It’s crucial to safeguard digital assets from potential threats such as cybercrime, data breaches, or unauthorized access. That’s why security measures should be a top priority for any software development project.
Python, one of the most popular programming languages in the world, offers various security capabilities that can be leveraged to ensure protection.
Understanding Python’s Security Features
Python is often touted as one of the most secure programming languages. This is in part due to its built-in security features that enable developers to create secure code. By adhering to secure coding practices, developers can safeguard their digital assets and prevent cyber attacks.
One of the key security features of Python is its automatic memory management. This means that developers don’t have to worry about manually managing memory and can avoid common memory-related vulnerabilities such as buffer overflows and null pointer dereferences.
Another important feature is Python’s ability to handle exceptions. Exceptions occur when something goes wrong in the code, and Python’s exception handling mechanisms allow for graceful error handling and the prevention of unintended consequences.
Python also has a strong focus on code readability and maintainability. By using clear, well-structured code, developers can more easily identify and fix potential security vulnerabilities.
When it comes to secure coding practices, there are several best practices that developers should follow:
- Ensure that all user input is validated and sanitized to prevent injection attacks
- Use encryption to protect sensitive data
- Implement secure authentication and authorization mechanisms
- Regularly update and patch software to address security vulnerabilities
Understanding Python’s Security Features in Action
To illustrate the effectiveness of Python’s security features, let’s compare it to another programming language, C++. In C++, developers must manually manage memory, which can lead to memory-related vulnerabilities. Additionally, exception handling in C++ can be complex and error-prone. By contrast, Python’s automatic memory management and exception handling make it easier to write secure code.
Let’s consider an example of secure coding in Python. When handling user input, it’s important to validate and sanitize it to prevent injection attacks.
Here’s an example of input validation and sanitization in Python:
import re
def validate_email(email):
email_regex = re.compile(r'^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$')
if not email_regex.match(email):
raise ValueError('Invalid email address')
return email
In this example, the code uses a regular expression to validate that the email address given is in the correct format. If the input doesn’t match the pattern, the code raises a ValueError. This is an example of secure coding in Python that helps prevent injection attacks.
Overall, Python’s security features make it a popular choice for developers who want to ensure the security of their applications. By following best practices and using secure coding techniques, developers can create secure and reliable software.
Common Security Vulnerabilities in Python
Python is a versatile and powerful programming language often used in developing web and mobile applications. Like with any other programming language, Python has inherent security risks that developers must be aware of to avoid common security vulnerabilities. In this section, we’ll explore some of the most common security flaws found in Python applications.
1. Injection Attacks
Python web applications are vulnerable to injection attacks, where an attacker leverages unvalidated user input to execute malicious code on the server. SQL injection attacks are the most prevalent injection attacks. In such an attack, an attacker inputs malicious SQL code in a form field, which is then executed on the server. This can result in data theft and/or loss. Prevent injection attacks by validating user input and using parameterized queries.
2. Cross-Site Scripting (XSS) Attacks
XSS attacks are another common vulnerability found in Python applications. Hackers inject malicious JavaScript code into a web page that unsuspecting users visit, then steal data or gain access to user accounts. XSS attacks are possible because developers don’t always sanitize user input properly before displaying it in a web page. To prevent XSS attacks, always sanitize user input and never trust user-generated content.
3. Authentication and Authorization Issues
Authentication and authorization flaws can allow hackers to gain unauthorized access to secure parts of a Python application. Weak passwords, hardcoded credentials, and user enumeration are common authentication issues in Python. Authorization issues include insufficient permissions and access control checks, which can allow hackers to perform functions they shouldn’t be able to. Developers can prevent authentication issues by enforcing strong password policies, using multi-factor authentication, and implementing robust session management. Authorization issues can be mitigated by applying the principle of least privilege and ensuring access controls are appropriate and enforced.
