How to use the Facebook Business API with Python: Start by installing the facebook-sdk
package, then authenticate using your app’s credentials for seamless integration.
The Facebook Business API is a powerful tool that can help businesses achieve marketing success. By leveraging the API with Python, businesses can access valuable insights, create and manage ads, target audiences, and automate campaign optimization.
Key Takeaways
- The Facebook Business API can be used to access valuable insights, create and manage ads, target audiences, and automate campaign optimization.
- Leveraging the API with Python allows businesses to streamline their marketing efforts and drive results.
- This article will provide code examples and step-by-step instructions to help you get started with the Facebook Business API in Python.
What is the Facebook Business API?
The Facebook Business API is a platform that allows businesses to programmatically manage their Facebook marketing campaigns. It provides a wide range of functionalities that enable businesses to create, manage, and optimize their ad campaigns on Facebook, Instagram, and other platforms.
The Facebook Business API is designed for businesses of all sizes, from small startups to large enterprises. It offers a variety of features, including ad creation and management, campaign optimization, audience targeting, and reporting and insights.
The Facebook Business API is a powerful tool for businesses looking to enhance their marketing strategies. By using the API, businesses can automate many of their marketing tasks, saving time and resources while improving campaign performance.
Getting Started with the Facebook Business API in Python
Before utilizing the Facebook Business API with Python, it is necessary to ensure that the required Python libraries are installed. In this section, we will guide you through the steps necessary to get started with the API.
Step 1: Install Required Libraries
The first step is to install the required Python libraries using pip. Open your command prompt and type the following command:
pip install facebook-business
This will install the Facebook Business SDK along with the necessary dependencies.
Step 2: Set Up API Credentials
Next, it is necessary to set up API credentials. Follow these steps:
- Login to your Facebook Business account
- Go to the Business Settings menu and select Generate Access Token
- Choose the appropriate permissions for the token based on your requirements
- Copy the token and store it securely
With the above steps, you are now ready to use the Facebook Business API with Python.
Retrieving Insights using the Facebook Business API in Python
One of the significant benefits of using the Facebook Business API with Python is the ability to access valuable insights about ad performance. By using the API, marketers can retrieve metrics for campaigns, ad sets, and individual ads, which can provide valuable data for optimizing future campaigns.
To retrieve insights using the Facebook Business API in Python, developers can use the ads-insights endpoint. This endpoint provides access to a wide range of metrics, including impressions, clicks, conversions, and cost per action.
Example: Retrieving Ad Metrics
Let’s say you want to retrieve metrics for a specific ad in your campaign. You can use the following Python code to fetch data for the ad:
from facebook_business.adobjects.ad import Ad from facebook_business.adobjects.adaccount import AdAccount from facebook_business.api import FacebookAdsApi from facebook_business.exceptions import FacebookRequestError access_token = 'your_access_token' app_secret = 'your_app_secret' app_id = 'your_app_id' ad_account_id = 'your_ad_account_id' FacebookAdsApi.init(access_token=access_token, app_secret=app_secret, app_id=app_id) fields = [Ad.Field.name, Ad.Field.impressions, Ad.Field.clicks] params = {'time_range': {'since':'2021-01-01','until':'2021-02-01'}} try: ad = Ad('your_ad_id') ad_insights = ad.get_insights(fields=fields, params=params) except FacebookRequestError as e: print('Error: ' + str(e))
In the code above, we’re using the Ad and AdAccount classes from the Facebook Business SDK to retrieve data for a specific ad. We’re also defining the fields we want to fetch, including the name, impressions, and clicks.
The params dictionary is used to specify the time range for the data we want to retrieve. In this example, we’re fetching data for the month of January 2021.
Once we have our parameters defined, we can use the get_insights() method to retrieve data for the ad. If successful, the method will return a list of AdsInsights objects, which contain the requested metrics.
Retrieving insights using the Facebook Business API in Python can provide valuable data for optimizing campaigns and improving marketing success. By using the ads-insights endpoint, developers can access a wide range of metrics and use them to inform future campaign strategy.
Creating Ads with the Facebook Business API in Python
One of the most powerful features of the Facebook Business API is the ability to create and manage ads programmatically. With Python, developers can automate the ad creation process and save time while also optimizing campaign performance.
