What are the Basic Python Skills a Junior Developer should have? Mastery over Python’s syntax, data types, and loops ensures solid foundational knowledge, paving the way for advanced development.
In today’s rapidly evolving tech industry, Python has emerged as one of the most popular programming languages. With its simple syntax and versatility, it has become a go-to language for many developers, including junior developers.
If you’re just starting out on your programming journey and want to know what basic Python skills you should have as a junior developer, you’ve come to the right place.
Let’s dive in.
Key Takeaways:
- Basic Python skills are essential for any junior developer looking to jumpstart their career.
- Python syntax and structure, working with data, object-oriented programming, handling exceptions, and code organization are all important areas to focus on.
- Continuously learning and staying updated with Python libraries and frameworks is crucial for enhancing skills and staying relevant in the field.
Understanding Python Syntax and Structure
Python is a high-level programming language that is known for its simplicity and ease of use. Before diving into more complex topics, it’s crucial for junior developers to have a solid understanding of Python’s syntax and structure.
Variables and Data Types
Variables are used in Python to store data values. They can be assigned different types of data, such as integers, strings, and booleans. Understanding data types is crucial for working with data in Python, and it’s important to note that Python is dynamically typed, meaning that the interpreter automatically assigns data types based on the assigned value.
Operators and Control Structures
Operators are used to perform operations on variables and data values in Python, such as arithmetic, assignment, and comparison. Control structures, such as if-statements and loops, are used to control the flow of a program based on certain conditions.
Functions
Functions are the building blocks of Python programs. They are used to encapsulate logic and promote code reuse. Understanding how to define and call functions is a key component of Python programming.
By having a strong comprehension of Python’s syntax and structure, junior developers can begin to write functional code that performs specific tasks.
Working with Data in Python
Python is a powerful programming language that offers a wide range of features for data manipulation and analysis. In this section, we will explore some fundamental concepts of data handling in Python.
Data Manipulation
Python provides a variety of tools and libraries for data manipulation, including NumPy, Pandas, and Scipy. NumPy is a library that provides support for multi-dimensional arrays and matrices, making it easy to work with large datasets. Pandas is a library that offers a set of powerful data manipulation tools, such as data cleaning, filtering, and grouping. Scipy provides a collection of mathematical algorithms, including linear algebra, optimization, and statistics.
Library | Description |
---|---|
NumPy | Library for multi-dimensional arrays and matrices |
Pandas | Library for data manipulation, cleaning, filtering, and grouping |
Scipy | Collection of mathematical algorithms, including linear algebra, optimization, and statistics |
Data Analysis
Python offers several tools and libraries for data analysis, including Matplotlib, Seaborn, and Plotly. Matplotlib is a powerful library for data visualization, offering a wide range of charts and graphs. Seaborn is a library for statistical data visualization, providing an interface to create informative and attractive visualizations. Plotly is a library for creating interactive and dynamic visualizations.
Library | Description |
---|---|
Matplotlib | Library for data visualization, offering a variety of charts and graphs |
Seaborn | Library for statistical data visualization and creating informative and attractive visualizations. |
Plotly | Library for creating interactive and dynamic visualizations |
Python Libraries for Working with Data
The following are some of the most popular Python libraries used for data manipulation and analysis:
- NumPy
- Pandas
- Matplotlib
- Seaborn
- Plotly
Python libraries make it easy to work with data in a variety of ways, from simple analysis to complex mathematical modeling. Learning to use these libraries effectively can make a significant difference in the quality and efficiency of your data analysis.
Object-Oriented Programming in Python
Object-oriented programming (OOP) is a popular programming paradigm used in Python. It allows us to create objects that contain both data and functionality, making it easier to organize and manage code. Understanding OOP is an essential basic Python skill for junior developers.
In Python, classes are used to create objects. A class is a blueprint for an object, and it can contain data, methods, and properties. Objects are instances of a class, and they can be manipulated using methods and properties.
One of the key benefits of OOP is inheritance. Inheritance allows classes to inherit data and functionality from other classes. This reduces code duplication and improves code organization.
Encapsulation is another important concept in OOP. Encapsulation is the practice of hiding data and functionality within a class, protecting it from external access. This helps to ensure data integrity and code reliability.
