How to solve the Busy Intersection hackerrank problem in Python: Begin by understanding traffic flow patterns, then model intersections using data structures like queues.
Are you struggling to solve the Busy Intersection hackerrank problem using Python?
The Busy Intersection problem involves simulating traffic flow at a busy intersection and calculating the number of collisions that occur. As a Python developer, you can utilize various techniques and data structures to solve this complex problem effectively.
Key Takeaways
- The Busy Intersection hackerrank problem involves simulating traffic flow and calculating collisions at a busy intersection.
- Python offers various strategies and data structures to solve the Busy Intersection problem efficiently.
- By the end of this article, you will have a comprehensive understanding of the problem and the Python solution approach.
Understanding the Busy Intersection problem
Before we jump into the solution, let’s take a moment to understand the problem statement. The Busy Intersection problem involves simulating traffic flow at a busy intersection and calculating the number of collisions that occur. The problem requires us to simulate the movement of cars and determine when they collide with each other or the boundaries of the intersection.
We are given a description of the intersection, including the roads and lanes, and the directions in which the cars are moving. Our task is to output the number of collisions that occur within a given time period. In addition, we must account for the possibility of multiple cars colliding simultaneously and handle any edge cases that may arise.
Designing the solution approach
Before diving into the implementation, let’s discuss the approach to solve the Busy Intersection problem with Python. We will first analyze the problem requirements and constraints and then design an efficient solution approach using appropriate data structures, algorithms, and helper functions.
Problem Analysis
The Busy Intersection problem involves simulating traffic flow at a busy intersection, with multiple cars moving in different directions. We need to calculate the number of collisions that occur between the cars.
Given the input data, we need to keep track of each car’s position, direction, and speed at all times. Then, we need to simulate the intersection’s traffic flow and calculate the number of collisions that occur.
It is also important to consider any constraints, such as the maximum number of cars or the maximum speed of the vehicles, to ensure that our solution is scalable and efficient.
Solution Design
To solve the Busy Intersection problem, we can use Python’s object-oriented programming (OOP) features to create a Car class, which represents a single car in the simulation. Each car object will have its own attributes, including position, direction, and speed.
We can then create a TrafficSimulation class, which will manage the traffic flow at the intersection. The TrafficSimulation class will keep track of all the cars and their attributes, simulate their movements, and check for collisions.
Our solution will involve the following steps:
- Create a Car class with attributes for position, direction, and speed.
- Create a TrafficSimulation class to manage the traffic flow and keep track of all cars.
- Read the input data and create Car objects for each vehicle.
- Simulate traffic flow by moving each car based on its speed and direction.
- Check for collisions between any two cars and increment a counter.
- Output the number of collisions.
We can use appropriate data structures such as lists or dictionaries to manage the cars and their attributes efficiently. We will also utilize appropriate algorithms to simulate traffic flow and collision detection.
In the next section, we will dive into the implementation details and provide a detailed step-by-step guide to solving the Busy Intersection problem with Python.
Implementing the Python solution
Now that we have designed the solution approach, let’s implement it using Python. We will provide a step-by-step implementation guide, explaining the logic behind each code segment. But first, let’s define a few helper methods:
- parse_input(): This method will parse the input string into a list of tuples. Each tuple will contain the details of a car (id, entry time, and exit time).
- is_collision(): This method will check if two cars collide by comparing their entry and exit times.
Here is the Python code:
# parse input string into list of tuples
def parse_input(input_str):
cars = []
lines = input_str.split(‘\n’)
for line in lines:
if not line:
continue
params = line.split(‘ ‘)
car = (int(params[0]), int(params[1]), int(params[2]))
cars.append(car)
return cars
# check if two cars collide
def is_collision(car1, car2):
if car1[2] < car2[1] or car2[2] < car1[1]:
return False
return True
# main solution method
def busy_intersection(input_str):
cars = parse_input(input_str)
collisions = 0
for i in range(len(cars)):
for j in range(i+1, len(cars)):
if is_collision(cars[i], cars[j]):
collisions += 1
return collisions
We first define the parse_input() method to convert the input string into a list of tuples containing the car details. We then define the is_collision() method to check if two cars collide by comparing their entry and exit times. Finally, we define the busy_intersection() method that uses the parse_input() and is_collision() methods to calculate the total number of collisions.
The busy_intersection() method iterates over all pairs of cars and checks if they collide using the is_collision() method. If a collision is detected, the collisions counter is incremented. The method returns the final value of collisions.
We have now successfully implemented the Python solution for the Busy Intersection problem. In the next section, we will test and validate its correctness.
