In today’s fast-paced technological landscape, artificial intelligence (AI) is making groundbreaking innovations in various industries. Python, a high-level programming language, has emerged as the preferred language for building AI solutions.
Python’s simplicity, extensive libraries, and community support make it a top choice for expert Python AI development and leveraging Python for intelligent AI projects.
Understanding Python’s Role in AI Development
Python is fast becoming the go-to language for artificial intelligence (AI) development, thanks to its simplicity, readability, and extensive library support. Furthermore, Python has a wide range of frameworks for AI development, making it a natural choice for building intelligent systems.
Python’s Advantages in AI Development
Python’s readability makes it easy for developers to write and maintain code, increasing productivity. Its extensive libraries offer pre-built functions and modules for tasks such as data manipulation, visualization, and machine learning. Python libraries such as NumPy, Pandas, and Matplotlib are widely used in data science and AI projects. In addition, Python’s large and active community contributes to constantly improving libraries and frameworks.
Python Frameworks for AI Development
Python has several frameworks for building AI solutions that leverage its powerful libraries. Two of the most popular frameworks are TensorFlow and PyTorch. TensorFlow is an open-source library for dataflow and differentiable programming across a range of tasks. PyTorch, on the other hand, is a popular deep learning framework, allowing for seamless GPU acceleration with tensor computations. Other popular Python frameworks for AI development include scikit-learn, Keras, and Theano.
Framework | Features |
---|---|
TensorFlow | Scalable, flexible, and portable |
PyTorch | Easy debugging, seamless GPU acceleration |
scikit-learn | Machine learning algorithms, easy data preprocessing |
Keras | High-level neural networks API, easy to use |
Theano | Mathematical operations, GPU optimization |
When comparing frameworks, it is important to consider factors such as ease of development, scalability, and performance. For instance, TensorFlow is favored for its scalability, while PyTorch’s intuitive API makes it accessible to beginners. Developers can use these frameworks to build intelligent applications for image recognition, natural language processing, and predictive analytics.
Key Concepts in Python AI Development
Python is a versatile programming language that is widely used in the field of artificial intelligence. Developers can leverage Python’s simplicity and powerful libraries to build intelligent systems and applications. In this section, we will cover some of the key concepts and techniques used in Python AI development.
Machine Learning
Machine learning is a subfield of AI that focuses on enabling machines to learn from data and make predictions or decisions without being explicitly programmed. Python has several libraries that facilitate machine learning, including scikit-learn, TensorFlow, and PyTorch.
Here is an example of some sample code that uses scikit-learn to train a simple logistic regression model on a dataset of iris flowers:
import numpy as np
from sklearn.linear_model import LogisticRegression
from sklearn.model_selection import train_test_split
from sklearn.datasets import load_iris
# load the iris dataset
iris = load_iris()
X = iris.data
y = iris.target
# split the data into training and test sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# train a logistic regression model on the training set
clf = LogisticRegression(random_state=42)
clf.fit(X_train, y_train)
# evaluate the model on the test set
accuracy = clf.score(X_test, y_test)
print("Test set accuracy: {:.2f}".format(accuracy))
This code loads the iris dataset, splits it into training and test sets, trains a logistic regression model on the training set, and evaluates the model’s accuracy on the test set.
Neural Networks
Neural networks are another key component of AI and machine learning, and Python has several libraries that facilitate building and training neural networks. One such library is Keras, which provides a high-level API for building and training neural networks.