By mastering OOP concepts like classes, objects, inheritance, and encapsulation, junior developers can write more efficient and organized code. This in turn helps to improve program performance and maintainability.
Handling Exceptions and Errors
In Python programming, errors and exceptions can occur due to various reasons like invalid inputs, logical errors, syntax errors, or system resource constraints. To build reliable and robust Python programs, it is essential to handle these exceptions and errors effectively.
Understanding Exceptions
Exceptions are error conditions that interrupt the normal flow of program execution. When an exception occurs, Python generates an error message and terminates the program if not handled properly. To avoid this, you can include exception handling statements in your code that detect and handle exceptions elegantly.
Error Handling Techniques
Python provides several built-in error handling techniques to catch and handle exceptions. These include try-except blocks, try-except-else blocks, and try-except-finally blocks. You can use these blocks to define the specific actions to be taken when an exception occurs.
Debugging Techniques
Debugging is an essential skill for any Python developer. It involves identifying and correcting errors in your code to ensure smooth program execution. Python provides several debugging techniques, such as using print statements, debugging tools like pdb, and automated testing frameworks.
Clean Code Practices
Python follows a clean and readable syntax, and it is critical to maintain this aspect while handling exceptions and errors. Clean code practices include writing clear and concise code, avoiding nested try-except blocks, and using meaningful variable names. Additionally, following the PEP 8 guidelines helps in writing clean and maintainable code.
Effective exception handling and error management are essential skills for every Python developer. Using the appropriate error handling techniques, debugging tools, and following clean code practices can ensure smooth program execution and maintain code quality.
Working with Files and File Handling
Python provides several ways to work with files, which is an essential aspect of programming. Files enable you to store and retrieve data, settings, and configurations that your program may need to access. The following outlines some basic concepts of file handling.
Reading Files
To read a file in Python, you can use the open() function, which returns a file object that you can use to access the file’s data. The function takes two arguments: the first argument is the file name and the second argument is the mode in which you want to open the file.
The following code snippet shows how to open a file in read mode and print its contents:
# Open the file in read mode file = open('example.txt', 'r') # Read the contents of the file content = file.read() # Print the contents print(content)
After reading the contents of the file, it is important to close the file using the close() method to free up resources.
Writing Files
Similar to reading files, you can use the open() function to write data to a file. The difference is that you need to specify the mode as ‘w’ (write) instead of ‘r’ (read).
The following code snippet shows how to write to a file:
# Open the file in write mode file = open('example.txt', 'w') # Write to the file file.write('Hello, World!') # Close the file file.close()
File Handling Operations
Python provides several built-in functions that you can use to perform operations on files. Some of the common file handling operations are:
Operation | Description |
---|---|
open() | Returns a file object that can be used to read, write, or append to a file |
close() | Closes a file |
read() | Reads the contents of a file |
write() | Writes data to a file |
append() | Appends data to a file |
It is important to handle errors when performing file handling operations. For example, if a file is not found, Python will raise a FileNotFoundError. You can handle this error using the try-except block.
Introduction to Python Libraries and Packages
Python, as an open-source programming language, is known for its extensibility and versatility. This is thanks, in part, to its vast collection of libraries and packages.
These libraries and packages extend Python’s capabilities by introducing new functionality, simplifying complex operations, and automating repetitive tasks.
Libraries and packages can be installed and imported into your Python scripts, giving you access to tools and functions that would otherwise have to be written from scratch.
Python Libraries and Packages
There are thousands of Python libraries and packages available, catering to different needs and improving various aspects of Python programming. Some popular Python libraries and packages include:
Library/Package Name | Description |
---|---|
NumPy | A library for scientific computing with Python. It provides fast and efficient array handling and advanced mathematical functions. |
Pandas | A data manipulation library that provides data structures for efficiently storing and manipulating tabular data, such as CSVs and Excel files. |
Matplotlib | A plotting library that allows you to create static, animated, and interactive visualizations in Python. |
Scikit-learn | A machine learning library that provides tools for data mining, data analysis, and predictive modeling. |
Installing and Importing Libraries
Python libraries and packages can be installed using the pip package installer, which is included with most Python installations. Once installed, you can import the library or package into your Python script using the import statement:
import numpy
You can also give the library or package an alias for easier use:
import pandas as pd
Some libraries and packages may have dependencies, so it is important to ensure that all required libraries are installed before importing them into your script.