Creating ads with the Facebook Business API in Python is a straightforward process. Developers can use the API to create various types of ads, including image ads, video ads, and carousel ads, each with its specific format and targeting options.
Creating Image Ads
Image ads are a popular format for Facebook ads, and developers can create them easily using the Facebook Business API in Python. The following code example demonstrates how to create a single image ad:
# import necessary modules and set up API credentials from facebook_business.adobjects.adcreativemediaelement import AdCreativeMediaElement from facebook_business.adobjects.adcreative import AdCreative from facebook_business.adobjects.adaccount import AdAccount ad_account = AdAccount('act_1234567890') ad_creative = AdCreative(parent_id=ad_account['id']) image_element = AdCreativeMediaElement(parent_id=ad_account['id']) image_element[AdCreativeMediaElement.Field.filename] = '/path/to/image.jpg' image_element[AdCreativeMediaElement.Field.media_type] = 'IMAGE' ad_creative[AdCreative.Field.object_story_spec] = {'page_id': '1234567890', 'link_data': {'message': 'Check out our new product!', 'name': 'New Product Ad', 'image_hash': image_element.get_hash()}} ad_creative.remote_create(params={'status': AdCreative.Status.active})
The code above imports necessary modules, sets up the API credentials, and creates an image ad creative with a message and a link to the advertised product. The filename of the image is specified, and the image element is created accordingly. Finally, the ad creative is created and set to active status.
Creating Video Ads
Video ads are an engaging format for Facebook ads, and developers can use the Facebook Business API in Python to create them programmatically. The following code example demonstrates how to create a video ad:
# import necessary modules and set up API credentials from facebook_business.adobjects.adcreativemediaelement import AdCreativeMediaElement from facebook_business.adobjects.adcreative import AdCreative from facebook_business.adobjects.adaccount import AdAccount ad_account = AdAccount('act_1234567890') ad_creative = AdCreative(parent_id=ad_account['id']) video_element = AdCreativeMediaElement(parent_id=ad_account['id']) video_element[AdCreativeMediaElement.Field.filename] = '/path/to/video.mp4' video_element[AdCreativeMediaElement.Field.media_type] = 'VIDEO' ad_creative[AdCreative.Field.object_story_spec] = {'page_id': '1234567890', 'video_data': {'description': 'Check out our new product in action!', 'video_id': video_element.get_hash()}} ad_creative.remote_create(params={'status': AdCreative.Status.active})
The code above imports necessary modules, sets up the API credentials, and creates a video ad creative with a description and a link to the advertised product. The filename of the video is specified, and the video element is created accordingly. Finally, the ad creative is created and set to active status.
Creating Carousel Ads
Carousel ads are a versatile format for Facebook ads, and developers can create them programmatically using the Facebook Business API in Python. The following code example demonstrates how to create a carousel ad:
# import necessary modules and set up API credentials from facebook_business.adobjects.adcreativemediaelement import AdCreativeMediaElement from facebook_business.adobjects.adcreative import AdCreative from facebook_business.adobjects.adaccount import AdAccount ad_account = AdAccount('act_1234567890') ad_creative = AdCreative(parent_id=ad_account['id']) image1_element = AdCreativeMediaElement(parent_id=ad_account['id']) image1_element[AdCreativeMediaElement.Field.filename] = '/path/to/image1.jpg' image1_element[AdCreativeMediaElement.Field.media_type] = 'IMAGE' image2_element = AdCreativeMediaElement(parent_id=ad_account['id']) image2_element[AdCreativeMediaElement.Field.filename] = '/path/to/image2.jpg' image2_element[AdCreativeMediaElement.Field.media_type] = 'IMAGE' ad_creative[AdCreative.Field.object_story_spec] = {'page_id': '1234567890', 'link_data': {'name': 'New Product Ad', 'multi_share_optimized': True, 'child_attachments': [{'link': 'http://example.com/product', 'name': 'Product 1', 'description': 'Description 1', 'image_hash': image1_element.get_hash()}, {'link': 'http://example.com/product', 'name': 'Product 2', 'description': 'Description 2', 'image_hash': image2_element.get_hash()}]}} ad_creative.remote_create(params={'status': AdCreative.Status.active})
The code above imports necessary modules, sets up the API credentials, and creates a carousel ad creative with the name of the advertised product and links to two different products and their descriptions. Two image elements are created, and the filenames and media types are specified. Finally, the ad creative is created and set to active status.