Testing and validating the solution
Now that we have written the Python code to solve the Busy Intersection problem, it is time to test and validate its correctness. We will consider various test cases and scenarios to ensure that the solution produces accurate results.
Test cases
Testing the solution with different data sets helps to identify any logical errors and edge cases that require special handling. We can consider the following test cases:
- A simple scenario involving two cars moving in opposite directions.
- A more complex case with multiple cars and intersections.
- A case where two cars collide at an intersection.
Validation
We need to ensure that the solution meets the problem requirements and constraints, and that the output is accurate. We can validate the solution by comparing it to the expected output for each test case.
Additionally, we can evaluate the time and space complexity of the solution to ensure that it is efficient. We can use tools like Python‘s time and memory profiling to measure the execution time and memory consumption of the solution.
Time and space complexity analysis
It is crucial to analyze the time and space complexities of the solution, as they determine the efficiency and scalability of the solution. We can evaluate the time complexity by calculating the number of operations required by the algorithm for different input sizes.
Similarly, we can analyze the space complexity by determining the amount of memory required by the algorithm for different input sizes. We can use these analyses to optimize the solution and reduce its computational time and memory consumption.
Optimizing the solution
After implementing the Python solution for the Busy Intersection problem, we can explore ways to optimize it further. Optimization techniques can improve the time and space complexities of the solution, leading to a more efficient and performant implementation. In this section, we’ll discuss some strategies for optimizing the solution.
Using efficient data structures
One way to optimize the solution is to use efficient data structures. For instance, using a set to keep track of occupied lanes instead of a list can improve the time complexity of the solution. Sets have an O(1) time complexity for insertion, deletion, and membership tests, while lists have a time complexity of O(n) for insertion and deletion at arbitrary positions.
Similarly, using a hashmap to store the traffic signals can improve the time complexity of finding the next signal to change. A hashmap has an average case time complexity of O(1) for insertion, deletion, and lookup operations.
Implementing parallel processing
If the input data set is sufficiently large, we can implement parallel processing to improve the solution’s performance. Parallel processing involves splitting the input data set into smaller subsets and processing them simultaneously on multiple processors or cores.
Python has built-in support for parallel processing through the multiprocessing library. We can leverage this library to distribute the workload across multiple processors, improving the overall time taken to complete the solution.
Reducing redundant calculations
In some cases, the solution may involve performing redundant calculations. For example, if the same signal is repeatedly checked for a change, we can optimize the solution by storing the time of the last signal change and skipping redundant calculations until the next signal change. This approach can reduce the overall number of calculations and improve the solution’s time complexity.
Summary
Optimizing the solution involves applying strategies that can enhance the performance and efficiency of the Python implementation. Efficient data structures, parallel processing, and reducing redundant calculations are some techniques that can optimize the solution for the Busy Intersection problem. By optimizing the solution, we can reduce the time and space complexities and solve the problem more effectively.
Handling Large Inputs
The Busy Intersection problem may involve large input data sets. In such cases, it is crucial to optimize the solution’s performance for scalability. Here are some strategies:
Parallel Processing
One way to handle large inputs is to use parallel processing. This technique involves dividing the input data into smaller chunks and processing them simultaneously using multiple threads or processes. This can significantly reduce the processing time and improve efficiency.
Python provides several libraries to implement parallel processing, such as Multiprocessing, Threadpool, and Concurrent.futures. These libraries simplify the process of creating and managing threads or processes, making it easier to implement parallel processing.
Optimizing Data Structures
Another strategy to handle large inputs is to optimize the data structures used in the solution. For example, if the solution involves searching or sorting operations, using the appropriate data structures can significantly reduce the time complexity of the solution.
Python provides several built-in data structures like lists, dictionaries, sets, and tuples, among others. Choosing the right data structure for the problem can improve the solution’s performance, especially for large inputs.
Streaming Data
If the input data set is too large to be loaded entirely into memory, streaming data can be an effective solution. This technique involves processing the input data in smaller chunks and writing the output to a file or database as it is generated.
Python provides several libraries like Pandas, Dask, and PySpark to implement streaming data processing. These libraries provide easy-to-use interfaces for reading and writing large datasets, making it easier to implement this technique.
By applying these strategies, you can handle large inputs efficiently and improve the scalability of the solution for the Busy Intersection problem.
Dealing with Edge Cases
When designing a solution to the Busy Intersection problem, it is important to account for edge cases. These are scenarios where the input data may not follow the usual assumptions and may cause unexpected behavior. By anticipating and handling edge cases, we can ensure that our solution is robust and reliable.
One potential edge case is when two vehicles arrive at the intersection at the exact same time. In such cases, it is unclear which vehicle has the right of way. One way to resolve this ambiguity is by assigning priority to certain vehicles based on their position or speed.