Python libraries and packages provide a powerful way to extend Python’s capabilities and simplify coding. By understanding how to install and import libraries, and getting familiar with popular options like NumPy and Pandas, junior developers can take their Python programming to the next level.
Web Development with Python
Python is a powerful language for web development, and there are several popular web frameworks available to choose from. These frameworks provide developers with the tools and functionality needed to build scalable and efficient web applications.
Python Web Frameworks
Flask and Django are two of the most popular Python web frameworks. Flask is a lightweight and modular framework that is ideal for small to medium-sized projects. Django, on the other hand, is a more comprehensive framework that provides a higher level of abstraction and is better suited for larger projects.
Creating a Web Application with Flask
To create a web application with Flask, you will need to install the Flask library and set up a basic project structure. Once you have done this, you can define routes, views, and templates to build your application.
Command/Code | Description |
---|---|
pip install flask | Installs Flask library |
mkdir myproject | Creates a directory for your project |
cd myproject | Navigates to project directory |
touch app.py | Creates a Python file for your application |
Creating a Web Application with Django
Creating a web application with Django involves setting up a project and an app, defining models, views, and templates, and running the development server. Django’s built-in administration interface also makes it easy to manage data using a web-based interface.
Command/Code | Description |
---|---|
pip install django | Installs Django library |
django-admin startproject myproject | Creates a new Django project |
cd myproject | Navigates to project directory |
python manage.py startapp myapp | Creates a new Django app |
Overall, Python is a versatile language that offers many opportunities for web development. By learning one of the popular web frameworks like Flask or Django, developers can build robust and dynamic web applications to meet their clients’ needs.
Testing and Debugging in Python
Testing and debugging are critical aspects of Python programming that ensure code quality and reliability. By identifying and fixing errors, we can prevent code crashes and ensure smooth functionality. Here are some techniques for testing and debugging in Python:
Unit Testing
Unit testing involves testing individual functions and code modules to ensure they work correctly. By breaking down the code into smaller components, we can identify and fix errors more efficiently. Python has various testing frameworks such as unittest, pytest, and nose which facilitate unit testing.
Debugging
Debugging is the process of identifying and fixing errors in a program. Python provides tools such as pdb (Python debugger), which allow developers to trace errors and step through the code line by line. Additionally, print statements are a simple yet effective way to identify errors by logging values at different stages of the code.
Code Reviews
Code reviews involve a team member independently reviewing the code for errors and suggesting optimizations. This process can help identify errors and improve code quality by providing an alternative perspective on the code.
By implementing effective testing and debugging techniques, we can ensure that our Python programs are error-free and reliable.
Best Practices and Code Organization
Writing clean and readable code is crucial for any Python developer, regardless of their level of expertise. By following best practices and maintaining a well-organized codebase, developers can improve the quality, reliability, and maintainability of their code.
Writing Modular and Readable Code
One of the key principles of writing maintainable code is to follow a modular approach. By breaking down complex tasks into smaller, more manageable chunks, developers can improve code reusability and minimize the risk of errors and bugs.
Another important aspect of code readability is to follow a consistent naming convention for variables, functions, and classes. This not only makes the code easier to understand but also helps other developers who might review or contribute to the codebase.
Following PEP 8 Guidelines
PEP 8 is a set of guidelines for coding conventions in Python. It provides recommendations for formatting code, naming conventions, and code organization. By following PEP 8 guidelines, developers can ensure their code is consistent, readable, and maintainable.
Using Version Control
Version control is a critical tool for managing code changes, collaborating with other developers, and tracking project progress. By using a version control system such as Git, developers can easily revert changes, merge code changes from multiple contributors, and maintain a historical record of code changes.
Documenting Code
Proper documentation is essential for understanding how a codebase works and how to use it effectively. By documenting code through comments, docstrings, and readme files, developers can make their code easier to understand and use.
Continuous Learning and Documentation
Learning and keeping up-to-date with Python programming is essential for any junior developer. There are numerous resources available online to help in continuous learning and development, including blogs, newsletters, and forums. Utilizing these resources is crucial to stay informed about new developments in Python, emerging trends, and best practices.