4. Security Misconfigurations
Security misconfigurations occur when developers don’t configure their applications or servers correctly. Misconfigurations can give an attacker unauthorized access to sensitive data or systems. This can happen when web servers are misconfigured, such as leaving default usernames and passwords or outdated software on the server. Developers can prevent security misconfigurations by regularly patching software and performing regular security checks and audits.
5. Cryptography Flaws
Python provides built-in modules for cryptographic functions such as hashing and encryption. However, if these functions are used improperly, they can lead to cryptography flaws. For example, using weak encryption algorithms can make sensitive data easily readable. To prevent cryptography flaws, developers should understand cryptography principles and use only well-tested and validated cryptographic libraries and functions.
By being aware of these common security vulnerabilities, developers can build secure and robust Python applications. Implementing secure coding practices and leveraging Python’s security features are critical to safeguarding digital assets from malicious attacks.
Secure Authentication and Authorization in Python
Authentication and authorization are crucial components of software security. Python offers a range of techniques and libraries for implementing secure authentication and authorization mechanisms.
One popular library for managing user authentication is Flask-Login. This library provides user session management, handles user authentication, and includes safe password hashing.
Here’s an example of how to use Flask-Login:
from flask_login import LoginManager, UserMixin, login_user, login_required, logout_user
app = Flask(__name__)
login_manager = LoginManager()
login_manager.init_app(app)
class User(UserMixin):
pass
@login_manager.user_loader
def user_loader(user_id):
return User.get(user_id)
@app.route('/login', methods=['GET', 'POST'])
def login():
if request.method == 'GET':
return render_template('login.html')
username = request.form['username']
password = request.form['password']
user = User.get(username)
if user and hash_password(password) == user.password:
login_user(user)
return redirect(url_for('protected'))
@app.route('/protected')
@login_required
def protected():
return 'Logged in as: ' + current_user.username
@app.route('/logout')
@login_required
def logout():
logout_user()
return 'Logged out'
Another popular library for managing authorization in Python is Flask-JWT. This library provides JSON Web Token (JWT) authentication for Flask APIs.
Here’s an example of using Flask-JWT:
from flask_jwt import JWT, jwt_required, current_identity
from werkzeug.security import safe_str_cmp
class User:
def __init__(self, id, username, password):
self.id = id
self.username = username
self.password = password
users = [User(1, 'user1', 'password1'), User(2, 'user2', 'password2')]
username_table = {u.username: u for u in users}
userid_table = {u.id: u for u in users}
def authenticate(username, password):
user = username_table.get(username, None)
if user and safe_str_cmp(user.password.encode('utf-8'), password.encode('utf-8')):
return user
def identity(payload):
user_id = payload['identity']
return userid_table.get(user_id, None)
jwt = JWT(app, authenticate, identity)
@app.route('/protected')
@jwt_required()
def protected():
return 'Logged in as: ' + current_identity.username
As with all security measures, it’s important to keep up to date with the latest techniques and best practices to ensure your applications are protected against evolving threats.
Data Encryption and Protection with Python
Data encryption and protection have become critical aspects of software development, especially with the rise of cyber threats and data breaches. Python offers a range of security libraries and frameworks, making it an excellent choice for developers who need to safeguard sensitive information.
One popular Python library for data encryption is Cryptography. Cryptography features a collection of algorithms and protocols that can be used to encrypt and decrypt data securely.
Here’s an example of how to encrypt a message using the Advanced Encryption Standard (AES) algorithm:
# Import the necessary libraries
from cryptography.fernet import Fernet
# Generate a key
key = Fernet.generate_key()
# Create a Fernet instance using the key
fernet = Fernet(key)
# Encrypt the message
encrypted_message = fernet.encrypt(b"My secret message")
To decrypt the message, the same key and algorithm are used:
# Decrypt the message
decrypted_message = fernet.decrypt(encrypted_message)
# Convert bytes to string
message = decrypted_message.decode()
Another popular Python library for data protection is PyCryptodome. PyCryptodome is a self-contained Python package that provides cryptographic primitives and protocols for various purposes.
