Python, with its simple and easy-to-learn syntax, has gained popularity as a versatile programming language that can be used for various business applications. Its wide range of libraries and frameworks enable businesses to streamline processes, improve efficiency, and achieve automation in their operations.
Key Takeaways
- Python is a versatile programming language with many business applications.
- Python’s libraries and frameworks enable businesses to streamline processes, improve efficiency, and achieve automation.
- Python is widely used in data analysis, web development, machine learning, artificial intelligence, automation of repetitive tasks, financial analysis, natural language processing, IoT, and database management.
Data Analysis and Visualization
Python’s extensive libraries, including Pandas and Matplotlib, make it an ideal language for data analysis and visualization tasks. Businesses can leverage Python’s data analysis capabilities to gain insights into their operations, customers, and market trends.
The following code is an example of how Python can be used for data analysis:
# Import Pandas library import pandas as pd # Read the data from a CSV file data = pd.read_csv("sales_data.csv") # Filter the data by year and month filtered_data = data[(data["Year"] == 2021) & (data["Month"] == "January")] # Calculate the total sales for the month total_sales = filtered_data["Sales"].sum() # Print the total sales print("Total sales for January 2021: ${}".format(total_sales))
Python’s visualization libraries, such as Matplotlib, enable businesses to create compelling visualizations of their data. The following code demonstrates how Python can be used for data visualization:
# Import Matplotlib library for plotting import matplotlib.pyplot as plt # Read the data from a CSV file data = pd.read_csv("sales_data.csv") # Filter the data by product category category_data = data.groupby("Product Category").sum()["Sales"] # Create a pie chart of the sales by product category plt.pie(category_data, labels=category_data.index, autopct='%1.1f%%') # Add a title to the chart plt.title("Sales by Product Category") # Display the chart plt.show()
Web Development
Python is a popular choice for web development due to its powerful frameworks like Django and Flask. These frameworks enable developers to build scalable and robust web applications in a short time. With Python, developers can reduce development time and focus on building user-centric features.
Django
Django is a high-level web framework for Python that draws inspiration from the Model-View-Controller (MVC) architectural pattern. It provides a lot of out-of-the-box functionality, such as authentication, database schema migration, and form handling. The framework follows the Don’t Repeat Yourself (DRY) principle and promotes rapid development. Django is used by companies like Instagram, Mozilla, and Spotify.
Flask
Flask is a micro web framework that is simple and easy to set up. It is lightweight, making it ideal for small projects and rapid prototyping. Flask is flexible and allows developers to choose the components they want to use. It is well suited for building RESTful APIs and web applications. Flask is used by companies like Airbnb, Netflix, and Lyft.
Machine Learning and Artificial Intelligence
Python’s versatility extends to the field of machine learning and artificial intelligence, where its extensive ecosystem of libraries allows businesses to develop intelligent systems. Python libraries like TensorFlow, scikit-learn, and Keras enable developers to build and train machine learning models efficiently and effectively.
One example of a machine learning model built using Python is a convolutional neural network for image classification. This model can classify images into different categories, such as identifying a picture of a dog as a “dog” or a picture of a cat as a “cat.” Here is a sample code for building a convolutional neural network using Keras:
# import necessary libraries import keras from keras.models import Sequential from keras.layers import Dense, Dropout, Flatten from keras.layers.convolutional import Conv2D, MaxPooling2D # create the model model = Sequential() model.add(Conv2D(32, kernel_size=(3, 3), activation='relu', input_shape=(28, 28, 1))) model.add(MaxPooling2D(pool_size=(2, 2))) model.add(Dropout(0.25)) model.add(Flatten()) model.add(Dense(128, activation='relu')) model.add(Dropout(0.5)) model.add(Dense(10, activation='softmax')) # compile the model model.compile(loss='categorical_crossentropy', optimizer='adam', metrics=['accuracy']) # train the model model.fit(X_train, y_train, validation_data=(X_test, y_test), epochs=10, batch_size=200)
With Python’s machine learning capabilities, businesses can develop intelligent systems to automate processes, improve decision-making, and enhance overall efficiency.
Automation of Repetitive Tasks
Python’s simplicity and versatility make it an excellent choice for automating repetitive processes, freeing up time for more high-value tasks. By writing scripts in Python, businesses can automate a wide range of tasks, from file backups to data processing and beyond.
