Cross-platform development has become increasingly important in today’s digital landscape. With the rise of mobile and IoT devices, developers face the challenge of creating applications that can run seamlessly across multiple platforms.
Python, a versatile and popular programming language, has emerged as a preferred choice for cross-platform development.
Key Takeaways
- Cross-platform development with Python is essential for creating applications that work seamlessly on multiple platforms.
- Python’s simplicity, readability, and extensive set of libraries make it a preferred choice for cross-platform development.
- Developers can choose from a variety of frameworks and tools available in Python for cross-platform development, such as Kivy, BeeWare, and PyQT.
Why Choose Python for Cross Platform Development
Python has become one of the most popular programming languages for cross-platform development. Its simplicity, readability, and extensive set of libraries make it an ideal choice for developing applications that can run seamlessly on different operating systems and devices. Python’s Simplicity and Readability
One of the most significant advantages of Python is its simplicity and readability. The language’s syntax is straightforward and easy to understand, making it an excellent choice for developers of all skill levels. Python code is also easy to read and maintain, making it an efficient language for collaborative development.
Python’s Extensive Set of Libraries and Frameworks
Python’s extensive set of libraries and frameworks are another reason why it’s a preferred choice for cross-platform development. These libraries and frameworks offer solutions for various tasks, including data processing, web development, artificial intelligence, and machine learning, among others. Some of the popular frameworks for cross-platform development in Python include Kivy, BeeWare, and PyQT.
Support for Multiple Operating Systems and Devices
Python’s ability to run on multiple operating systems and devices is another significant advantage for cross-platform development. Python code can run on various platforms, including Windows, macOS, Linux, and Unix, among others. This means that developers can write code once and deploy it to multiple platforms, saving time and resources.
Ability to Write Once and Run Anywhere
Python’s ability to write once and run anywhere is a crucial factor in cross-platform development. Python code written on one platform can run on any other platform without the need for modification. This feature allows developers to create applications that can run on multiple devices with minimal effort, making it an efficient process.
Understanding Cross Platform Development
Cross-platform development refers to the process of creating software applications that can run on multiple operating systems (OS) and devices. In today’s digital landscape, where customers use a variety of devices such as smartphones, tablets, laptops, and desktop computers, cross-platform development has become a necessary practice for software developers.
However, building a cross-platform application that can operate optimally on many different platforms isn’t a simple task. There are several challenges that developers need to overcome, such as the fragmentation of the device market, diverse operating systems, varying hardware capabilities, and user interface discrepancies. These issues can make it difficult to create a consistent and seamless user experience across all platforms where the application is deployed.
Fortunately, Python has emerged as one of the preferred languages for cross-platform development because of its ease of use, availability of extensive libraries and frameworks, and support for multiple operating systems and devices. Python developers can write code that is platform-agnostic, meaning that it can run on any device or operating system without requiring platform-specific adjustments. This creates a significant advantage for the developer, who can write code once and use it anywhere, reducing development time and costs.
Cross Platform Development Frameworks in Python
Python offers a wide range of cross-platform development frameworks that make it easy to build applications that can run on different operating systems and devices. Here are some popular frameworks that you can use for cross-platform development in Python:
Framework | Description | Use Case |
---|---|---|
Kivy | A cross-platform framework for building touch-enabled applications. It supports iOS, Android, Windows, OS X, and Linux. | Building mobile apps and games |
BeeWare | A collection of tools and libraries for building native user interfaces. It supports iOS, Android, Windows, OS X, and Linux. | Developing user interfaces for different platforms |
PyQt | A set of Python bindings for the Qt application framework. It supports Windows, OS X, and Linux. | Building cross-platform graphical user interfaces |
These frameworks provide a range of features and benefits that make cross-platform development easier and more efficient. With their support for multiple platforms and devices, you can focus on writing code that works across various environments without worrying about the underlying details of each platform.
Cross Platform Development Frameworks in Python with Kivy
Kivy is a popular cross-platform development framework for Python that is designed for building touch-enabled applications. It provides a range of user interface elements and widgets that you can use to create visually appealing applications that can run on multiple devices and platforms.
The following code demonstrates the use of Kivy in building a simple application that displays a button and a label:
Code Example: ``` import kivy from kivy.app import App from kivy.uix.button import Button from kivy.uix.label import Label class MyFirstKivyApp(App): def build(self): button = Button(text="Click Me!") label = Label(text="Hello, world!") return button, label if __name__ == "__main__": MyFirstKivyApp().run() ```
The code creates a button and a label using Kivy’s user interface widgets, and returns them as the application’s root widget. When the button is clicked, the label’s text changes to “Hello, world!”.
This is just a simple example of what you can achieve with Kivy. With its support for touch input, multimedia, and animation, you can create advanced applications that work seamlessly on different platforms.
Next, we’ll explore BeeWare, another popular cross-platform development framework in Python.
Code Example 1 – Using Kivy for Cross Platform Development
Here, we will provide an example code to demonstrate how to use the Kivy framework for building cross-platform applications with Python.