Here is an example of some sample code that uses Keras to build a simple feedforward neural network for classifying images in the MNIST dataset:
import keras
from keras.datasets import mnist
from keras.models import Sequential
from keras.layers import Dense, Dropout, Flatten
from keras.layers import Conv2D, MaxPooling2D
from keras import backend as K
# load the MNIST dataset
(x_train, y_train), (x_test, y_test) = mnist.load_data()
# reshape the data and normalize it
x_train = x_train.reshape(x_train.shape[0], 28, 28, 1)
x_test = x_test.reshape(x_test.shape[0], 28, 28, 1)
x_train = x_train.astype('float32') / 255
x_test = x_test.astype('float32') / 255
# convert the labels to one-hot encoding
y_train = keras.utils.to_categorical(y_train, 10)
y_test = keras.utils.to_categorical(y_test, 10)
# build the neural network
model = Sequential()
model.add(Conv2D(32, kernel_size=(3, 3), activation='relu', input_shape=(28, 28, 1)))
model.add(MaxPooling2D(pool_size=(2, 2)))
model.add(Flatten())
model.add(Dense(128, activation='relu'))
model.add(Dense(10, activation='softmax'))
# compile the model
model.compile(loss=keras.losses.categorical_crossentropy, optimizer=keras.optimizers.Adam(), metrics=['accuracy'])
# train the model
model.fit(x_train, y_train, batch_size=128, epochs=10, validation_data=(x_test, y_test))
# evaluate the model
score = model.evaluate(x_test, y_test, verbose=0)
print('Test loss:', score[0])
print('Test accuracy:', score[1])
This code loads the MNIST dataset, reshapes and normalizes the data, converts the labels to one-hot encoding, builds a feedforward neural network with two hidden layers and one output layer, compiles the model, trains the model on the training set, and evaluates the model’s performance on the test set.
Natural Language Processing
Natural Language Processing (NLP) is a subfield of AI that focuses on enabling computers to understand, interpret, and interact with human language. Python has several libraries that facilitate NLP, including NLTK, spaCy, and TextBlob.
Here is an example of some sample code that uses NLTK to perform sentiment analysis on a sample text:
import nltk
from nltk.sentiment import SentimentIntensityAnalyzer
# create a sentiment analyzer
sia = SentimentIntensityAnalyzer()
# analyze a sample text
text = "I love this product! It has exceeded all my expectations."
sentiment = sia.polarity_scores(text)
# print the sentiment scores
print(sentiment)
This code creates a sentiment analyzer using NLTK’s SentimentIntensityAnalyzer class, analyzes a sample text for sentiment, and prints the sentiment scores.
Data Preprocessing
Data preprocessing is an important step in AI and machine learning that involves cleaning, transforming, and preparing data for analysis. Python has several libraries that facilitate data preprocessing, including NumPy, Pandas, and TensorFlow.
Here is an example of some sample code that uses NumPy to normalize a dataset of iris flowers:
import numpy as np
from sklearn.datasets import load_iris
# load the iris dataset
iris = load_iris()
X = iris.data
# normalize the data
X_norm = (X - np.min(X, axis=0)) / (np.max(X, axis=0) - np.min(X, axis=0))
# print the normalized data
print(X_norm)
This code loads the iris dataset, normalizes the data using NumPy, and prints the normalized data.
Exploring Python AI Frameworks
Python offers a range of powerful AI frameworks that facilitate the development of intelligent systems. Here we compare three popular frameworks: TensorFlow, PyTorch, and scikit-learn.
TensorFlow
TensorFlow is an open-source, versatile library developed by Google Brain Team for numerical computation and large-scale machine learning. It is widely used for developing neural networks and deep learning models. TensorFlow offers an extensive set of APIs for building, training, and deploying ML models. One of the significant features of TensorFlow is its ability to handle large datasets and distributed training.
Pros | Cons |
---|---|
– Large community support | – Steep learning curve |
– Robust production deployment | – Cumbersome syntax |
– High scalability | – Limited visualization options |
Below is an example of a TensorFlow code snippet for training a simple neural network:
import tensorflow as tf
model = tf.keras.Sequential([
tf.keras.layers.Dense(64, activation='relu', input_shape=(784,)),
tf.keras.layers.Dense(10)
])
model.compile(optimizer=tf.keras.optimizers.Adam(0.01),
loss=tf.keras.losses.CategoricalCrossentropy(from_logits=True),
metrics=['accuracy'])
model.fit(x_train, y_train, epochs=5, batch_size=32,
validation_data=(x_val, y_val))
PyTorch
PyTorch is a widely-used, open-source ML library developed by Facebook. PyTorch offers a dynamic computational graph, which makes it easier to debug and understand. It is known for its simplicity and flexibility, making it an ideal framework for rapid prototyping and research.
