Artificial intelligence (AI) and machine learning (ML) have catapulted to the forefront of modern technological innovations, and Python has emerged as the go-to programming language for developing intelligent solutions. Python’s simplicity, flexibility, and vast ecosystem of libraries and frameworks make it an ideal choice for building sophisticated machine learning models and AI applications.
Understanding Artificial Intelligence and Machine Learning
Artificial Intelligence (AI) and Machine Learning (ML) are two of the fastest-growing fields in the tech industry. The concepts of AI and ML have been around for several decades, but the recent explosion of data and advancements in computing power has led to a renewed interest in these fields. Python has emerged as the programming language of choice for implementing intelligent solutions due to its versatility, simplicity, and extensive libraries.
What is Artificial Intelligence?
Artificial Intelligence (AI) refers to the development of computer systems that can perform tasks that would typically require human intelligence, such as problem-solving, decision-making, perception, and language understanding. AI can be classified into two categories: weak AI and strong AI. Weak AI is designed to perform a specific task, while strong AI is designed to mimic human intelligence and perform any intellectual task.
What is Machine Learning?
Machine Learning (ML) is a subset of AI that involves the development of algorithms that can learn from data and make predictions or decisions. ML can be classified into three categories: supervised learning, unsupervised learning, and reinforcement learning. Supervised learning involves training a model on labeled data to make predictions on new, unseen data. Unsupervised learning involves discovering patterns in unlabeled data without explicit guidance. Reinforcement learning involves training an agent to interact with an environment and learn from feedback.
How Python is used in AI and ML
Python has become the go-to language for AI and ML due to its simple syntax, ease of use, and vast ecosystem of libraries. Python libraries like TensorFlow, PyTorch, and scikit-learn provide a convenient and flexible interface for implementing complex algorithms and models. These libraries abstract away low-level implementation details, allowing developers to focus on high-level concepts and experimentation. Furthermore, Python’s vast community of developers and researchers has resulted in an extensive collection of tutorials, code examples, and research papers, making it easy to get started with AI and ML.
Overall, Python is an excellent language for exploring AI and machine learning. Its simplicity, versatility, and powerful libraries make it an ideal choice for implementing intelligent solutions, even for those new to the field. In the next section, we will delve into popular Python libraries used in AI and ML.
Python Libraries for AI and ML
Python has gained massive popularity in the field of AI and machine learning due to its simplicity, flexibility, and the rich collection of libraries available. In this section, we will explore some of the most popular Python libraries used for AI and machine learning, providing code examples and highlighting their unique features and strengths.
TensorFlow
TensorFlow is an open-source library developed by Google Brain for building and training machine learning models. It is widely used for deep learning applications and offers a range of tools for both beginners and experts. Here’s an example of how to create a simple neural network using TensorFlow:
import tensorflow as tf
model = tf.keras.Sequential([
tf.keras.layers.Dense(64, activation='relu', input_shape=(784,)),
tf.keras.layers.Dense(10)
])
The above code creates a sequential model with two layers – the first layer with 64 neurons and a ReLU activation function, and the second output layer with 10 neurons for classification.
scikit-learn
scikit-learn is a popular Python library that provides various machine learning algorithms for classification, regression, clustering, and dimensionality reduction. It has a simple and intuitive API and works well with other Python libraries like NumPy and Pandas. Here’s an example of how to use scikit-learn for linear regression:
from sklearn.linear_model import LinearRegression
import numpy as np
X = np.array([[1, 2], [3, 4], [5, 6]])
y = np.array([3, 7, 11])
model = LinearRegression()
model.fit(X, y)
predictions = model.predict([[7, 8], [9, 10]])
The above code creates a linear regression model with input features X and output y, fits the model to the data, and makes predictions on new data.