Note: This code is just a sample and may not represent a complete or optimized application.
# Importing the Kivy framework import kivy kivy.require('1.11.1') from kivy.app import App from kivy.uix.label import Label # Creating a custom App class class HelloApp(App): def build(self): return Label(text='Hello, Kivy!') # Running the app on multiple platforms if __name__ == '__main__': HelloApp().run()
In this code, we start by importing the Kivy framework and specifying the required version. We then create a custom HelloApp
class, which inherits from the App
class provided by Kivy. In the build()
method of this class, we simply return a Label
widget that displays the text “Hello, Kivy!”
Finally, we run the application using the run()
method of the HelloApp
object. This code can be used to create a simple “Hello World” application that runs on various platforms, including desktops, mobile devices, and web browsers.
Kivy is a powerful and easy-to-use framework that allows developers to create visually appealing and responsive applications for multiple platforms with Python.
Code Example 2 – Building Cross Platform Apps with BeeWare
BeeWare is another popular cross-platform development framework in Python. It provides a set of tools and libraries that enable developers to write applications that work seamlessly on different platforms, including Windows, Linux, macOS, iOS, and Android.
One of the main advantages of BeeWare is its use of native widgets, which makes it possible to create applications that have a native look and feel on each platform. This ensures that the user experience remains consistent regardless of the device or operating system being used.
Here is an example of how to build a cross-platform application using BeeWare:
# Import the required libraries from beeware import ui from beeware.widgets import Text, Button, Window # Create the application window window = Window(title="My BeeWare App") # Create the text widget text = Text('Hello, World!') # Create the button widget button = Button('Click Me!') # Define the event handler function def on_button_click(): text.set_value('Button Clicked!') # Bind the event handler to the button button.on_click = on_button_click # Add the widgets to the window window.add(text) window.add(button) # Run the application ui.run()
This code creates a simple window with a text widget and a button widget. When the button is clicked, the text widget’s value is changed to “Button Clicked!”.
BeeWare is a powerful framework for cross-platform development in Python. It offers a range of features and tools that enable developers to create visually appealing and responsive applications that work seamlessly on different platforms.
Code Example 3 – Developing Cross Platform Applications with PyQT
PyQt is a popular framework for building cross-platform graphical user interfaces in Python. It provides a set of Python bindings for the Qt application framework and supports multiple platforms, including Windows, macOS, and Linux.
To demonstrate the use of PyQt for cross-platform development, consider the following code example:
'import sys from PyQt5.QtWidgets import QApplication, QWidget app = QApplication(sys.argv) window = QWidget() window.setGeometry(100, 100, 280, 80) window.setWindowTitle('Cross-Platform Development with PyQt') window.show() sys.exit(app.exec_())'
This code creates a simple window with a title and dimensions that can be run on Windows, macOS, or Linux. The first line imports the ‘sys’ module, which provides access to some variables used or maintained by the interpreter and to functions that interact strongly with the interpreter.
The next statement imports QApplication and QWidget classes from the PyQt5.QtWidgets module. The QApplication class manages the GUI application’s control flow and main settings while the QWidget class provides the necessary framework for building the application’s window.
After importing the necessary modules and classes, the code creates a QApplication object by passing ‘sys.argv’ as an argument. This object is required by PyQt to initialize the application and process command-line arguments correctly.
The code then creates a QWidget object called ‘window’, sets its geometry (position and dimensions), adds a title, and shows it on the screen via the ‘show’ method. Finally, the code exits the application’s main loop by calling ‘sys.exit(app.exec_())’.
This code example demonstrates how easy it is to create cross-platform graphical user interfaces with PyQt in Python. With its powerful features, PyQt is an excellent choice for developers looking to build visually appealing and responsive applications that can be deployed on multiple platforms.
Best Practices for Cross Platform Development with Python
When it comes to cross-platform development with Python, there are certain best practices that developers should keep in mind. These practices can help ensure that the application runs smoothly on different platforms and devices, while also maintaining platform independence and consistent user experience. Here are some of the best practices to follow:
- Write platform-agnostic code: To ensure that the application runs seamlessly on different platforms, it is important to write code that is platform-agnostic. This means avoiding platform-specific features and libraries and instead using cross-platform libraries and frameworks.
- Optimize performance for different devices: Different devices have varying hardware capabilities, and it’s important to optimize performance accordingly. This can involve using device-specific APIs and optimizing code for efficient memory usage.
- Handle user interface discrepancies: Different platforms have different user interface guidelines, and it’s important to handle these discrepancies in a way that maintains a consistent user experience across platforms. This can involve using platform-specific UI elements or adapting the UI to match the platform’s guidelines.
- Ensure consistent user experience across platforms: It’s important to ensure that the user experience is consistent across platforms, regardless of the differences in hardware and software. This can involve testing the application on different platforms and making adjustments as necessary.
Following these best practices can help ensure a smooth and consistent experience for users of cross-platform applications developed with Python.
