Best Software Testing techniques for Django: Leverage Django’s built-in TestCase class, ensuring database integrity and isolating tests for consistent, reliable results.
As experienced developers, we understand the value of reliable and efficient testing practices to ensure the functionality, security, and performance of Django applications.
Key Takeaways
- Effective software testing is crucial for high-quality Django web applications.
- Comprehensive testing approaches including unit, integration, functional, performance, and security testing should be prioritized.
- Continuous Integration and Continuous Testing practices can significantly improve the efficiency and reliability of Django development processes.
Importance of Software Testing in Django Development
We understand the crucial role that software testing plays in Django development. In fact, testing is an integral part of the development lifecycle, ensuring the functionality, security, and overall performance of your Django applications. Neglecting proper testing practices can lead to numerous potential consequences, including severe security vulnerabilities, poor performance, and dissatisfied customers.
As Django developers, we must prioritize testing to ensure the success of our projects. Testing not only identifies and addresses issues early on in the development process but also saves time and money in the long run. It ensures that our applications function as expected, meet business requirements, and provide a high-quality user experience.
Unit Testing in Django
Is an essential practice for ensuring the quality and functionality of your Django applications. Unit testing involves testing individual units or components of your code, such as functions or methods, to ensure they behave as intended. In Django, unit testing is typically implemented using the built-in unittest module, which provides a simple and easy-to-use framework for writing test cases.
To get started with unit testing in Django, it’s essential to understand the anatomy of a test case. A test case typically consists of a set of test methods that validate specific behaviors or functionality of your code. Each test method should test a single unit or component of your code and be given a descriptive name that clearly communicates what is being tested.
When it comes to testing Django-specific functionality, such as models or views, it’s essential to use Django-specific testing tools. Django provides a TestCase class that extends the unittest.TestCase class and provides additional functionality specific to Django. For example, the TestCase class provides methods for creating test data fixtures and for testing views within the context of a Django request.
Testing Django Models
When testing Django models, it’s essential to ensure that the model’s behavior and interactions with the database are working correctly. To achieve this, it’s recommended to use the Django TestCase class’s setUpTestData() method to create a set of test data that can be used across multiple test cases.
Once the test data has been set up, it’s recommended to write test methods that validate the individual behaviors and interactions of the model. For example, you might write a test method that validates that a new model instance is created correctly or that a specific field value is set correctly.
Testing Django Views
Testing Django views is essential to ensuring the functionality and behavior of your Django application. When testing views, it’s essential to ensure that they return the correct HTTP response code and that the response content is formatted and structured correctly.
To test Django views, it’s recommended to use the Django TestCase class’s Client object, which provides a simple and easy-to-use interface for making HTTP requests to your application.
When writing test methods for Django views, it’s recommended to use the assertContains() and assertNotContains() methods to ensure that the response content contains the expected content, such as HTML tags or specific text. Additionally, you might write test methods that validate the behavior of your views in response to particular query parameters or POST data.
Integration Testing in Django
Integration testing is an essential aspect of Django development, ensuring the seamless integration of different components within a web application. It involves testing different modules of the application together to verify if they work in sync and produce the expected results.
In Django, integration testing covers a wide range of scenarios, including testing database interactions, APIs, and third-party integrations. Effective integration testing requires careful planning and execution, as it can identify potential issues early in the development cycle and save significant time and effort in the long run.
Testing Database Interactions
Testing database interactions is an essential component of integration testing in Django. A database is an integral part of most web applications and is used to store and retrieve data. In Django, this is typically done using Object-Relational Mapping (ORM).
During integration testing, it is essential to ensure that the ORM code is working correctly and that the data is being stored and retrieved accurately. This involves testing the database schema, relationships, and querying mechanism. It is recommended to use an in-memory SQLite database for testing to speed up the testing process and avoid conflicts with the production database.
Testing APIs
Django provides a robust framework for building APIs, enabling seamless data exchange between different components of the application. Testing APIs is a crucial aspect of integration testing in Django, as it ensures that the API endpoints are working as expected, and the data transfer is occurring without any errors.