Managing Campaigns with the Facebook Business API in Python
In this section, we will explore how to manage campaigns using the Facebook Business API in Python. The following code examples will demonstrate how to create, update, and delete campaigns, as well as how to retrieve campaign performance data.
Creating a Campaign
To create a new campaign, use the following code:
import facebook def create_campaign(access_token, account_id, campaign_name): graph = facebook.GraphAPI(access_token) fields = {'name': campaign_name, 'status': 'PAUSED', 'objective': 'LINK_CLICKS'} return graph.request('/'+ account_id +'/adcampaigns', args=fields, method='POST')
In this example, access_token
is your Facebook API access token, account_id
is your Facebook Business Manager account ID, and campaign_name
is the name you want to give your new campaign. The fields
dictionary specifies the campaign properties such as name, status, and objective.
Updating a Campaign
To update an existing campaign, use the following code:
def update_campaign(access_token, campaign_id, new_name): graph = facebook.GraphAPI(access_token) fields = {'name': new_name} return graph.request('/'+ campaign_id, args=fields, method='POST')
Here, access_token
is your Facebook API access token, campaign_id
is the ID of the campaign you want to update, and new_name
is the updated name you want to give to your campaign.
Deleting a Campaign
To delete a campaign, use the following code:
def delete_campaign(access_token, campaign_id): graph = facebook.GraphAPI(access_token) return graph.request('/'+ campaign_id, method='DELETE')
In this example, access_token
is your Facebook API access token, and campaign_id
is the ID of the campaign you want to delete.
Retrieving Campaign Performance Data
To retrieve data about your campaign’s performance, use the following code:
def get_campaign_insights(access_token, campaign_id): graph = facebook.GraphAPI(access_token) fields = {'level':'campaign', 'fields': 'reach, impressions, spends', 'time_range': {'since':'2021-01-01', 'until':'2021-01-31'}} return graph.request('/'+ campaign_id +'/insights', args=fields, method='GET')
In this example, access_token
is your Facebook API access token, campaign_id
is the ID of the campaign you want to retrieve insights for, and fields
specifies the data you want to receive. Here, we are requesting data about reach, impressions, and spends during the month of January 2021.
These code examples demonstrate the basic functionality of managing campaigns with the Facebook Business API in Python. By utilizing these methods, you can create, update, delete, and retrieve insights about your campaigns efficiently and effectively.
Targeting Audiences with the Facebook Business API in Python
One of the key benefits of using the Facebook Business API with Python is the ability to target specific audiences for your ads. By defining custom audiences and setting targeting parameters, you can ensure that your ads reach the right people, increasing the chances of conversions and achieving your marketing objectives.
Defining Custom Audiences
The first step in targeting audiences with the Facebook Business API in Python is defining custom audiences. Custom audiences are groups of people that you can create based on a variety of parameters, including website visitors, email subscribers, and app users. You can define custom audiences using the API, and then use them in your ad targeting.
Here’s an example code snippet for creating a custom audience based on website visitors:
// Define the custom audience params = {'name': 'Website Visitors', 'subtype': 'WEBSITE', 'description': 'People who have visited our website'} custom_audience = ad_account.create_custom_audience(fields=[], params=params)
// Add the website visitors rule to the custom audience rule = {'url': {'i_contains': 'example.com'}} custom_audience.update({CustomAudience.Field.rule: rule})
In this example, we create a custom audience called “Website Visitors” and specify that it is based on website traffic. We then add a rule that includes anyone who visited a page on our website that contains the text “example.com”. This creates a custom audience of people who have visited our website and matches our targeting criteria.
Setting Targeting Parameters
Once you have defined your custom audience, you can use it to target your ads to specific groups of people. You can set a variety of targeting parameters, including age, gender, location, interests, and behaviors. Here’s an example code snippet for setting targeting parameters:
// Define the ad set targeting targeting = {Targeting.Field.geo_locations: {'countries': ['US']}, Targeting.Field.age_min: 18, Targeting.Field.age_max: 65, Targeting.Field.genders: [1], Targeting.Field.custom_audiences: [{'id': custom_audience['id']}]}
// Create the ad set with the targeting ad_set = ad_account.create_ad_set(fields=[], params={'name': 'Website Visitors Ad Set', 'campaign_id': campaign['id'], 'daily_budget': 1000, 'billing_event': 'IMPRESSIONS', 'optimization_goal': 'REACH', 'bid_amount': 100, 'targeting': targeting})
In this example, we define the targeting parameters for an ad set. We specify that we want to target people in the United States between the ages of 18 and 65, who identify as female (gender ID 1), and who are included in our custom audience of website visitors. We then create an ad set with these targeting parameters.