Documentation is a fundamental aspect of software development and should not be overlooked. Documenting code helps to ensure that others can easily understand and use the code, and it also simplifies maintenance and future development.
There are several tools available, such as Sphinx and Docstring, that assist in creating comprehensive and useful documentation.
Additionally, contributing to documentation projects for popular libraries, frameworks, and tools can help to enhance your skills and provide exposure to the wider Python community.
Common Problems and Solutions – What are the Basic Python Skills a Junior Developer should have?
Basic Python Skills a Junior Developer Should Have:
- Understanding of Python Syntax and Structure: Familiarity with indentation, variables, data types, and loops.
- Proficiency in Basic OOP Concepts: Knowledge of classes, objects, inheritance, and encapsulation.
- Comfortable with Common Data Structures: Lists, tuples, dictionaries, and sets.
- Basic knowledge of File I/O: How to read from and write to files.
- Grasp of Error Handling: Using try-except blocks to manage exceptions.
1. Problem: Overlooking Pythonic Conventions
Consequences: Code that is hard for other Python developers to understand, making collaboration difficult.
Solution:
Follow PEP 8: PEP 8 is Python’s official style guide. Adhering to it ensures writing clean and Pythonic code.
Example: Instead of if x == True:
use if x:
.
“Readability counts.”
Key Takeaway: Embrace Python’s conventions for more readable and maintainable code.
2. Problem: Inefficient Data Structure Usage
Consequences: Slower program execution, higher memory consumption, and potential scalability issues.
Solution:
Choose the Right Tool: Understand the strengths and weaknesses of each data structure.
Example: Use sets for membership tests instead of lists for faster lookups.
Pro Tip: Lists are great for ordered collections, but dictionaries excel in key-value pair storage and retrieval.
Key Takeaway: Optimal data structure choice can dramatically enhance performance.
3. Problem: Inadequate Error Handling
Consequences: Unexpected program crashes, difficult-to-debug issues, and poor user experience.
Solution:
Anticipate and Catch Errors: Use try-except blocks proactively to handle potential issues.
Example: When reading a file, account for the possibility that it might not exist.
Code Sample:
try:
with open('file.txt', 'r') as f:
content = f.read()
except FileNotFoundError:
print("The file does not exist.")
Key Takeaway: Robust error handling ensures smoother program execution and improved user experience.
4. Problem: Not Utilizing Built-in Libraries and Functions
Consequences: Reinventing the wheel, longer development time, and potentially less efficient solutions.
Solution:
Familiarize with the Standard Library: Python’s standard library is vast and offers tools for a multitude of common tasks.
Example: Instead of manually coding a file path joiner, use os.path.join()
.
Pro Tip: Explore Python’s official documentation to discover helpful built-in modules and functions.
Key Takeaway: Leveraging built-ins can save time and result in more efficient code.
5. Problem: Hardcoding Values Instead of Using Variables or Constants
Consequences: Difficulties in updating or scaling the code, introducing potential errors during modifications.
Solution:
Use Constants for Fixed Values: If a value is consistent across the program, define it as a constant.
Example: Instead of using the number 3.14
everywhere, define PI = 3.14
and use the variable.
“Code is read much more often than it is written.” – Guido van Rossum (Python’s creator)
Key Takeaway: Dynamic values, even if currently fixed, should be represented as variables or constants for future flexibility.
While learning the basics is essential for junior developers, avoiding common pitfalls is equally vital. By understanding and addressing these challenges, beginners can lay a strong foundation for a prosperous Python development journey.
Conclusion – What are the Basic Python Skills a Junior Developer should have?
In wrapping up, the journey of a junior Python developer is punctuated by mastering foundational skills.
Python Fundamentals:
- Syntax & Structure: The backbone of any Python program, understanding correct indentation and the language’s nuances is crucial.
Core Concepts:
- Data Types: Dive into strings, integers, lists, and dictionaries to manipulate and store information.
- Loops: Harness the power of for and while loops to iterate and automate repetitive tasks effectively.
Decision Making:
- Conditionals: Navigate code flow seamlessly using if, elif, and else statements.
