Are you tired of spending hours on manual tasks that should be automated? Look no further than Python scripting solutions. Python offers a wide range of tools and libraries that make scripting tasks more efficient, whether you are an experienced programmer or just starting out.
With expert Python scripting solutions, you can craft scripts with Python that can save you time and effort. By leveraging the power of Python, you can create efficient scripts that automate tasks and streamline workflows.
Why Choose Python for Scripting?
If you’re looking for a language that can handle a variety of scripting tasks, Python is a great choice. Python scripting solutions offer developers a flexible and powerful way to automate tasks and streamline workflows.
Python is known for its simplicity and readability, making it easy for developers of all levels to learn and use. Its clean syntax and large standard library provide developers with a wealth of functionality right out of the box.
Additionally, Python has a strong community of developers who contribute to open-source libraries and frameworks, making it a versatile language for scripting tasks. By leveraging Python for efficient scripting, you can significantly reduce development time and effort.
Python Scripting Basics
Python is a versatile language that allows developers to create powerful scripts for various purposes. Before diving into advanced scripting concepts, let’s cover the basics of Python scripting.
In Python, scripts are written in plain text files with a .py extension. These files can be executed directly or imported as modules into other scripts. Python uses indentation to define code blocks, making it easy to read and understand.
Here is an example of a simple Python script that prints “Hello, world!” to the console:
# Script example
print("Hello, world!")
The above script demonstrates the basic structure of a Python script. The first line is a comment, which starts with the “#” symbol and is used to provide context or explanations to the code. The second line is the actual code that prints the message to the console.
Python also has a built-in interpreter that allows you to execute Python code interactively in a console, without having to write a separate script. This can be useful for quick testing or experimentation.
Here is an example of using the Python interpreter to evaluate an expression:
# Interpreter example
>>> 2 + 2
4
The above example shows how you can use the interpreter to perform basic arithmetic operations, such as addition.
Now that we have covered the basics of Python scripting, we can move on to more advanced concepts and techniques.
Leveraging Python Libraries for Scripting
Python scripting solutions offer a vast array of libraries that can help make your scripting tasks more efficient. These libraries contain pre-built functions and classes that can be utilized to solve common scripting problems.
The standard library in Python already contains a multitude of functionalities that can be used for scripting. For instance, the os module provides functions for working with files and directories in different operating systems. The subprocess module allows you to spawn new processes, connect to their input or output streams, and obtain their return codes. The datetime module provides classes for working with dates and times.
However, sometimes, you may need to use additional libraries to expand your scripting capabilities. One such library is requests, a simple and elegant HTTP library that enables you to send HTTP/1.1 requests easily.
Code Example using “requests” library | Code Example without “requests” library |
---|---|
response = requests.get(“https://www.example.com”)print(response.status_code) print(response.content) print(response.headers) | url = “https://www.example.com”response = urllib.request.urlopen(url) print(response.status) content = response.read() headers = response.info() print(content) print(headers) |
As you can see, using the requests library makes it easier and faster to make an HTTP request and retrieve its response than without it.
Another popular library is numpy, which allows for efficient numerical operations and array manipulation in Python. Its extensive library of mathematical functions and operations make it an excellent choice for scientific computing.
When selecting libraries, make sure to research and evaluate their functionalities to ensure that they meet your scripting needs.
Python Frameworks for Scripting
Python’s powerful library ecosystem isn’t the only tool developers can leverage when crafting their scripts. Python also offers robust frameworks for tackling scripting tasks, making it an optimal choice for efficient scripting. These frameworks provide a structured approach to the development process, often taking care of common tasks such as URL routing, database integration, and authentication, allowing developers to focus on the unique functionalities of their scripts.
1. Flask
Flask is a lightweight yet powerful micro-framework that enables developers to build web applications quickly and efficiently with Python. Its simplicity makes it an ideal choice for scripting tasks that require a high degree of flexibility. Flask can be paired with many extensions, including SQLAlchemy for database integration and Flask-RESTful for API development. Here’s an example of a simple Flask application:
Example:
Code: | from flask import Flask |
---|---|
app = Flask(__name__) | |
@app.route('/') | |
def hello_world(): | |
return 'Hello, World!' | |
if __name__ == '__main__': | |
app.run() |
This script creates a new Flask application and defines a single route that returns “Hello, World!” when accessed.