Creating Lookalike Audiences
In addition to custom audiences, you can also create lookalike audiences using the Facebook Business API in Python. Lookalike audiences are groups of people who share similar characteristics to your existing customers or website visitors, and can be a powerful tool for expanding your reach and finding new potential customers.
Here’s an example code snippet for creating a lookalike audience:
// Define the source audience for the lookalike audience params = {'name': 'Website Visitors Lookalike', 'source_type': 'CUSTOM_AUDIENCE', 'origin_audience_id': custom_audience['id'], 'lookalike_spec': {'type': 'similarity', 'country': 'US', 'ratio': 0.01}}
// Create the lookalike audience
lookalike_audience = ad_account.create_custom_audience(fields=[], params=params)
In this example, we create a lookalike audience based on our custom audience of website visitors. We specify that we want the lookalike audience to be similar to the source audience, located in the United States, and with a ratio of 0.01 (indicating that we want a lookalike audience that represents 1% of the total population at that location).
By defining custom and lookalike audiences and setting targeting parameters, you can use the Facebook Business API with Python to reach the right people with your ads, and achieve your marketing objectives.
Managing Page Posts with the Facebook Business API in Python
If you’re looking to manage your Facebook page posts efficiently, the Facebook Business API in Python can help. The API allows you to create, update, and delete posts, as well as retrieve engagement metrics. Here are some code examples to get you started.
Create a Page Post
To create a new page post, use the Page.create_feed()
method. Here’s an example:
# Import the necessary libraries from facebook_business.api import FacebookAdsApi from facebook_business.adobjects.page import Page # Set up the API credentials app_id = 'your_app_id' app_secret = 'your_app_secret' access_token = 'your_access_token' FacebookAdsApi.init(app_id, app_secret, access_token) # Create the page post page_id = 'your_page_id' page = Page(page_id) page.create_feed( message='Your message here', link='http://www.example.com', picture='http://www.example.com/image.jpg' )
This code creates a new page post with a message, a link, and an image. You can include additional parameters such as a video or a call-to-action button.
Update a Page Post
To update an existing page post, use the Page.update_feed()
method. Here’s an example:
# Import the necessary libraries from facebook_business.api import FacebookAdsApi from facebook_business.adobjects.page import Page # Set up the API credentials app_id = 'your_app_id' app_secret = 'your_app_secret' access_token = 'your_access_token' FacebookAdsApi.init(app_id, app_secret, access_token) # Update the page post post_id = 'your_post_id' page_post = Page(post_id) page_post.update_feed( message='Your updated message here', link='http://www.example.com/new_link' )
This code updates an existing page post’s message and link. You can include additional parameters such as a new image or a different call-to-action button.
Delete a Page Post
To delete an existing page post, use the Page.delete_feed()
method. Here’s an example:
# Import the necessary libraries from facebook_business.api import FacebookAdsApi from facebook_business.adobjects.page import Page # Set up the API credentials app_id = 'your_app_id' app_secret = 'your_app_secret' access_token = 'your_access_token' FacebookAdsApi.init(app_id, app_secret, access_token) # Delete the page post post_id = 'your_post_id' page_post = Page(post_id) page_post.delete_feed()
This code deletes an existing page post. You can also delete multiple posts at once by passing in an array of post IDs to the Page.delete_feed()
method.
Retrieve Post Engagement Metrics
To retrieve engagement metrics for a specific page post, use the Insights.get()
method. Here’s an example:
# Import the necessary libraries from facebook_business.api import FacebookAdsApi from facebook_business.adobjects.page import Page from facebook_business.adobjects.advideo import AdVideo from facebook_business.adobjects.insightsresult import InsightsResult # Set up the API credentials app_id = 'your_app_id' app_secret = 'your_app_secret' access_token = 'your_access_token' FacebookAdsApi.init(app_id, app_secret, access_token) # Retrieve the post engagement metrics post_id = 'your_post_id' post = AdVideo(post_id) insights = post.get_insights( params={'metric':'post_engaged_users'} ) for insight in insights: print(insight[InsightsResult.Field.post_engaged_users])
This code retrieves engagement metrics for a specific page post and prints the number of people who engaged with the post. You can include additional parameters such as likes, comments, and shares.