Pros | Cons |
---|---|
– Easy to debug and understand | – Limited built-in capabilities |
– Simple syntax and intuitive APIs | – Limited deployment options |
– Dynamic computation graph | – Smaller community support than TensorFlow |
Here’s an example of a PyTorch code snippet for training a simple neural network:
import torch
model = torch.nn.Sequential(
torch.nn.Linear(784, 64),
torch.nn.ReLU(),
torch.nn.Linear(64, 10)
)
loss_fn = torch.nn.CrossEntropyLoss()
optim = torch.optim.Adam(model.parameters(), lr=0.01)
for epoch in range(5):
for i, data in enumerate(train_dataloader, 0):
inputs, labels = data
optim.zero_grad()
outputs = model(inputs)
loss = loss_fn(outputs, labels)
loss.backward()
optim.step()
scikit-learn
scikit-learn is a popular, user-friendly ML library that offers a wide range of algorithms for supervised and unsupervised learning, as well as tools for model selection and evaluation. It is widely-used in industry and academia for building simple-to-complex ML models and systems. scikit-learn is built on top of NumPy and SciPy, both of which are important Python libraries for scientific computing.
Pros | Cons |
---|---|
– Simple, easy-to-use APIs | – Limited support for deep learning |
– Comprehensive documentation and resources | – Limited scalability |
– Versatile and diverse set of algorithms | – Limited deployment options |
Below is an example of a scikit-learn code snippet for building a simple decision tree classifier:
from sklearn.datasets import load_iris
from sklearn.tree import DecisionTreeClassifier
from sklearn.model_selection import train_test_split
iris = load_iris()
X_train, X_test, y_train, y_test = train_test_split(
iris.data, iris.target, stratify=iris.target, random_state=42
)
tree_clf = DecisionTreeClassifier(max_depth=2)
tree_clf.fit(X_train, y_train)
When choosing an AI framework, it’s important to consider your specific needs and use cases. TensorFlow is an excellent choice for building large, complex neural networks, while PyTorch is more suited for research and rapid prototyping. scikit-learn is a great choice for developing simple-to-medium complexity ML models.
Python vs. Other Languages for AI Development
Python is becoming increasingly popular for AI development, but how does it compare to other languages? Let’s take a closer look.
Advantages of Python in AI Development
Python’s popularity in AI development can be attributed to its simplicity, ease of use, and extensive libraries. Its syntax is easy to read and write, making it more accessible for beginners. Additionally, Python’s libraries provide a wide range of functionality for data manipulation, machine learning, and visualization, such as NumPy, Pandas, and Matplotlib.
Comparison with R
R is another popular language for data analysis and machine learning. While R has some strengths in statistical computing and visualizations, Python is generally more versatile and has a larger community. Python’s libraries provide more diverse functionality, and its syntax is generally considered easier to read and write.
For example, let’s compare the code for importing a CSV file in both languages:
R: data <- read.csv("filename.csv")
Python: import pandas as pd
data = pd.read_csv("filename.csv")
Comparison with Java
Java is another language widely used in AI development, particularly in building enterprise-level applications. However, Java’s syntax is more verbose and may require more lines of code to accomplish the same task as Python. Java also has fewer libraries and tools specifically designed for AI.
Let’s compare the code for printing “Hello, World!” in both languages:
Java: public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Python: print("Hello, World!")
While there are some advantages to using R or Java in AI development, Python’s versatility, ease of use, and extensive libraries make it the preferred choice for many.
Industry Applications of Python AI Development
Python AI development has seen widespread adoption across various industries, from healthcare to finance and marketing.
Here are some examples of how Python is being used to develop intelligent systems:
- Healthcare: Python is being used to build predictive models that can identify patients at risk of developing chronic conditions. For example, researchers at Stanford University used Python’s scikit-learn library to develop a model that can predict breast cancer risk with remarkable accuracy.
- Finance: Python is being used to build intelligent trading systems that can analyze market data and make predictions about future trends. For example, the investment management firm Two Sigma uses Python extensively to develop its trading algorithms.
- Marketing: Python is being used to build recommendation engines that can provide personalized product recommendations to customers. For example, Amazon uses Python to power its recommendation engine, which is responsible for generating 35% of the company’s revenue.