2. Django
Django is a full-stack web framework that offers a high level of abstraction and a significant amount of “out-of-the-box” functionality. It has a powerful ORM (Object-Relational Mapping) library, which allows developers to write Python code that interacts with databases without having to write SQL queries manually. Django also includes a built-in admin interface, making it simple to perform CRUD (Create, Read, Update, Delete) operations on database records.
Here’s an example of a simple Django application:
Example:
Code: | from django.http import HttpResponse |
---|---|
from django.urls import path | |
from django.conf import settings | |
from django.conf.urls.static import static | |
def hello_world(request): | |
return HttpResponse("Hello, World!") | |
urlpatterns = [ | |
path('', hello_world, name='hello_world'), | |
] | |
if settings.DEBUG: | |
urlpatterns += static(settings.MEDIA_URL, document_root=settings.MEDIA_ROOT) |
This script creates a new Django application and defines a single URL pattern that maps to a view function that returns “Hello, World!” when accessed.
By leveraging these frameworks, developers can save time and effort when crafting their scripts, with both Flask and Django offering streamlined approaches to web development and database integration.
Comparing Python with Other Scripting Languages
While Python is a popular choice for scripting, it’s important to compare it with other languages to determine which language best suits your needs. Let’s compare Python with Ruby and JavaScript, two other popular scripting languages.
Ruby
Ruby is a scripting language that is often compared to Python due to its focus on readability, simplicity, and ease of use. However, Ruby tends to prioritize code maintainability over performance.
Here’s an example of a simple Ruby script:
# Simple Ruby Script
def greeting(name) puts "Hello, #{name}!" end greeting("World")
This script defines a function greeting
that takes a name argument and outputs a greeting message. The output of this script would be:
Hello, World!
JavaScript
JavaScript is a scripting language that is often used for web development. It is also a popular choice for building cross-platform desktop and mobile applications. JavaScript tends to prioritize performance over readability and maintainability.
Here’s an example of a simple JavaScript script:
// Simple JavaScript Script
function greeting(name) { console.log(`Hello, ${name}!`); } greeting("World");
This script defines a function greeting
that takes a name argument and outputs a greeting message. The output of this script would be:
Hello, World!
Overall, Python’s simplicity, readability, and extensive library ecosystem make it a popular choice for scripting. However, depending on your specific use case, Ruby or JavaScript may be better suited for your needs.
Advanced Python Scripting Techniques
Once you have a solid foundation in Python scripting, you can take your skills to the next level by exploring advanced techniques. Here are some topics to consider:
1. Multithreading
Python’s threading module allows you to run multiple threads (smaller units of code) simultaneously within a single process. This can help speed up your scripts by dividing the workload across multiple threads. For example:
import threading def my_function(): # Do some work here # Create threads t1 = threading.Thread(target=my_function) t2 = threading.Thread(target=my_function) # Start threads t1.start() t2.start() # Wait for threads to finish t1.join() t2.join()
2. Asynchronous Programming
Asynchronous programming allows your scripts to continue running while waiting for I/O operations such as file reads or network requests. Python’s asyncio module provides a way to write asynchronous code that is easier to read and maintain.
For example:
import asyncio async def my_function(): # Do some work here # Create an event loop loop = asyncio.get_event_loop() # Run the function asynchronously loop.run_until_complete(my_function())
3. Performance Optimization
When working with large datasets, performance optimization can be crucial for ensuring your scripts run efficiently. Python provides a range of tools for optimizing performance, including the built-in timeit module for measuring code execution time and the PyPy interpreter for faster execution.
For example:
import timeit def my_function(): # Do some work here # Measure execution time print(timeit.timeit(my_function, number=10000))
4. Other Advanced Techniques
Other advanced topics to explore include decorators, generators, and metaclasses. These can help you write cleaner and more efficient code, but require a deeper understanding of Python’s syntax and object-oriented features.
By mastering these advanced techniques, you can take your Python scripts to the next level and tackle even more complex scripting tasks.