With these code examples, you can easily manage your Facebook page posts using the Facebook Business API in Python. Explore more functionalities and features of the API to enhance your marketing campaign.
Automating Campaign Optimization with the Facebook Business API in Python
One of the most significant advantages of using the Facebook Business API with Python is the ability to automate campaign optimization. This means that advertisers can save time and effort by letting the API handle routine tasks and adjustments based on performance data.
There are several strategies for automating campaign optimization with the Facebook Business API in Python. These include:
- Automatically adjusting ad bids based on performance: The API can monitor ad performance and automatically adjust bids to achieve the desired results. For example, if an ad is not getting enough impressions, the API can increase the bid to improve visibility.
- Automatically adjusting ad targeting based on performance: The API can also use performance data to adjust targeting parameters. For example, if an ad is not getting clicks from a specific audience, the API can exclude that audience from future targeting.
- Automatically adjusting campaign budgets based on performance: The API can monitor campaign spending and adjust budgets to optimize performance. For example, if a campaign is spending too quickly without achieving the desired results, the API can lower the budget to conserve spend.
To implement these strategies, developers can use the Python SDK to create rules that automate adjustments based on specific performance metrics. For example, a developer could create a rule that adjusts ad bids when the cost per click exceeds a certain threshold.
It’s important to note that while automating campaign optimization can save time and effort, it’s still essential to monitor and review performance data regularly. Developers should test different optimization strategies and make adjustments based on the results to ensure the best possible performance.
Troubleshooting and Best Practices for Using the Facebook Business API with Python
As with any API, developers may encounter issues when using the Facebook Business API with Python. Here are some common troubleshooting tips:
- Ensure that you have the latest version of the necessary libraries installed
- Check that your API credentials are correct and up-to-date
- Verify that your ad account has the necessary permissions to perform the desired actions
- Review the Facebook for Developers documentation for additional guidance
In addition to troubleshooting, it’s important to follow best practices when utilizing the Facebook Business API with Python:
- Implement error handling and exception catching to avoid crashes or unexpected behavior
- Stay aware of rate limits and adjust your code accordingly to avoid exceeding these limits
- Be mindful of data privacy regulations and ensure that your code is compliant with Facebook’s policies
- Regularly review and update your code to take advantage of new features and improvements
By following these tips and best practices, you can ensure smooth usage of the Facebook Business API with Python and avoid potential pitfalls.
Conclusion – How to use the Facebook Business API with Python
Using the Facebook Business API with Python can significantly contribute to marketing success. With the showcased code examples, businesses can automate and optimize campaigns, retrieve valuable insights, create targeted audiences, and manage page posts efficiently.
It’s important to note that effective utilization of the API requires proper planning and execution. Follow the best practices discussed in this article to avoid potential pitfalls and ensure smooth usage.
By implementing the learnings from this article, businesses can gain a competitive advantage and achieve their marketing goals. So, get started with the Facebook Business API and Python today to unleash the full potential of your marketing strategy.
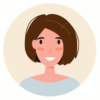
Lydia is a seasoned technical author, well-versed in the intricacies of software development and a dedicated practitioner of Python. With a career spanning 16 years, Lydia has made significant contributions as a programmer and scrum master at renowned companies such as Thompsons, Deloit, and The GAP, where they have been instrumental in delivering successful projects.
A proud alumnus of Duke University, Lydia pursued a degree in Computer Science, solidifying their academic foundation. At Duke, they gained a comprehensive understanding of computer systems, algorithms, and programming languages, which paved the way for their career in the ever-evolving field of software development.
As a technical author, Lydia remains committed to fostering knowledge sharing and promoting the growth of the computer science community. Their dedication to Python development, coupled with their expertise as a programmer and scrum master, positions them as a trusted source of guidance and insight. Through their publications and engagements, Lydia continues to inspire and empower fellow technologists, leaving an indelible mark on the world of scientific computer science.