ZeroMQ, and Redis.
Cache Optimization
Caching is a technique used to store frequently accessed data in memory, reducing the number of database queries required. It speeds up the application and reduces server load. Python provides several caching frameworks, including Memcached and Redis.
Asynchronous Programming
Asynchronous programming is a technique used to write non-blocking code that can handle multiple requests concurrently. It involves using callbacks, coroutines, or threads to perform tasks in the background while the main thread handles requests. This technique enables high performance and scalability by allowing the application to handle multiple requests simultaneously.
Python provides several frameworks for asynchronous programming, including asyncio, Twisted, and Tornado.
Industry Example
Spotify, the popular music streaming service, uses Python extensively in their backend systems. They use Python for their data processing pipeline, as well as their web services. Python’s flexibility and scalability enable them to handle millions of users and serve millions of requests per day.
By leveraging Python for software architecture, developers can create robust and scalable applications that can handle increased workload or traffic. Using architectural patterns and techniques such as horizontal and vertical scaling, message queuing, cache optimization, and asynchronous programming, developers can build high-performance applications that can handle any workload or traffic.
Ensuring Security in Python Software Architecture
Security is a critical aspect of Python software architecture. With the rise of cyber attacks and data breaches, it is crucial to design software solutions with security in mind. When building a Python application, it is essential to follow best practices for ensuring security.
Encryption is one of the fundamental techniques for securing data in Python applications. By using encryption algorithms, one can protect sensitive data from unauthorized access. For instance, the Advanced Encryption Standard (AES) is a widely used encryption algorithm in Python, which can be easily integrated into applications.
Authentication and authorization are also crucial components of secure Python software architecture. Authentication ensures that only authorized users can access the application, while authorization determines the level of access that users can have. For example, one can use JSON Web Token (JWT) for authentication and authorization in Python applications.
Another technique for ensuring security in Python software architecture is input validation. Input validation is the process of ensuring that user input is within the expected range. By validating input, one can prevent various types of attacks such as SQL injection and cross-site scripting (XSS) attacks. Python provides several libraries for input validation, such as the validators library.
When designing secure Python software architecture, it is also essential to follow the principle of least privilege. The principle of least privilege states that a user should only have the minimum necessary access to perform their job. By following this principle, one can reduce the risk of data breaches and cyber attacks.
Code Example:
Example 1: Using AES encryption in Python
Library | Example Code |
---|---|
pycryptodome | # Define encryption functiondef encrypt(plaintext, key): # Create encryption object cipher = AES.new(key, AES.MODE_ECB) # Pad the plaintextplaintext = plaintext + ((16 – len(plaintext) % 16) * ‘ ‘) # Encrypt the plaintextciphertext = cipher.encrypt(plaintext) # Base64 encode the ciphertextreturn base64.b64encode(ciphertext) # Define decryption functiondef decrypt(ciphertext, key): # Create decryption object cipher = AES.new(key, AES.MODE_ECB) # Base64 decode the ciphertextciphertext = base64.b64decode(ciphertext) # Decrypt the ciphertextplaintext = cipher.decrypt(ciphertext) # Remove padding from the plaintextreturn plaintext.rstrip()
|
The above example demonstrates how to use the pycryptodome library for AES encryption in Python. The code defines two functions: encrypt and decrypt, which take plaintext and key as input and return ciphertext and decrypted plaintext, respectively. The code also demonstrates how to test the encryption and decryption functions using a sample plaintext and key.
Example 2: Using validators library for input validation in Python
Library | Example Code |
---|---|
validators | # Validate email addressif validators.email(‘example@domain.com’): print(‘Valid email address.’) else: print(‘Invalid email address.’) |
The above example demonstrates how to use the validators library for input validation in Python. The code validates an email address using the email function provided by the library. The code prints whether the email address is valid or not.
Designing Robust Error Handling in Python Software Architecture
Errors are inevitable in software development, and it’s crucial to design a robust error handling system to mitigate their impact on your application’s performance and stability. In Python software architecture, there are various error handling techniques and patterns that you can leverage to ensure a reliable and scalable system.
Try/Except Blocks
One of the most common error handling techniques in Python is the try/except block. It allows you to test a block of code for errors and execute alternate code if an error occurs. Here’s an example:
Code Example: |
---|
|
In this example, we attempt to divide 10 by 0, which triggers a ZeroDivisionError. However, we catch the error with the except block and print a custom error message instead of allowing the application to crash.
Logging
Logging is another crucial technique for error handling in Python software architecture. It allows you to record and analyze errors that occur in your application. The Python standard library provides a logging module that you can use to implement logging in your application. Here’s an example:
Code Example: |
---|
try:result = 10 / 0 except ZeroDivisionError as error: logging.exception(error) |
In this example, we configure the logging module to record all log messages to a file named example.log and set the logging level to DEBUG. Then we attempt to divide 10 by 0, which generates a ZeroDivisionError, and we use the logging module to log the error to the file.