Recent trends in Python AI development include:
- Increased focus on ethical considerations: As AI becomes more prevalent in society, there is a growing need to ensure that it is used in an ethical and responsible manner. Python developers are increasingly focusing on issues like bias, fairness, and transparency to ensure that their AI systems are built with social responsibility in mind.
- Greater use of deep learning: Deep learning has emerged as a powerful technique for training highly accurate AI models. Python’s TensorFlow framework has played a significant role in popularizing deep learning, and many Python developers are now exploring the possibilities of advanced deep learning techniques like GANs and autoencoders.
- Integration with other emerging technologies: Python AI development is increasingly being integrated with other emerging technologies like the Internet of Things (IoT) and blockchain. For example, Python is being used to build intelligent IoT systems that can collect and analyze data from connected devices, while blockchain-based smart contracts are being used to automate AI decision-making processes.
Python AI Development Best Practices
Developing AI solutions with Python requires a particular set of best practices to ensure successful projects.
Here are some tips to help you:
1. Data Preparation
Preparing data is crucial to developing accurate AI models. It is essential to ensure that data is accurate, relevant, and sufficient for the model’s requirements. Use data cleaning techniques to identify and remove any inconsistent, irrelevant, or redundant data.
Example:
Data Before Cleaning | Data After Cleaning |
---|---|
Firstname,Lastname,Age,Gender,Salary,Country John,Doe,35,M,5000,USA Jane,Doe,?,?,?,UK Adam,Smith,42,M,6000,USA Sarah,Connor,36,F,5500,USA,Canada | Firstname,Lastname,Age,Gender,Salary,Country John,Doe,35,M,5000,USA Adam,Smith,42,M,6000,USA Sarah,Connor,36,F,5500,USA |
2. Model Selection
Choosing the right model is critical to the success of your AI project. Consider factors like accuracy, training time, complexity, and resource requirements when selecting a model.
Example:
- Linear Regression: useful for predicting continuous values, such as stock prices
- Decision Trees: useful for classification tasks with structured data, such as customer segmentation
- Convolutional Neural Networks: useful for image recognition tasks, such as self-driving cars
3. Hyperparameter Tuning
Hyperparameters are variables that affect model performance, like learning rate, regularization, and batch size. Tuning these parameters can significantly improve the accuracy of your model.
Example:
Hyperparameter | Value | Accuracy |
---|---|---|
Learning Rate | 0.1 | 82% |
Learning Rate | 0.01 | 89% |
Learning Rate | 0.001 | 92% |
4. Deployment Considerations
Consider deployment early in the development process to ensure that your AI system can be implemented and maintained efficiently. Factors to consider include scalability, security, and integration with existing systems.
Example:
“When deploying an AI system in healthcare, it is essential to comply with the necessary regulations to ensure patient privacy and safety.”
By implementing these best practices, you can ensure your Python AI development projects are successful. Remember to constantly evaluate and iterate your models to keep up with changing industry trends.
Challenges in Python AI Development
While Python is a powerful tool for AI development, it is not without its challenges. It is important to be aware of these hurdles and strategies for overcoming them to ensure successful projects. Here are some of the common challenges faced in Python AI development:
Data Scarcity
One of the biggest challenges in AI development is finding and preparing high-quality data. This is especially true in cases where there is a scarcity of data. To overcome this, developers can use techniques such as data augmentation or transfer learning to leverage existing data and generate more data for training models.
Model Interpretability
Another challenge in AI development is model interpretability. Deep learning models especially tend to be black boxes, making it difficult to understand how they come to their conclusions. This can be problematic when these models are used to make decisions with significant consequences. To address this, techniques such as LIME and SHAP can be used to provide explanations for model outputs.
Performance Optimization
As AI projects become more complex, performance optimization becomes a key concern. Large datasets and complex models can make the training process incredibly slow. To address this, developers can use techniques such as distributed training or model quantization to speed up the process.
Ethical Considerations
With the increasing use of AI in various industries, there is a growing concern over ethical considerations. Biases in data or models can lead to discriminatory outcomes. To address this, developers can adopt practices such as establishing diverse development teams, responsibly sourcing data, and regularly auditing models for biases.
By understanding and addressing these challenges, developers can ensure successful Python AI development projects that are ethical, high-performing, and interpretable.