During API testing, it is essential to verify the request and response data, authentication and authorization mechanisms, error handling, and performance under different load scenarios. Several tools are available to test APIs, including Django’s built-in test client, third-party libraries like requests, and specialized API testing frameworks like RESTful API Testing Automation (RAITA).
Testing Third-Party Integrations
Django provides an extensive range of third-party libraries, enabling developers to add new functionalities to their applications seamlessly. However, these integrations can sometimes introduce new bugs or issues, making thorough testing vital.
During integration testing, it is essential to verify the compatibility and functionality of third-party libraries with the application. This includes testing different scenarios, error handling, and performance under different load scenarios.
By adopting best practices for integration testing in Django, including testing database interactions, APIs, and third-party integrations, you can ensure the seamless integration of different components within your web application. This ultimately leads to more reliable and secure applications, enhancing customer satisfaction.
Functional Testing in Django
Functional testing is an essential aspect of software testing, and Django is no exception. It aims to verify the functionality and behavior of your Django application from an end-user’s perspective. In this section, we will discuss some techniques to conduct effective functional tests in Django.
Simulating user interactions: One way to perform functional testing in Django is to simulate user interactions with your application. You can use testing frameworks such as Selenium to automate user interactions such as clicking buttons, filling out forms, and navigating your application.
Handling form submissions: Forms are integral components of Django applications, and testing them is crucial to ensure that data is processed accurately. You can use Django’s built-in testing tools to simulate form submissions and verify that the data is processed correctly.
Verifying expected outcomes: Functional tests should verify that the application behaves as expected. You can define specific test cases to check that the correct data is displayed to the user, and the behavior of various application components such as templates and views conform to your expectations.
Several frameworks and libraries facilitate functional testing in Django. For instance, Django’s built-in test framework provides useful tools to automate testing of Django applications. The django.test.Client
class allows you to simulate user interactions with your application, while the unittest.TestCase
class provides powerful methods to test your application’s functionality.
In addition, third-party frameworks such as pytest-django
and Django Live Test
offer additional functionalities and ease of use in conducting functional tests in Django projects.
Performance Testing in Django
Performance testing critically ensures your Django application can handle large volumes of traffic and users while maintaining optimal performance. Common techniques like load testing, stress testing, and benchmarking actively test performance in Django projects.
By load testing, you can identify how many concurrent users your Django application handles before performance issues occur. Stress testing involves actively pushing your application beyond normal conditions to see behavior under extreme loads. Benchmarking helps measure how quickly specific Django functionalities execute under a given load.
Tools like Apache JMeter, Locust, and Artillery can actively simulate traffic and load for performance testing Django. By using these tools, you can proactively identify bottlenecks and potential performance issues to address them in a timely manner. Performance testing is key to building Django applications that can handle real-world demands.
Load Testing in Django using Apache JMeter
Apache JMeter is a popular open-source tool for load testing in Django. It can simulate heavy loads and stress scenarios by generating a large number of requests and measuring the response times. Below are the steps to perform load testing in Django using Apache JMeter:
- Download and install Apache JMeter on your system.
- Create a new test plan and add a thread group to the test plan.
- Configure the thread group with the number of threads and ramp-up time.
- Add a sampler to the thread group to specify which URL(s) to test.
- Configure the sampler with the necessary parameters and test data.
- Run the test and analyze the results to identify performance bottlenecks and issues.
Load testing is essential for identifying how your Django application behaves under heavy traffic. It helps in optimizing your application’s performance and ensures that it can handle a large number of users and requests.
Security Testing in Django
Security is a top priority in web application development. Neglecting security testing can lead to severe consequences, such as data breaches and loss of user trust. As such, it is crucial to implement robust security testing practices in your Django projects.
Common Security Vulnerabilities
In Django development, common security vulnerabilities include cross-site scripting (XSS), SQL injection, and cross-site request forgery (CSRF). These vulnerabilities can compromise the confidentiality, integrity, and availability of your application.