Raising Exceptions
Another error handling technique in Python software architecture is raising exceptions. You can raise exceptions explicitly using the raise statement. Here’s an example:
Code Example: |
---|
|
In this example, we define a function that calculates the square root of a number. However, we raise a ValueError if the input number is negative, preventing the function from performing an invalid calculation.
By implementing these and other error handling techniques and patterns in your Python software architecture, you can design a robust and reliable system that can withstand errors and maintain user trust.
Optimal Database Design in Python Software Architecture
Database design is a crucial aspect of software architecture, and Python provides powerful tools for designing efficient and scalable databases. To ensure optimal database design in Python software architecture, it is important to consider key principles such as normalization, indexing, and partitioning.
Normalization is the process of organizing data in a database to eliminate redundancy and dependency. By reducing redundancy, normalization ensures that the database is consistent and easier to maintain. In Python, the sqlalchemy library provides a powerful Object Relational Mapping (ORM) framework for database normalization, enabling developers to work with objects instead of writing raw SQL queries.
Indexing is another important principle in database design, which involves creating indexes on database tables to speed up queries. In Python, the django.db.models library provides built-in support for indexing, allowing developers to define indexes on database models using the db_index attribute.
Partitioning is the process of dividing a large database table into smaller, more manageable partitions. This helps to improve query performance by reducing the amount of data that needs to be scanned. In Python, the psycopg2 library provides support for table partitioning, enabling developers to partition large tables based on specific criteria such as date range or geographical location.
Code Example: Normalizing a Database using SQLAlchemy
from sqlalchemy import Column, Integer, String,
ForeignKey from sqlalchemy.orm import relationship from
sqlalchemy.ext.declarative import declarative_base Base =
declarative_base() class Author(Base): __tablename__ = 'authors' id =
Column(Integer, primary_key=True) name = Column(String) class Book(Base):
__tablename__ = 'books' id = Column(Integer, primary_key=True) title =
Column(String) author_id = Column(Integer, ForeignKey('authors.id'))
author = relationship('Author')
In this example, we define two database tables using the declarative_base function provided by SQLAlchemy. The Author table has a primary key column of id and a name column. The Book table has a primary key column of id, a title column, and a foreign key column of author_id that references the id column in the Author table. The relationship function defines a one-to-many relationship between the Author and Book tables.
By using object-oriented programming concepts and the ORM framework provided by SQLAlchemy, we can achieve a normalized database design that is easier to maintain and more scalable.
Implementing Real-Time Functionality with Python
Real-time functionality is becoming increasingly important in today’s software landscape. Python, with its powerful libraries and frameworks, provides numerous options for implementing real-time features into applications. Real-time functionality can include anything from live chat to real-time analytics. In this section, we will explore some of the technologies and frameworks that enable real-time capabilities in Python software architecture.
WebSockets
WebSockets is a protocol that enables bidirectional communication between a server and a client over a long-held TCP connection. Unlike traditional HTTP requests, WebSockets allow for real-time data to be transmitted between the server and the client without the need for continuous polling. Python has several WebSocket libraries, including WebSockets and Autobahn.
Here is an example of using WebSockets to create a real-time chat application:
import asyncio
import websockets
async def chat(websocket, path):
async for message in websocket:
await asyncio.wait([websocket.send(message) for websocket in websockets])
start_server = websockets.serve(chat, "localhost", 8765)
asyncio.get_event_loop().run_until_complete(start_server)
asyncio.get_event_loop().run_forever()
Server-Sent Events
Server-Sent Events (SSE) is a technology that allows a server to push events to a client in real-time. SSE is a simpler alternative to WebSockets, as it only supports server-to-client communication. Python has several SSE libraries, including sseclient and Flask-SSE.
Here is an example of using the Flask-SSE library to implement real-time updates:
from flask import Flask, render_template
from flask_sse import sse
app = Flask(__name__)
app.config["REDIS_URL"] = "redis://localhost:6379/0"
app.register_blueprint(sse, url_prefix="/stream")
@app.route("/")
def index():
return render_template("index.html")
@app.route("/update")
def update():
import time
from flask_sse import Message
time.sleep(1)
msg = Message("This is a test message", channel="test")
app.extensions["redis"].publish_message("test", msg)
return "Update sent!"
if __name__ == "__main__":
app.run(debug=True)
Python has a variety of options for implementing real-time functionality in software architecture. WebSockets and SSE are just two of the many technologies and frameworks available to developers. By leveraging Python’s powerful libraries and frameworks, developers can create robust and scalable real-time applications.
Testing and Debugging in Python Software Architecture
Testing and debugging are important aspects of Python software architecture. They ensure the quality and reliability of the application. Here are some techniques and methodologies for testing and debugging in Python software architecture:
Unit Testing
Unit testing is a type of testing that focuses on testing individual units of the application. In Python, you can use the built-in unittest module for unit testing, which allows you to write test cases and execute them.