Here’s an example of how to use the SHA-256 algorithm to hash a password:
# Import the necessary libraries
from Crypto.Hash import SHA256
# Convert the password to bytes
password = "mysecurepassword".encode()
# Hash the password
hash_object = SHA256.new(data=password)
# Get the hashed password as a hex string
hashed_password = hash_object.hexdigest()
Using these libraries, Python developers can easily and securely handle sensitive data in their applications.
Securing Web Applications with Python
Web application security is critical in protecting digital assets from malicious attacks. Python provides a range of frameworks that can be used to develop secure web applications. In this section, we will explore the best practices for securing web applications using Python web frameworks.
Django vs Flask: Which is more Secure?
Django and Flask are two popular Python web frameworks used for building web applications. While both frameworks have security features, Django has more built-in security features than Flask.
Below is an example of how Django handles user authentication:
import django.contrib.auth as authenticate
user = authenticate(username=username, password=password)
if user is not None:
# User authenticated successfully
else:
# Invalid username or password
On the other hand, Flask requires the use of third-party libraries for user authentication.
Here’s an example of how Flask handles user authentication using Flask-Login:
from flask_login import LoginManager, UserMixin
login_manager = LoginManager()
login_manager.init_app(app)
class User(UserMixin):
pass
@login_manager.user_loader
def load_user(user_id):
return User.get(user_id)
As you can see, using Flask requires more effort to implement user authentication. However, both frameworks are highly secure and are widely used in the industry.
Implementing HTTPS Encryption
HTTPS encryption is an essential security feature that protects web applications from eavesdropping and man-in-the-middle attacks. Python provides the ‘ssl’ module that can be used to enable HTTPS encryption in web applications.
Here is an example of how to use ‘ssl’ module with Flask:
from flask import Flask
import ssl
app = Flask(__name__)
context = ssl.SSLContext(ssl.PROTOCOL_TLSv1_2)
context.load_cert_chain(certfile='cert.pem', keyfile='key.pem')
if __name__ == '__main__':
app.run(ssl_context=context)
By providing the SSLContext object to the Flask run method, the application will be served over HTTPS.
Preventing Cross-Site Request Forgery (CSRF) Attacks
CSRF attacks are a type of security vulnerability that enables attackers to execute unauthorized actions on behalf of authenticated users. Python web frameworks such as Django provide built-in CSRF protection.
Here is an example of how prevent CSRF attacks using Django:
{% csrf_token %}
By including the {% csrf_token %} tag in the HTML form, Django ensures that the submitted data came from a legitimate source.
Python web frameworks provide a variety of security features that can be used to secure web applications. Django and Flask are both highly secure frameworks that can be used to develop secure web applications. Implementing HTTPS encryption, enabling CSRF protection, and using secure authentication mechanisms are essential security features that should be implemented in all web applications.
Protecting against Cross-Site Scripting (XSS) Attacks
Cross-site scripting (XSS) attacks are a common type of security vulnerability in web applications. They occur when an attacker injects malicious code into a web page viewed by other users. This code can steal sensitive information or manipulate the user’s session.
Python provides several security measures to prevent XSS attacks. One common technique is input sanitization, which involves removing any potentially harmful code from user input. The MarkupSafe library is a great example of a Python library that can be used for input sanitization.
MarkupSafe is a library for Python that makes it easy to use HTML, XML, and JSON in your code without worrying about XSS vulnerabilities. It automatically escapes any special characters in the input, making it safe for use in web applications.
Another technique for preventing XSS attacks in Python is to use a web framework that supports automatic input sanitization. For example, the Django web framework includes built-in protections against XSS attacks using its templating engine.
Here’s an example of how to use Django’s built-in protections against XSS:
from django.template import Context, Template, RequestContext
template = Template("Hello {{ name }}!")
context = RequestContext(request, {'name': 'alert("XSS Attack!");
'}) output = template.render(context)
In this example, the Template object is used to create a simple web page that displays a greeting to the user. The Context object is used to pass data to the template, including the user’s name. The RequestContext object is used to automatically sanitize the user’s name to prevent XSS attacks.