Tools for Security Testing in Django
To identify and mitigate these risks, various security testing tools are available for Django projects. For example, the Django Debug Toolbar can help detect common security issues. Additionally, static code analysis tools, such as Bandit and SonarQube, can identify vulnerabilities in the codebase.
Best Practices for Security Testing in Django
Alongside utilizing security testing tools, following security best practices is essential. For example, implementing user authentication and authorization mechanisms, validating user inputs, and securely storing sensitive data can significantly reduce the likelihood of security breaches. Additionally, keeping your Django application up-to-date with security patches and updates is crucial.
Overall, security testing should not be overlooked in Django development. By implementing security testing tools and best practices, you can improve the security of your application and protect user data.
Continuous Integration and Continuous Testing in Django
Continuous integration (CI) and continuous testing (CT) can streamline the software development process by automating the testing process and reducing the time spent on manual testing. By integrating tests into the development workflow, you can catch potential issues early on and ensure that your Django application remains stable and reliable throughout the development process.
CI/CT Workflow
The CI/CT workflow involves several key steps:
- Developer pushes code changes to a central repository.
- CI server checks out the updated codebase.
- CI server compiles the code and runs automated tests.
- If tests pass, the code is deployed to a staging environment.
- CT tools continue to run tests in the staging environment.
- If all tests pass in the staging environment, the code is deployed to production.
Implementing CI/CT practices can be challenging, but the benefits to development speed, quality, and reliability are significant.
CI/CT Tools for Django
Django provides a suite of testing tools that can be easily incorporated into a CI/CT pipeline, including the built-in test runner and fixtures framework. Additionally, there are many third-party tools and frameworks available that can further enhance CI/CT practices in Django:
Tool/ Framework | Description |
---|---|
Django Jenkins | Integrates Jenkins with Django’s test suite to provide detailed reports and analysis. |
Travis CI | A popular cloud-based CI service that can integrate with Django’s test suite. |
CircleCI | Another cloud-based CI service that can integrate with Django’s test suite. |
PyTest | A popular testing framework that can be used with Django projects. |
By integrating CI/CT practices and tools into your Django development workflow, you can enhance the quality, reliability, and speed of your software development process.
Conclusion
Best Software Testing techniques for Django: Embrace Test-Driven Development (TDD), writing tests before code, ensuring functionality aligns with requirements and reduces regression risks.
By exploring the importance of software testing in Django development, we have discussed various techniques like unit testing, integration testing, functional testing, performance testing, and security testing. Adopting continuous testing practices allows you to prioritize testing and ensure high quality and reliability for your Django web applications.
Remember that testing is an essential, continuous process throughout the development lifecycle, not a one-time task. Neglecting proper testing can lead to severe consequences like security breaches, functionality issues, and performance bottlenecks.
By implementing these software testing techniques and best practices, you can minimize risks and improve customer satisfaction. The ultimate goal is to deliver the best possible user experience while maintaining the integrity and security of your Django projects. Proper testing practices are key to achieving this.
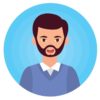
Matthew is a technical author with a passion for software development and a deep expertise in Python. With over 20 years of experience in the field, he has honed his skills as a software development manager at prominent companies such as eBay, Zappier, and GE Capital, where he led complex software projects to successful completion.
Matthew’s deep fascination with Python began two decades ago, and he has been at the forefront of its development ever since. His experience with the language has allowed him to develop a keen understanding of its inner workings, and he has become an expert at leveraging its unique features to build elegant and efficient software solutions.
Matthew’s academic background is rooted in the esteemed halls of Columbia University, where he pursued a Master’s degree in Computer Science.
As a technical author, Matthew is committed to sharing his knowledge with others and helping to advance the field of computer science. His contributions to the scientific computer science community are invaluable, and his expertise in Python development has made him a sought-after speaker and thought leader in the field.