PyTorch
PyTorch is another popular open-source machine learning library that is widely used for deep learning applications. It provides dynamic computational graphs and automatic differentiation, which make it easier to build and train complex models. Here’s an example of how to use PyTorch for image classification:
import torch
import torchvision
model = torchvision.models.resnet18(pretrained=True)
model.fc = torch.nn.Linear(512, 10)
The above code loads a pre-trained ResNet18 model and modifies the output layer to classify 10 classes.
These are just a few examples of the powerful Python libraries available for AI and machine learning. Each library has its own unique features and strengths, making it important to choose the right one for your project needs.
Data Preprocessing and Feature Engineering
When it comes to AI and machine learning projects, data preprocessing and feature engineering are critical steps that can significantly impact the accuracy and reliability of the final model. With Python, these tasks can be handled efficiently and effectively.
Let’s start by discussing data cleaning. This is the process of identifying and correcting or removing inaccurate or incomplete data from a dataset. Python provides several libraries such as Pandas and NumPy that make it easy to clean data. Here’s an example code snippet that demonstrates how to remove rows with missing values:
import pandas as pd
df = pd.read_csv('data.csv')
df.dropna(inplace=True)
Next, let’s talk about data normalization. This step involves scaling the data to a common range to avoid any bias towards specific features. Python provides libraries such as scikit-learn that offer several normalization techniques. Here’s an example code snippet that demonstrates how to normalize data using the MinMaxScaler:
from sklearn.preprocessing import MinMaxScaler
scaler = MinMaxScaler()
normalized_data = scaler.fit_transform(data)
Finally, let’s discuss feature engineering. This step involves transforming raw data into features that better represent the underlying problem. Python provides several libraries such as NumPy and Pandas that can be used for feature engineering tasks. Here’s an example code snippet that demonstrates how to create new features using Pandas:
import pandas as pd
df = pd.read_csv('data.csv')
df['new_feature'] = df['feature_1'] + df['feature_2']
As you can see, Python provides several powerful libraries that make data preprocessing and feature engineering tasks easy and efficient. Leveraging Python for these tasks is a crucial step towards developing intelligent solutions.
Supervised Learning Algorithms and Python
Supervised learning is a machine learning technique where the algorithm is trained on labeled data to make predictions or decisions. Python is a popular language for implementing supervised learning algorithms due to its ease of use and availability of libraries.
Linear Regression
Linear regression is a simple yet powerful algorithm used for predicting continuous values. It works by finding the best-fit line that describes the relationship between the independent and dependent variables. In Python, the scikit-learn library provides a simple interface for implementing linear regression:
from sklearn.linear_model import LinearRegression
reg = LinearRegression().fit(X_train, y_train)
reg.score(X_test, y_test)
Decision Trees
Decision trees are versatile algorithms that can be used for both regression and classification problems. They work by recursively splitting the data into subsets based on the most significant feature. In Python, scikit-learn provides an implementation of decision trees:
from sklearn.tree import DecisionTreeRegressor
reg = DecisionTreeRegressor().fit(X_train, y_train)
reg.score(X_test, y_test)
Support Vector Machines
Support vector machines are powerful algorithms used for classification and regression tasks. They work by finding the best hyperplane that separates the different classes or predicts the target value. In Python, scikit-learn’s implementation of support vector machines is straightforward:
from sklearn.svm import SVR
reg = SVR().fit(X_train, y_train)
reg.score(X_test, y_test)
Python provides various libraries for implementing supervised learning algorithms, making it an ideal language for developing intelligent solutions. With its ease of use and extensive documentation, Python is a first choice for developers and data scientists looking to implement these algorithms in their projects for AI and machine learning with Python, Python for intelligent solutions.
Unsupervised Learning and Python
In the world of machine learning, unsupervised learning techniques stand apart as powerful tools for discovering patterns and hidden structures in data. Python is a popular language of choice for implementing unsupervised machine learning algorithms due to its ease of use and versatility.
Clustering
One of the most common applications of unsupervised learning is clustering, where data points are grouped together based on their similarity. Python offers several libraries for clustering, including Scikit-learn and PyClustering.