Other Python web frameworks, such as Flask and Pyramid, also provide built-in protections against XSS attacks. However, it’s important to note that these protections are not foolproof and should be used in conjunction with other security measures, such as input validation and data encryption.
Recommended Python Security Measures to Protect Against XSS Attacks:
- Use input sanitization libraries like MarkupSafe
- Choose a web framework that supports automatic input sanitization
- Implement additional security measures like input validation and data encryption
Managing Input Validation and Sanitization
One of the most common security vulnerabilities in software applications is the failure to properly validate and sanitize user input. Hackers can exploit this vulnerability to inject malicious code or data into the application, compromising its security. Python provides several techniques and libraries to manage input security.
Input validation is the process of ensuring that user input conforms to expected formats and types. Python provides built-in functions like isdigit()
and isalpha()
that can be used to validate input. Additionally, the re
module can be used to validate input using regular expressions.
Input sanitization is the process of removing potentially malicious characters or code from user input. Python provides the html
module, which can be used to escape and sanitize HTML input. Additionally, the bleach
library can be used to sanitize input by removing or escaping potentially dangerous characters and code.
It is important to note that input validation and sanitization should be used together to provide maximum security. Input validation alone may not be enough to prevent all forms of attacks, while input sanitization alone may not be effective in removing all potentially malicious input.
Examples of Input Validation and Sanitization in Python
Input Validation:
Code | Description |
---|---|
if not str.isalnum(input_string): | Validates that the input string only contains alphanumeric characters. |
if not re.fullmatch(r'^[a-zA-Z0-9_]{3,20}$', input_string): | Validates that the input string contains only alphanumeric characters, underscores, and is between 3 and 20 characters in length using regular expressions. |
Input Sanitization:
Code | Description |
---|---|
import html | Uses the html.escape() function to escape potentially dangerous characters in an input string. |
import bleach | Uses the bleach.clean() function to remove potentially dangerous HTML tags and attributes from an input string. |
Mitigating SQL Injection Attacks in Python
SQL Injection attacks have been one of the most common security threats to web applications. These attacks occur when an attacker inserts malicious SQL statements into the input fields of a web application, which can lead to the manipulation or destruction of your database and sensitive data.
Fortunately, Python provides several techniques and practices that can be implemented to prevent SQL Injection attacks.
Firstly, it is important to use parameterized queries to sanitize user input, which prevents SQL Injection attacks by separating user input from the SQL code. Python libraries, such as psycopg2 and MySQLdb, provide parameterized queries functionality.
Let’s take an example where a user is required to input their username and password to log in to a web application. A simple SQL statement might look like this:
SELECT * FROM users WHERE username= '$username' AND password= '$password';
However, if an attacker inputs ' OR 1=1 --
in the username field, the SQL statement would look like this:
SELECT * FROM users WHERE username='' OR 1=1 --' AND password= '$password';
This statement returns all the rows from the users table, including the sensitive data it contains.
By using parameterized queries in Python, the same statement would look like this:
SELECT * FROM users WHERE username= %s AND password= %s;
Then, by leveraging prepared statements, the same query would look like this:
SELECT * FROM users WHERE username= ? AND password= ?;
Both of these statements eliminate the possibility of SQL Injection attacks as the user input is sanitized and separated from the SQL code.
Another technique to consider is input validation. Validating user input can help mitigate the risk of SQL Injection attacks by ensuring that only acceptable types of input are accepted. Regular expressions in Python are a useful technique for input validation.
SQL Injection attacks can pose a significant threat to the security of your Python web applications and data. By implementing parameterized queries and input validation techniques, you can help prevent such attacks.
Continuous Monitoring and Threat Detection
Ensuring the ongoing security of your Python applications requires continuous monitoring and threat detection. This means regular review of access logs, server performance, and application behavior to detect potential vulnerabilities and threats.