Real-world Applications:
- File I/O: Acquire the know-how to read from and write to files, an essential skill for practical programming.
Stepping into OOP:
- Classes & Objects: An introduction to Object-Oriented Programming is vital, allowing developers to write organized and scalable code.
Key Takeaway: For any junior developer, these foundational skills in Python are the stepping stones to more intricate and advanced programming. Equip yourself with these basics, and the path to expertise becomes a straightforward journey.
Whether it’s the unique nuances of Python’s syntax, the dynamics of loops, or the initiation into Object-Oriented Programming, each step is a building block to a promising coding career.
As newcomers progress, these basic competencies not only serve as their toolkit for solving diverse problems but also lay the groundwork for advanced exploration.
For every aspiring developer, it’s these essentials that bridge the gap between mere interest and real-world application. Dive deep, practice consistently, and let Python’s versatility be your playground.
FAQs – What are the Basic Python Skills a Junior Developer should have?
1. FAQ: What programming fundamentals should a junior Python developer master?
Answer: A junior Python developer should be well-versed in Python’s basic syntax, including variables, data types (like strings, integers, lists, and dictionaries), loops, and conditionals.
Example: Understanding how to loop through a list to manipulate or analyze each item.
for item in my_list:
print(item * 2)
Pro Tip: Familiarize yourself with Python’s interactive shell, IDLE
, to experiment with and test these fundamentals in real-time.
2. FAQ: How important is understanding Object-Oriented Programming (OOP) for a Python beginner?
Answer: While not mandatory initially, grasping OOP concepts such as classes, objects, inheritance, and encapsulation will be essential as the developer progresses.
Example: Creating a simple class for a Dog
object.
class Dog:
def __init__(self, name):
self.name = name
def bark(self):
return "Woof!"
Pro Tip: Start with basic class creation and gradually delve into more complex OOP principles.
3. FAQ: Are there specific tools or libraries a junior Python developer should get acquainted with?
Answer: While the Python Standard Library offers numerous tools, it’s beneficial for juniors to familiarize themselves with commonly used built-in functions and modules like os
, sys
, and datetime
.
Example: Using the datetime
module to get the current date.
from datetime import date
today = date.today()
print("Today's date:", today)
Pro Tip: Use Python’s official documentation to explore and learn about the multitude of tools available in the Standard Library.
4. FAQ: How essential is error handling for a beginner?
Answer: It’s vital. Juniors should understand how to use try-except blocks to handle potential exceptions, ensuring smoother program execution.
Example: Handling division by zero error.
try:
result = 10 / 0
except ZeroDivisionError:
print("You can't divide by zero!")
Pro Tip: Start by handling common errors like TypeError
and ValueError
, then expand your knowledge to more specific exceptions.
5. FAQ: How can a junior developer optimize their code for efficiency?
Answer: Initially, the focus should be on writing clear and functional code. As they progress, they can delve into optimizing by selecting appropriate data structures, minimizing redundancy, and leveraging built-in functions.
Example: Use list comprehensions for more concise code.
squares = [x**2 for x in range(10)]
Pro Tip: Before optimization, ensure the code is correct and readable. The mantra “Make it work, make it right, and then make it fast” holds true.
The journey from being a junior Python developer to an expert involves continuous learning and practice. However, having a solid foundation in these basics ensures a smoother transition to more complex challenges and projects.
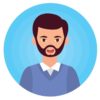
Matthew is a technical author with a passion for software development and a deep expertise in Python. With over 20 years of experience in the field, he has honed his skills as a software development manager at prominent companies such as eBay, Zappier, and GE Capital, where he led complex software projects to successful completion.
Matthew’s deep fascination with Python began two decades ago, and he has been at the forefront of its development ever since. His experience with the language has allowed him to develop a keen understanding of its inner workings, and he has become an expert at leveraging its unique features to build elegant and efficient software solutions.
Matthew’s academic background is rooted in the esteemed halls of Columbia University, where he pursued a Master’s degree in Computer Science.
As a technical author, Matthew is committed to sharing his knowledge with others and helping to advance the field of computer science. His contributions to the scientific computer science community are invaluable, and his expertise in Python development has made him a sought-after speaker and thought leader in the field.