To give an example of how Python can be used for automating tasks, consider the following code snippet:
# Import the OS module import os # Define source and destination directories source = '/path/to/source/directory' destination = '/path/to/destination/directory' # Loop through all files in the source directory for filename in os.listdir(source): # Define the file paths source_file = os.path.join(source, filename) destination_file = os.path.join(destination, filename) # Copy the file to the destination directory shutil.copy(source_file, destination_file)
This simple script uses the os and shutil modules to copy all files from a source directory to a destination directory. By scheduling this script to run periodically, businesses can automate their file backup process and ensure important data is always safe.
Financial Analysis and Trading
Python has become the language of choice for financial analysis and building trading algorithms. Its simplicity, paired with the rich and robust libraries like NumPy and pandas, make it an ideal tool for market analysis and prediction.
One common task in financial analysis is calculating the moving average of a stock price. Python makes it straightforward to quickly compute the moving average with just a few lines of code. Here’s an example:
# Import the necessary libraries import pandas as pd import yfinance as yf # Download data from Yahoo Finance msft = yf.Ticker("MSFT") data = msft.history(period="max") # Define the window size window_size = 20 # Calculate the moving average data['MA'] = data['Close'].rolling(window_size).mean() # Visualize the results import matplotlib.pyplot as plt plt.plot(data['Close']) plt.plot(data['MA']) plt.legend(['Close', 'MA']) plt.show()
This code downloads Microsoft’s stock price history from Yahoo Finance, calculates the moving average with a window size of 20, and plots both the closing price and the moving average on a graph. The resulting visualization can help traders spot trends and make informed decisions based on the data.
Python’s versatility and powerful libraries make it an excellent choice for financial analysis and trading algorithms. By leveraging Python’s capabilities, traders and analysts can gain insights into the market and potentially improve their returns.
Natural Language Processing
Natural Language Processing (NLP) involves analyzing and understanding human language. Python, with libraries like NLTK and spaCy, has become a popular language for NLP tasks such as sentiment analysis and text classification.
An example of sentiment analysis using Python is as follows:
import nltk from nltk.sentiment import SentimentIntensityAnalyzer nltk.download('vader_lexicon') def sentiment_scores(sentence): sid_obj = SentimentIntensityAnalyzer() score_dict = sid_obj.polarity_scores(sentence) print("Overall sentiment dictionary is : ", score_dict) print("Sentence Overall Rated As ", end = " ") if score_dict['compound'] >= 0.05: print("Positive") elif score_dict['compound'] <= - 0.05: print("Negative") else: print("Neutral")
This code uses Python’s NLTK library to perform sentiment analysis on a given sentence, outputting a positive, negative, or neutral rating based on the sentiment dictionary.
Data Analysis and Visualization
Python’s extensive libraries, such as Pandas and Matplotlib, make it ideal for data analysis and visualization tasks. These libraries offer various tools for data manipulation and transformation, statistical and mathematical operations, and plotting and visualization of data in different formats.
For instance, using the Pandas library, businesses can easily load, analyze, and manipulate large datasets with ease. Data can be grouped, filtered, and sorted using just a few lines of code. Similarly, the Matplotlib library can create a wide range of static and interactive visualizations, including line charts, scatterplots, bar charts, and heatmaps.
Example Code for Data Analysis using Python:
# Load the 'sales_data.csv' file into a Pandas DataFrame import pandas as pd df = pd.read_csv('sales_data.csv') # Compute the mean and standard deviation of the unit price column mean_price = df['unit_price'].mean() std_price = df['unit_price'].std() # Create a bar chart of the total revenue by product category import matplotlib.pyplot as plt grouped_df = df.groupby('product_category')['revenue'].sum() grouped_df.plot(kind='bar') plt.title('Total Revenue by Product Category') plt.xlabel('Product Category') plt.ylabel('Revenue') plt.show()
This example code loads a CSV file containing sales data into a Pandas DataFrame, computes the mean and standard deviation of the unit price column, and creates a bar chart of the total revenue by product category using Matplotlib.
Overall, Python’s data analysis and visualization capabilities can provide valuable insights and enable businesses to make data-driven decisions.
Database Management
Python offers a wide range of libraries for interacting with databases and performing various database management tasks. One such library is SQLAlchemy, a popular SQL toolkit and Object-Relational Mapping (ORM) library. SQLAlchemy allows developers to work with databases using Python objects, providing a high level of abstraction and reducing the complexity of interacting with databases.
Another popular library for database management tasks in Python is psycopg2, a PostgreSQL database adapter. psycopg2 provides a simple and efficient way to interact with PostgreSQL databases using Python.
import psycopg2 # Connect to the PostgreSQL database conn = psycopg2.connect
( host="localhost", database="mydatabase", user="myusername", password="mypassword" )
# Create a cursor object cur = conn.cursor() # Execute a SELECT statement cur.execute
("SELECT * FROM mytable") # Fetch all rows from the result set rows = cur.fetchall()
# Print the rows for row in rows: print(row) # Close the cursor and
connection cur.close() conn.close()
Using Python libraries for database management tasks can greatly enhance the efficiency of data-related processes for businesses.