Fortunately, there are a number of Python security tools and frameworks that can help automate the monitoring process to provide better protection and reduce the risk of a security breach.
Python Security Tools for Continuous Monitoring
Tool | Description |
---|---|
Wazuh | A security detection, visibility, and compliance open source project that includes a number of tools and features for monitoring and analyzing potential security threats. |
Fail2ban | A log analysis and intrusion prevention framework that blocks IP addresses after a specified number of failed authentication attempts. Fail2ban can be integrated with Python applications to provide automated protection. |
Splunk | A popular security information and event management (SIEM) tool that provides real-time threat detection and alerting features. Splunk can be used to monitor and analyze logs from Python applications. |
Integration of such tools with your Python application can provide ongoing protection and peace of mind, even as the application evolves and grows over time.
Implementing Threat Detection with Python
Another way to detect threats is to implement threat detection mechanisms directly within the Python application itself. One approach is to use a Python framework like Scikit-learn to analyze incoming data and identify potential threats. Another option is to use Python libraries like Scapy to monitor network traffic and detect suspicious patterns.
By implementing these threat detection mechanisms, your application can quickly respond to potential security threats, reducing the likelihood of an attack and minimizing the impact if one does occur.
Remember, continuous monitoring and threat detection are critical components of effective security measures. By leveraging Python security tools and implementing threat detection mechanisms, you can better safeguard your digital assets against potential threats.
Securing APIs with Python
APIs have become the backbone of modern web applications, providing a way for systems to communicate with each other. However, they also introduce security risks that need to be addressed. Building secure APIs is essential in safeguarding digital assets and ensuring the protection of user data. Python offers several security solutions that can help developers create secure APIs.
API Security Considerations
When designing and implementing APIs with Python, several security considerations need to be taken into account. First and foremost, API endpoints need to be secured against unauthorized access. This can be achieved by implementing secure authentication and authorization mechanisms.
APIs also need to be protected against common attacks such as cross-site scripting (XSS), SQL injection, and other vulnerabilities that can compromise the security of the system.
Furthermore, API security should include rate limiting to prevent resource exhaustion attacks and limit the number of requests an API consumer can make in a given time frame.
Python Security Solutions for API Security
Python provides several security solutions that can be utilized for securing APIs.
Flask-RESTful
Pros | Cons |
---|---|
Easy to use and flexible | Limited built-in security features |
Provides support for authentication and authorization mechanisms | Not suitable for large-scale applications |
Django REST framework
Pros | Cons |
---|---|
Built-in support for authentication and authorization mechanisms | Can be complex to set up and configure |
Provides comprehensive security features | Not suitable for small projects or quick prototyping |
Both Flask-RESTful and Django REST framework provide support for secure authentication and authorization mechanisms. They also include features to prevent common attacks such as XSS and SQL injection.
Authentication and Authorization in Flask-RESTful
Flask-RESTful provides a simple way to add authentication and authorization mechanisms to API endpoints. The most common way to implement authentication in Flask-RESTful is by using JSON Web Tokens (JWTs).
JWTs are a secure way to transmit user authentication data between the client and the server. Once a user logs in, they receive a JWT that is included in all subsequent requests to the API.
Authorization can be implemented by using Flask-RESTful’s built-in decorators such as @jwt_required. These decorators can be used to restrict access to certain endpoints based on the user’s role or permissions.
Authentication and Authorization in Django REST framework
Django REST framework provides built-in support for several authentication and authorization mechanisms.
The most commonly used authentication method is token authentication. Token authentication works by generating a unique token for each user that is included in all subsequent requests to the API.
Django REST framework also provides support for OAuth2 and JSON Web Tokens (JWTs).
Authorization can be implemented by using Django REST framework’s built-in permissions system. Permissions can be assigned to users or groups and can restrict access to specific API endpoints.
Securing APIs with Python is essential in ensuring the protection of digital assets and user data. Python provides several security solutions that can be utilized for securing APIs. Flask-RESTful and Django REST framework are both popular Python web frameworks that provide built-in support for secure authentication and authorization mechanisms, as well as other security features.