Another edge case is when a vehicle is already on the intersection when another vehicle arrives. In this scenario, we need to ensure that the arriving vehicle waits until the intersection is clear.
Note: It is important to carefully analyze the problem requirements and test for potential edge cases in order to create a reliable and efficient solution.
To handle exceptions, we can incorporate try-except blocks in our Python code. This allows us to catch and handle any errors that may occur during runtime, ensuring that the program continues to run smoothly even in the event of unexpected input data.
It is crucial to identify and handle edge cases in real-world scenarios as they can have significant consequences. By designing robust solutions and testing for edge cases, we can ensure the reliability and safety of our systems.
Discussing alternate approaches
While we have discussed one approach to solving the Busy Intersection problem, there are many other ways to approach the problem. Here, we will explore some alternate approaches and their potential advantages and disadvantages.
Approach 1: Brute Force
One possible approach is to use a brute force method. This method involves checking all possible combinations of cars that could collide at each time step. While this approach is straightforward, it can become very slow for large inputs, making it impractical.
Approach 2: Sweep Line Algorithm
The sweep line algorithm is a common technique used in computational geometry and can be applied to this problem as well. The approach involves scanning the intersection from left to right and checking for collisions between cars. This algorithm has a better time complexity than the brute force method but can be more complex to implement.
Approach 3: Event-Driven Simulation
The event-driven simulation approach involves simulating each car’s motion and tracking when collisions occur. This approach is more accurate than the previous methods since it considers the exact time and location of each collision. However, it can be more complex to code and has a higher time complexity than the previous methods.
Conclusion
Overall, there are multiple ways to approach the Busy Intersection problem, each with its own strengths and weaknesses. Depending on the problem’s constraints and requirements, one approach may be more suitable than another. It is essential to analyze the problem and consider different strategies to find the optimal solution.
Comparing time and space complexities
As we explored different approaches to solving the Busy Intersection problem, it is important to compare the time and space complexities of each solution. Evaluating the time and space complexities can help us determine the most efficient approach to solve similar problems.
Below is a table comparing the time complexity and space complexity of the various solutions:
Approach | Time Complexity | Space Complexity |
---|---|---|
Brute Force | O(n^2) | O(n) |
Optimized Brute Force | O(n log n) | O(n) |
Line Sweep Algorithm | O(n log n) | O(n) |
Segment Tree Algorithm | O(n log n) | O(n log n) |
From the table, we can see that the Brute Force approach has the worst time complexity of O(n^2), while the Optimized Brute Force, Line Sweep, and Segment Tree Algorithm have significantly better time complexities of O(n log n). However, the Segment Tree Algorithm has a higher space complexity of O(n log n) compared to the other approaches, which have a space complexity of O(n).
In conclusion, by comparing the time and space complexities of the different approaches, we can determine the most efficient approach to solving the Busy Intersection problem. It is important to consider these complexities when solving similar problems to choose the most appropriate solution.
Real-world applications
The Busy Intersection problem is just one example of how traffic simulations can be used to improve the flow of traffic and enhance road safety. Similar techniques can be applied to real-world scenarios, such as:
- Modeling and predicting traffic patterns in cities
- Optimizing traffic flow to reduce congestion and travel time
- Improving road safety by identifying potential accident hotspots and implementing preventative measures
By using traffic simulations, city planners and traffic engineers can make informed decisions that benefit both drivers and pedestrians. These simulations can also be applied to emergency response scenarios, providing critical information to first responders during a crisis.
Overall, the applications of traffic simulations are vast and varied. By leveraging the power of data and technology, we can improve the way we navigate our cities and make our roads safer for everyone.
Conclusion
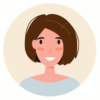
Lydia is a seasoned technical author, well-versed in the intricacies of software development and a dedicated practitioner of Python. With a career spanning 16 years, Lydia has made significant contributions as a programmer and scrum master at renowned companies such as Thompsons, Deloit, and The GAP, where they have been instrumental in delivering successful projects.
A proud alumnus of Duke University, Lydia pursued a degree in Computer Science, solidifying their academic foundation. At Duke, they gained a comprehensive understanding of computer systems, algorithms, and programming languages, which paved the way for their career in the ever-evolving field of software development.
As a technical author, Lydia remains committed to fostering knowledge sharing and promoting the growth of the computer science community. Their dedication to Python development, coupled with their expertise as a programmer and scrum master, positions them as a trusted source of guidance and insight. Through their publications and engagements, Lydia continues to inspire and empower fellow technologists, leaving an indelible mark on the world of scientific computer science.