Business Applications of Python: Common Problems and Solutions
Python, with its clear syntax and wide range of libraries, is increasingly popular for business applications. However, like any tool, it isn’t without its challenges. Here are five common problems in business applications using Python, the consequences of these problems, and expert solutions to address them.
1. Problem: Performance Limitations
Consequences:
Reduced application responsiveness.
Longer computation times for data-heavy applications.
Slower runtime performance compared to languages like C++ or Java.
Solution: Cython and JIT Compilation
- Cython: It allows Python to be converted into C code, which can then be compiled. This improves execution speed, especially in computationally intensive parts of the application.
- Just-In-Time (JIT) Compilation (e.g., PyPy): PyPy is an alternative Python interpreter that uses JIT to speed up the execution of Python code.
Expert Quote: “Cython is gold. It allows us to reach near-C speeds with Python-like simplicity.” — A Python Developer.
2. Problem: Global Interpreter Lock (GIL)
Consequences:
Inefficiencies in multi-threading processes.
Inability to fully utilize multi-core processors.
Solution: Multi-processing and Alternative Python Implementations
- Multi-processing: Use Python’s multiprocessing module to run separate processes and sidestep GIL.
- Jython or IronPython: These do not have GIL and allow for true multi-threading.
Key Takeaway: For CPU-bound tasks, consider multiprocessing or an alternative Python implementation without GIL.
3. Problem: Packaging and Dependency Issues
Consequences:
Version conflicts can lead to runtime errors.
Difficulty in managing and deploying applications across different environments.
Solution: Virtual Environments and Dependency Management Tools
- Virtualenv: Allows developers to create isolated Python environments.
- Pipenv or Poetry: These are modern tools that combine package management and environment management.
List of Common Tools:
- Virtualenv
- Pipenv
- Poetry
4. Problem: Memory Consumption
Consequences:
Higher memory usage, especially compared to languages like C.
Possible increased operational costs.
Solution: Memory Profiling and Optimization
- Memory Profilers (e.g., mprof): These tools can identify memory leaks or high consumption areas.
- Optimizing Code: Use efficient data structures and algorithms.
Table: Tools vs. Use-cases
Tools | Use-case |
---|---|
mprof | Memory usage over time |
objgraph | Object reference graphs |
5. Problem: Limited Mobile Development Support
Consequences:
Inability to create native mobile applications directly.
Dependency on third-party tools for mobile app development.
Solution: Use Python Frameworks for Mobile
- Kivy: A popular Python library for developing multitouch applications.
- BeeWare: A set of tools and libraries for building native mobile apps with Python.
Key Takeaway: While Python isn’t the first choice for mobile development, frameworks like Kivy and BeeWare offer possibilities.
While Python is a powerful and versatile language, it’s essential to be aware of its limitations, especially in a business context. However, with the right tools and approaches, many of these challenges can be effectively addressed.
Conclusion
Python has proven to be an incredibly versatile tool in various business applications, enabling businesses to achieve greater efficiency, automation, and streamlined operations. Its applications in data analysis, web development, machine learning, automation, financial analysis, natural language processing, IoT, and database management demonstrate its vast reach.
By leveraging Python and its extensive libraries, businesses can enhance their operations, improve data analysis and visualization, build robust web applications, develop intelligent systems, automate repetitive tasks, perform financial analysis, and manage databases effectively. Python’s simplicity, versatility, and efficiency make it a valuable tool for businesses seeking to remain competitive in today’s rapidly evolving and technologically driven environment.
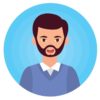
Matthew is a technical author with a passion for software development and a deep expertise in Python. With over 20 years of experience in the field, he has honed his skills as a software development manager at prominent companies such as eBay, Zappier, and GE Capital, where he led complex software projects to successful completion.
Matthew’s deep fascination with Python began two decades ago, and he has been at the forefront of its development ever since. His experience with the language has allowed him to develop a keen understanding of its inner workings, and he has become an expert at leveraging its unique features to build elegant and efficient software solutions.
Matthew’s academic background is rooted in the esteemed halls of Columbia University, where he pursued a Master’s degree in Computer Science.
As a technical author, Matthew is committed to sharing his knowledge with others and helping to advance the field of computer science. His contributions to the scientific computer science community are invaluable, and his expertise in Python development has made him a sought-after speaker and thought leader in the field.