Conclusion: Unlocking Advanced Protection with Python Security
Hackers and cybercriminals are constantly evolving their tactics to gain unauthorized access to sensitive data, making it imperative for companies to safeguard their digital assets. Python, with its advanced security capabilities, offers expert security solutions to ensure protected software.
FAQ
Q: What are Python security solutions?
A: Python security solutions are measures and techniques used to safeguard digital assets and protect against security threats in software developed using the Python programming language.
Q: What are the security capabilities of Python?
A: Python provides built-in security features and libraries that can be utilized to enhance the security of applications. These features include secure coding practices, data encryption, authentication and authorization mechanisms, and more.
Q: What are common security vulnerabilities in Python?
A: Common security vulnerabilities in Python applications include SQL injection attacks, cross-site scripting (XSS) attacks, insecure input validation, and inadequate data encryption. It is important to be aware of these vulnerabilities and take appropriate measures to mitigate them.
Q: How can I implement secure authentication and authorization in Python?
A: Python offers various techniques and libraries for implementing secure authentication and authorization mechanisms. Examples include using secure password hashing algorithms, implementing multi-factor authentication, and utilizing secure session management.
Q: What Python libraries can I use for data encryption and protection?
A: Python provides popular libraries and frameworks such as Cryptography and PyCryptodome for data encryption and protection. These libraries offer a range of cryptographic functions and algorithms to secure sensitive data.
Q: How can I secure web applications developed using Python?
A: Securing web applications in Python involves following best practices such as input validation and sanitization, secure session management, implementing secure communication protocols (HTTPS), and utilizing secure coding practices. It is also important to choose web frameworks with built-in security features.
Q: How can I protect against cross-site scripting (XSS) attacks in Python?
A: To protect against XSS attacks, it is important to sanitize and validate user input, properly encode output when displaying user-generated content, and implement strict content security policies. Utilizing security libraries and frameworks like Django can further enhance protection against XSS attacks.
Q: What is input validation and sanitization in Python?
A: Input validation and sanitization involve checking and cleaning user input to prevent security vulnerabilities such as SQL injection attacks and cross-site scripting. It ensures that the input is in the expected format and does not contain malicious code.
Q: How can I mitigate SQL injection attacks in Python?
A: Mitigating SQL injection attacks in Python involves using parameterized queries or prepared statements instead of concatenating user input directly into SQL queries. It is also essential to validate and sanitize user input to prevent any malicious SQL code injection.
Q: Why is continuous monitoring and threat detection important in Python?
A: Continuous monitoring and threat detection are crucial for maintaining the security of Python applications. They help identify and respond to potential security threats in real-time, allowing for timely remediation actions. Python offers various security tools and frameworks for automated monitoring.
Q: How can I secure APIs developed using Python?
A: Securing APIs in Python involves implementing authentication mechanisms such as OAuth or token-based authentication, applying rate limiting to prevent abuse, encrypting sensitive data exchanged over the API, and conducting thorough input validation on API endpoints.
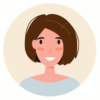
Lydia is a seasoned technical author, well-versed in the intricacies of software development and a dedicated practitioner of Python. With a career spanning 16 years, Lydia has made significant contributions as a programmer and scrum master at renowned companies such as Thompsons, Deloit, and The GAP, where they have been instrumental in delivering successful projects.
A proud alumnus of Duke University, Lydia pursued a degree in Computer Science, solidifying their academic foundation. At Duke, they gained a comprehensive understanding of computer systems, algorithms, and programming languages, which paved the way for their career in the ever-evolving field of software development.
As a technical author, Lydia remains committed to fostering knowledge sharing and promoting the growth of the computer science community. Their dedication to Python development, coupled with their expertise as a programmer and scrum master, positions them as a trusted source of guidance and insight. Through their publications and engagements, Lydia continues to inspire and empower fellow technologists, leaving an indelible mark on the world of scientific computer science.