For example, let’s say we have a dataset containing information about different types of flowers, including their petal length and width. We can use K-means clustering to group similar flowers together:
import numpy as np
from sklearn.cluster import KMeans
from sklearn.datasets import make_blobs
import matplotlib.pyplot as plt
X, y = make_blobs(n_samples=300, centers=4, cluster_std=0.60, random_state=0)
plt.scatter(X[:,0], X[:,1])
plt.show()
kmeans = KMeans(n_clusters=4)
pred_y = kmeans.fit_predict(X)
plt.scatter(X[:,0], X[:,1])
plt.scatter(kmeans.cluster_centers_[:, 0], kmeans.cluster_centers_[:, 1], s=300, c='red')
plt.show()
This code generates a scatter plot of the flower data and clusters them into four distinct groups using K-means clustering.
Dimensionality Reduction
Another common application of unsupervised learning is dimensionality reduction, which involves reducing the number of features in a dataset while retaining important information. Python offers several libraries for dimensionality reduction, including Scikit-learn and Tensorflow.
For example, let’s say we have a dataset containing information about different types of food, including their nutritional values. We can use principal component analysis (PCA) to reduce the number of features while retaining the most important information:
import numpy as np
from sklearn.decomposition import PCA
from sklearn.datasets import load_wine
import matplotlib.pyplot as plt
wine = load_wine()
X = wine.data
y = wine.target
pca = PCA(n_components=2)
X_pca = pca.fit_transform(X)
plt.scatter(X_pca[:,0], X_pca[:,1], c=y)
plt.show()
This code generates a scatter plot of the food data after using PCA to reduce the number of features to two principal components.
Association Rule Learning
Finally, association rule learning is a technique used to discover interesting relationships between variables in a dataset. Python offers several libraries for association rule learning, including Apriori and FPGrowth.
For example, let’s say we have a dataset containing information about grocery store transactions, including items purchased by customers. We can use association rule learning to discover which items are commonly purchased together:
from mlxtend.preprocessing import TransactionEncoder
from mlxtend.frequent_patterns import apriori
from mlxtend.frequent_patterns import association_rules
import pandas as pd
dataset = [['bread', 'jam', 'butter'], ['bread', 'butter'], ['jam', 'butter'], ['jam', 'butter', 'milk'], ['jam']]
te = TransactionEncoder()
te_ary = te.fit(dataset).transform(dataset)
df = pd.DataFrame(te_ary, columns=te.columns_)
freq_items = apriori(df, min_support=0.6, use_colnames=True)
rules = association_rules(freq_items, metric="confidence", min_threshold=0.7)
print(rules)
This code generates rules that can be used to predict which items are likely to be bought together in future transactions.
The possibilities of unsupervised learning with Python are endless. With the help of its vast libraries and frameworks, it is easy to explore massive datasets and gain insights that may otherwise have gone unnoticed.
Neural Networks and Deep Learning with Python
Neural networks and deep learning are among the most powerful and fascinating applications of artificial intelligence and machine learning. Neural networks are computer algorithms that mimic the structure and function of the human brain, allowing them to learn from data, recognize patterns, and make decisions. Deep learning, on the other hand, is a subset of machine learning that employs neural networks with multiple layers for more complex tasks.
Python is an ideal language for building and training neural networks and implementing deep learning. Its simplicity, flexibility, and rich ecosystem of libraries make it a favorite among data scientists and machine learning practitioners.
Two popular deep learning frameworks in Python are Keras and TensorFlow. Keras is a high-level library that provides an easy-to-use API for building and training neural networks. TensorFlow, on the other hand, is a more low-level library that allows for more fine-grained control over the neural network architecture and optimization algorithms.