Conclusion: Python AI Development for the Future
Python has become the language of choice for AI development, and for good reason. It offers an easy-to-learn syntax, a wide range of libraries, and a supportive community. As we have seen throughout this article, Python is used extensively in various industries to develop intelligent systems that optimize processes, improve decision-making, and enhance user experience.
Whether you are new to AI development or an experienced data scientist, Python provides a powerful platform for building innovative solutions that solve complex problems. Its versatility and accessibility make it an ideal choice for developing and deploying intelligent applications at scale.
Python AI development is crucial for the future of AI and machine learning. With emerging technologies and trends in the field, there is no doubt that Python will continue to play a key role in advancing the capabilities and efficiency of AI systems.
FAQ
Q: What is Python AI development?
A: Python AI development refers to the process of using the Python programming language to build artificial intelligence solutions. It involves implementing and leveraging various AI algorithms, frameworks, and libraries available in Python to create intelligent systems.
Q: Why is Python preferred for AI development?
A: Python is preferred for AI development due to its simplicity, extensive libraries, and community support. It provides a user-friendly syntax that makes it easier to write and understand code. Python also offers a wide range of AI-specific libraries, such as TensorFlow and PyTorch, that facilitate the implementation of complex AI algorithms.
Q: What are some key concepts in Python AI development?
A: Key concepts in Python AI development include machine learning, neural networks, natural language processing, and data preprocessing. Machine learning involves training models on data to make predictions or decisions. Neural networks are a type of machine learning model inspired by the human brain. Natural language processing focuses on understanding and processing human language. Data preprocessing involves cleaning and transforming data to make it suitable for AI algorithms.
Q: What are some popular Python AI frameworks?
A: Some popular Python AI frameworks include TensorFlow, PyTorch, and scikit-learn. These frameworks provide a collection of tools and libraries for building and training AI models. TensorFlow and PyTorch are widely used for deep learning tasks, while scikit-learn is more focused on traditional machine learning algorithms.
Q: How does Python compare to other languages for AI development?
A: Python has several advantages over other languages for AI development. It is known for its readability and ease of use, making it accessible to both beginners and experienced developers. Python also has a large and active community, which means there is extensive support and documentation available. Additionally, Python offers a vast number of libraries specifically designed for AI development, which gives it a competitive edge over languages like R and Java.
Q: What are some industry applications of Python AI development?
A: Python AI development has various applications across industries. In healthcare, Python is used for medical imaging analysis and predicting diseases. In finance, Python is used for algorithmic trading and fraud detection. In marketing, Python is used for customer segmentation and recommendation systems. These are just a few examples, and Python’s versatility allows it to be applied in many other sectors as well.
Q: What are some best practices for Python AI development?
A: Some best practices for Python AI development include properly preparing and preprocessing data, carefully selecting appropriate models and algorithms, tuning hyperparameters to optimize model performance, and considering deployment considerations such as scalability and real-time processing. It is also important to keep up with the latest advancements in AI and continuously update models as new data becomes available.
Q: What are some challenges in Python AI development?
A: Some common challenges in Python AI development include dealing with data scarcity or low-quality data, ensuring model interpretability and explainability, optimizing performance and efficiency, and addressing ethical considerations and biases in AI algorithms. Overcoming these challenges requires careful data handling, robust evaluation techniques, and thoughtful consideration of the societal and ethical implications of AI development.
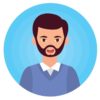
Matthew is a technical author with a passion for software development and a deep expertise in Python. With over 20 years of experience in the field, he has honed his skills as a software development manager at prominent companies such as eBay, Zappier, and GE Capital, where he led complex software projects to successful completion.
Matthew’s deep fascination with Python began two decades ago, and he has been at the forefront of its development ever since. His experience with the language has allowed him to develop a keen understanding of its inner workings, and he has become an expert at leveraging its unique features to build elegant and efficient software solutions.
Matthew’s academic background is rooted in the esteemed halls of Columbia University, where he pursued a Master’s degree in Computer Science.
As a technical author, Matthew is committed to sharing his knowledge with others and helping to advance the field of computer science. His contributions to the scientific computer science community are invaluable, and his expertise in Python development has made him a sought-after speaker and thought leader in the field.