Challenges and Limitations of Cross Platform Development in Python
Cross-platform development with Python comes with its own set of challenges and limitations that developers need to be aware of in order to make informed decisions during the development process. Below are some of the common challenges and limitations that developers may face:
Platform-specific bugs
While Python supports multiple operating systems and devices, there may still be platform-specific bugs that can cause issues during the development process. In order to mitigate this challenge, developers should thoroughly test their applications on different platforms and address any bugs as soon as possible.
Performance trade-offs
Cross-platform development often requires developers to make performance trade-offs in order to ensure their applications can run seamlessly across different devices and operating systems. This can be particularly challenging when working with resource-intensive applications or those that require real-time interaction.
Compatibility concerns
Developers may also face compatibility concerns when developing cross-platform applications. Differences in software versions, hardware configurations, and third-party libraries can all impact the compatibility of an application across different platforms. To mitigate this challenge, developers should stay up-to-date with the latest software and hardware configurations, and test their applications rigorously on different platforms.
Despite these challenges and limitations, cross-platform development with Python remains a popular choice among developers due to its simplicity, versatility, and growing set of frameworks and tools available.
Future of Cross Platform Development with Python
As the digital landscape continues to expand, cross-platform development is becoming increasingly important. With the rise of mobile and IoT devices, there is a growing demand for applications that can run seamlessly on different operating systems and devices.
Python is a versatile programming language that is well-suited for cross-platform development. With its simplicity, readability, and extensive set of libraries and frameworks, Python has become a popular choice for building applications that work on multiple platforms.
Python’s growth is set to continue, and with it, the evolution of cross-platform development frameworks. As the demand for cross-platform applications increases, developers can expect to see more advancements and innovations in the field.
The Python community is continuously working on improving cross-platform development frameworks such as Kivy, BeeWare, and PyQT. These frameworks provide powerful solutions for building cross-platform applications and are continually evolving to meet the changing needs of developers.
In the future, we can expect to see more tools and libraries emerge that will make it even easier for developers to create applications that work seamlessly on different platforms. As Python continues to grow in popularity, so too will the demand for cross-platform development, making it an exciting field to watch.
FAQs – Cross Platform Development with Python
1. What frameworks can I use for cross-platform development with Python?
Answer: There are several robust frameworks, but some of the most popular include:
Kivy: For mobile and multi-touch applications.
PyQt or PySide: For desktop applications across Windows, Mac, and Linux.
BeeWare: Allows you to write apps using Python and then deploy them on multiple platforms.
Pro Tip: Choose a framework based on your target platform and UI needs.
2. How does Python ensure consistent performance across platforms?
Answer: Python is an interpreted language, meaning it runs inside a virtual environment. This ensures consistent behavior regardless of the operating system. However, always test on all target platforms to catch any OS-specific quirks.
Code Sample:
import sys
print(sys.platform) # Check the platform you're currently on
Key Point: While Python ensures consistent logic execution, UI elements might still need adjustments per platform.
3. What challenges should I be aware of when using Python for cross-platform development?
Answer: Some challenges include:
Different OS-specific behaviors or features.
Mobile platforms may need special permissions or have restrictions.
UI consistency across platforms.
Pro Tip: Always check the documentation of your chosen framework for platform-specific considerations.
4. How do I handle platform-specific code in Python?
Answer: Use the sys
module to detect the platform and execute platform-specific code accordingly.
Code Sample:
import sys
if sys.platform == "win32":
# Execute Windows-specific code
elif sys.platform == "darwin":
# Execute macOS-specific code
Key Point: Modularize platform-specific code for better readability and maintainability.
5. Are there any limitations when developing cross-platform mobile apps with Python?
Answer: Yes. While frameworks like Kivy are powerful, they may not have the native feel of apps developed using platform-specific languages. Additionally, performance-intensive apps might not be as efficient as native solutions.
Pro Tip: For CPU-intensive operations or to achieve a truly native feel, sometimes a hybrid approach—combining Python with native code—is best.
Conclusion – Cross Platform Development with Python
Cross-platform development with Python is a powerful approach, especially for rapid prototyping or when targeting multiple platforms simultaneously. However, developers should be aware of platform-specific nuances and always test across all target platforms.
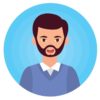
Matthew is a technical author with a passion for software development and a deep expertise in Python. With over 20 years of experience in the field, he has honed his skills as a software development manager at prominent companies such as eBay, Zappier, and GE Capital, where he led complex software projects to successful completion.
Matthew’s deep fascination with Python began two decades ago, and he has been at the forefront of its development ever since. His experience with the language has allowed him to develop a keen understanding of its inner workings, and he has become an expert at leveraging its unique features to build elegant and efficient software solutions.
Matthew’s academic background is rooted in the esteemed halls of Columbia University, where he pursued a Master’s degree in Computer Science.
As a technical author, Matthew is committed to sharing his knowledge with others and helping to advance the field of computer science. His contributions to the scientific computer science community are invaluable, and his expertise in Python development has made him a sought-after speaker and thought leader in the field.