Keras | TensorFlow |
---|---|
from keras.models import Sequential from keras.layers import Dense, Dropout model = Sequential() model.add(Dense(64, input_dim=100, activation=’relu’)) model.add(Dropout(0.5)) model.add(Dense(64, activation=’relu’)) model.add(Dropout(0.5)) model.add(Dense(10, activation=’softmax’)) model.compile(loss=’categorical_crossentropy’, optimizer=’adam’, metrics=[‘accuracy’]) model.fit(X_train, y_train, epochs=20, batch_size=128, validation_data=(X_test, y_test)) | import tensorflow as tf from tensorflow import keras from tensorflow.keras import layers model = keras.Sequential() model.add(layers.Dense(64, activation=’relu’, input_dim=100)) model.add(layers.Dropout(0.5)) model.add(layers.Dense(64, activation=’relu’)) model.add(layers.Dropout(0.5)) model.add(layers.Dense(10, activation=’softmax’)) model.compile(loss=’categorical_crossentropy’, optimizer=’adam’, metrics=[‘accuracy’]) model.fit(X_train, y_train, epochs=20, batch_size=128, validation_data=(X_test, y_test)) |
Both Keras and TensorFlow support a wide range of neural network architectures, including convolutional neural networks (CNNs), recurrent neural networks (RNNs), and generative adversarial networks (GANs). They also provide tools for visualizing and monitoring the training process, such as loss and accuracy plots.
Python’s ability to create and train neural networks has been critical in the development of various AI-powered applications, including image and speech recognition, natural language processing, and robotics.
The combination of Python and deep learning has opened up unprecedented opportunities for intelligent solutions in a variety of fields. With Keras and TensorFlow, building and training neural networks has never been easier, making it possible for more people to explore and innovate in the exciting world of AI and machine learning.
Natural Language Processing (NLP) with Python
Natural Language Processing (NLP) is a field of AI that deals with understanding and processing human languages. NLP has several applications, including chatbots, sentiment analysis, and language translation. Python has become the go-to language for NLP, thanks to its vast array of libraries and frameworks designed specifically for this purpose.
Text Classification
Text classification is the process of assigning labels or categories to text based on its content. Python has several libraries, including scikit-learn and NLTK, that make text classification easy and intuitive. For example, the following code snippet shows how scikit-learn can be used for text classification:
from sklearn.feature_extraction.text import CountVectorizer
from sklearn.naive_bayes import MultinomialNB
from sklearn.pipeline import Pipeline
text_clf = Pipeline([
('vect', CountVectorizer()),
('clf', MultinomialNB()),
])
# Train the model
text_clf.fit(X_train, y_train)
# Make a prediction
predicted = text_clf.predict(X_test)
Sentiment Analysis
Sentiment analysis is the process of determining whether a piece of text expresses a positive or negative sentiment. Python has several libraries, including TextBlob and NLTK, that make sentiment analysis simple and straightforward. For example, the following code snippet shows how TextBlob can be used for sentiment analysis:
from textblob import TextBlob
text = "I love this product!"
blob = TextBlob(text)
# Determine sentiment polarity (-1 to 1)
sentiment = blob.sentiment.polarity
Language Generation
Language generation is the process of creating new text based on a given input or prompt. Python has several libraries, including GPT-2 and TextGenRNN, that make language generation easy and intuitive. For example, the following code snippet shows how TextGenRNN can be used for language generation:
from textgenrnn import textgenrnn
textgen = textgenrnn()
textgen.train_from_file('input.txt', num_epochs=5)
# Generate new text
generated_text = textgen.generate(n=1, prefix='hello world', temperature=0.5)
By leveraging Python’s vast array of NLP libraries and frameworks, it is possible to build intelligent solutions that can understand and process human languages with ease.
Reinforcement Learning and Python
Reinforcement learning is a subfield of machine learning that focuses on enabling agents to learn through interacting with an environment. Python has become a popular language for implementing reinforcement learning algorithms because of its efficiency in handling complex operations and its rich ecosystem of libraries and frameworks.
One popular reinforcement learning algorithm is Q-Learning, which involves learning the optimal action-value function for an agent in a given environment. The basic structure of Q-Learning involves updating a table of values that represents the rewards an agent can expect to receive for each possible action in a given state. Python’s NumPy library is commonly used for building this table, as it provides an efficient way to store and manipulate arrays of values.