Here is an example:
import unittest
from my_module import add
class TestAddFunction(unittest.TestCase):
def test_addition(self):
result = add(1, 2)
self.assertEqual(result, 3)
if __name__ == '__main__':
unittest.main()
In this example, we import the add function from our module and define a test case for testing its functionality. We use the assertEqual method to compare the result of the function with the expected output.
Integration Testing
Integration testing is a type of testing that focuses on testing the integration of different components of the application. In Python, you can use the pytest framework for integration testing.
Here is an example:
import pytest
from my_module import add
def test_addition():
result = add(1, 2)
assert result == 3
In this example, we define a test case for testing the functionality of the add function using the assert statement. We use the pytest framework to run the integration test.
Debugging Techniques
Debugging is the process of identifying and fixing errors in the application. Here are some debugging techniques that are commonly used in Python software architecture:
- Logging: Logging is a technique that involves using log messages to track the flow of the application and identify errors. In Python, you can use the built-in logging module for logging.
- Debugging Tools: Python provides many debugging tools, such as pdb (Python Debugger) and PyCharm, that allow you to debug your application step-by-step.
- Error Handling: Proper error handling is essential for debugging. You can use try-except statements to catch errors and handle them.
Testing and debugging are critical for ensuring the quality and reliability of Python software architecture. By using these techniques and methodologies, you can identify errors and ensure that your application is functioning correctly.
Conclusion
Python software architecture is a crucial aspect of designing software solutions with Python. Through this article, we’ve explored the various principles of Python software architecture and discussed the importance of leveraging Python for robust architecture.
FAQ
Q: What is Python software architecture?
A: Python software architecture is the process of designing and structuring software solutions using the Python programming language. It involves creating a framework that supports the development and maintenance of robust, scalable, and maintainable applications.
Q: Why is Python software architecture important?
A: Python software architecture is important because it allows developers to design and build applications that are efficient, scalable, and maintainable. It helps in creating a structure that enables easier code maintenance, debugging, and collaboration among team members.
Q: How can Python be leveraged for robust architecture?
A: Python can be leveraged for robust architecture by utilizing its built-in features and libraries. Python offers a rich ecosystem of frameworks and tools that enable developers to design scalable and efficient software solutions. By leveraging Python’s flexibility and extensive libraries, developers can ensure the robustness of their architecture.
Q: What are the key principles of Python software architecture?
A: The key principles of Python software architecture include modularity, scalability, and maintainability. Modularity allows developers to break down the application into smaller, manageable components. Scalability ensures that the application can handle increased load and user demand. Maintainability focuses on creating code that is easy to understand, update, and debug.
Q: Which Python frameworks are commonly used for software architecture?
A: Some commonly used Python frameworks for software architecture include Django, Flask, and Pyramid. Each framework has its strengths and weaknesses, and the choice depends on the specific requirements of the project.
Q: How can real-time functionality be implemented in Python software architecture?
A: Real-time functionality can be implemented in Python software architecture using technologies and frameworks such as WebSocket, asynchronous programming with libraries like asyncio, and message queue systems like RabbitMQ. These tools enable developers to build applications that can process and respond to real-time data.
Q: What are some best practices for ensuring security in Python software architecture?
A: Some best practices for ensuring security in Python software architecture include implementing encryption for sensitive data, implementing user authentication and authorization mechanisms, and regularly updating and patching software to protect against vulnerabilities.
Q: How can robust error handling be designed in Python software architecture?
A: Robust error handling in Python software architecture can be achieved by using try-except blocks to catch and handle exceptions, logging error information for debugging purposes, and implementing appropriate error messages and responses for users.
Q: How can optimal database design be achieved in Python software architecture?
A: To achieve optimal database design in Python software architecture, developers can use techniques such as database normalization, indexing, and query optimization. It is important to design the database schema according to the specific requirements of the application to ensure efficiency and scalability.
Q: What are some testing and debugging methodologies for Python software architecture?
A: Some testing and debugging methodologies for Python software architecture include unit testing, integration testing, and automated testing. Debugging techniques such as logging, breakpoints, and code inspection can be used to identify and fix issues in the code.
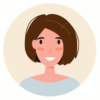
Lydia is a seasoned technical author, well-versed in the intricacies of software development and a dedicated practitioner of Python. With a career spanning 16 years, Lydia has made significant contributions as a programmer and scrum master at renowned companies such as Thompsons, Deloit, and The GAP, where they have been instrumental in delivering successful projects.
A proud alumnus of Duke University, Lydia pursued a degree in Computer Science, solidifying their academic foundation. At Duke, they gained a comprehensive understanding of computer systems, algorithms, and programming languages, which paved the way for their career in the ever-evolving field of software development.
As a technical author, Lydia remains committed to fostering knowledge sharing and promoting the growth of the computer science community. Their dedication to Python development, coupled with their expertise as a programmer and scrum master, positions them as a trusted source of guidance and insight. Through their publications and engagements, Lydia continues to inspire and empower fellow technologists, leaving an indelible mark on the world of scientific computer science.