Python Code Example: Q-Learning with NumPy |
---|
|
Another popular reinforcement learning algorithm is Deep Q Networks (DQNs), which involves using deep neural networks to approximate the action-value function. Python’s TensorFlow and Keras libraries are commonly used for building DQNs, as they provide powerful tools for creating complex neural network architectures.
Python Code Example: Deep Q Networks with TensorFlow | Python Code Example: Deep Q Networks with Keras |
---|---|
|
|
Reinforcement learning is being applied in a wide range of industries, including gaming, robotics, and finance. One notable example is AlphaGo, a computer program that defeated world champion Go player Lee Sedol in a five-game match. AlphaGo was trained using a combination of supervised and reinforcement learning techniques, demonstrating the potential for machine learning to achieve remarkable feats.
FAQ
Q: What is the role of Python in AI and machine learning?
A: Python is widely used in AI and machine learning due to its versatility, ease of use, and extensive library support. It provides a powerful and flexible platform for implementing intelligent solutions.
Q: What are some popular Python libraries for AI and ML?
A: Some popular Python libraries for AI and ML include TensorFlow, scikit-learn, and PyTorch. These libraries offer a wide range of functionality and make it easier to develop and deploy machine learning models.
Q: What are the critical steps of data preprocessing and feature engineering in AI and ML?
A: Data preprocessing and feature engineering are crucial steps in AI and ML projects. They involve tasks such as cleaning and transforming data to make it suitable for analysis and identifying relevant features that will contribute to accurate and reliable model predictions.
Q: What are some supervised learning algorithms that can be implemented in Python?
A: Python supports various supervised learning algorithms such as linear regression, decision trees, and support vector machines. These algorithms can be used to train models that make predictions based on labeled data.
Q: What is unsupervised learning and how can it be implemented in Python?
A: Unsupervised learning is a machine learning technique where algorithms are trained on unlabeled data to discover patterns and underlying structures. In Python, algorithms such as clustering, dimensionality reduction, and association rule learning can be used to perform unsupervised learning tasks.
Q: How can neural networks and deep learning be implemented using Python?
A: Python provides powerful frameworks like Keras and TensorFlow for implementing neural networks and deep learning models. These frameworks offer high-level abstractions and computational efficiency, making it easier to build complex neural networks for various applications.
Q: How can natural language processing (NLP) be implemented using Python?
A: Python offers a range of libraries and tools for implementing NLP tasks such as text classification, sentiment analysis, and language generation. Libraries like NLTK and spaCy provide pre-built models and functions that facilitate NLP development.
Q: What is reinforcement learning and how can it be implemented in Python?
A: Reinforcement learning is a type of machine learning where an agent learns through interactions with an environment to maximize rewards. Python supports reinforcement learning algorithms like Q-Learning and Deep Q Networks, which can be used to develop intelligent systems for gaming and robotics.
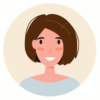
Lydia is a seasoned technical author, well-versed in the intricacies of software development and a dedicated practitioner of Python. With a career spanning 16 years, Lydia has made significant contributions as a programmer and scrum master at renowned companies such as Thompsons, Deloit, and The GAP, where they have been instrumental in delivering successful projects.
A proud alumnus of Duke University, Lydia pursued a degree in Computer Science, solidifying their academic foundation. At Duke, they gained a comprehensive understanding of computer systems, algorithms, and programming languages, which paved the way for their career in the ever-evolving field of software development.
As a technical author, Lydia remains committed to fostering knowledge sharing and promoting the growth of the computer science community. Their dedication to Python development, coupled with their expertise as a programmer and scrum master, positions them as a trusted source of guidance and insight. Through their publications and engagements, Lydia continues to inspire and empower fellow technologists, leaving an indelible mark on the world of scientific computer science.