Industry Examples of Python Scripting Solutions
Python scripting has proven to be a valuable solution in many industries, showcasing its versatility and ease of use. Here are some examples:
Industry | Use Case |
---|---|
Web Development | Python’s web frameworks, such as Django and Flask, have made it a popular choice for web development. Instagram, for example, is built on Django. |
Scientific Research | Python’s scientific libraries, such as NumPy and SciPy, have made it a top choice for data analysis and scientific computing. NASA uses Python for data analysis and visualization. |
Finance | Python’s libraries, such as Pandas and Scikit-learn, have made it a popular choice for financial analysis and modeling. JPMorgan Chase uses Python for analyzing financial data. |
Machine Learning | Python’s libraries, such as TensorFlow and PyTorch, have made it a top choice for machine learning and artificial intelligence. Google uses Python extensively for machine learning tasks. |
These examples demonstrate how Python scripting can be leveraged for efficient solutions across various industries.
Best Practices for Python Scripting
Python scripting is a powerful tool, but to make the most of it, it’s important to follow best practices. These practices will help you write clean, efficient, and maintainable code.
Code Organization
Organizing your code is essential for readability and maintainability. Use meaningful variable names and organize your code into functions and classes wherever possible. Use comments to explain complex or important sections of your code.
Error Handling
Error handling is an important aspect of scripting. Use try-except blocks to handle exceptions and avoid crashing your script. Use logging to keep track of errors and debug your code effectively.
Testing
Testing is an important part of the development process. Use automated testing frameworks like unittest or pytest to ensure your code is functioning as expected. Write test cases to cover different scenarios and edge cases.
Performance Optimization
Optimizing your code is important for performance and scalability. Use profiling tools like cProfile or line_profiler to identify bottlenecks in your code. Use list comprehensions and generators to reduce memory usage and improve performance.
By following these best practices, you can write effective Python scripts that are efficient, maintainable, and scalable.
Conclusion
Python scripting solutions provide developers with a versatile and efficient way to automate tasks and streamline workflows. By leveraging the extensive library ecosystem and frameworks available in Python, you can craft scripts that save time and effort, whether you are a beginner or an experienced programmer. Remember to follow best practices for clean and effective Python scripts, and stay updated with the latest trends and advancements in Python scripting. With Python at your service, the possibilities for efficient scripting are endless.
FAQ
Q: What is Python scripting?
A: Python scripting is the process of writing scripts in the Python programming language to automate tasks and perform various functions. It is widely used in areas such as web development, data analysis, and scientific research.
Q: How is Python scripting beneficial?
A: Python scripting offers several benefits, including simplicity, readability, and a large standard library that provides a wide range of functionality. It also has a strong community of developers contributing to open-source libraries and frameworks, making it versatile for scripting tasks.
Q: How do I write a Python script?
A: Python scripts are written in plain text files with a .py extension. You can write the script using any text editor and save it with the .py extension. Python scripts can be executed directly or imported as modules into other scripts.
Q: What are Python libraries for scripting?
A: Python libraries are pre-built functions and classes that provide solutions for common scripting problems. For example, the os module offers functions for working with files and directories, while the requests library simplifies HTTP requests.
Q: Are there frameworks available for Python scripting?
A: Yes, Python offers powerful frameworks like Flask and Django for scripting tasks. These frameworks provide a structured way to build web applications with features such as URL routing, database integration, and authentication.
Q: How does Python compare to other scripting languages?
A: Python offers simplicity, readability, and a large library ecosystem, making it a popular choice for scripting. However, it’s important to consider other languages like Ruby and JavaScript and choose the one that best suits your specific scripting needs.
Q: What are some advanced Python scripting techniques?
A: Once you have a foundation in Python scripting, you can explore advanced techniques like multithreading, asynchronous programming, and performance optimization. These techniques can enhance the functionality and performance of your scripts.
Q: Can you provide examples of Python scripting in different industries?
A: Python scripting is widely used in various industries, such as web development, scientific research, and data analysis. It has been used to build websites, automate tasks, analyze large datasets, and solve complex scripting problems in different domains.
Q: What are some best practices for Python scripting?
A: To write clean and effective Python scripts, it’s important to follow best practices. These include organizing your code, handling errors gracefully, and writing tests to ensure the reliability and maintainability of your scripts.
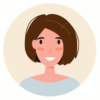
Lydia is a seasoned technical author, well-versed in the intricacies of software development and a dedicated practitioner of Python. With a career spanning 16 years, Lydia has made significant contributions as a programmer and scrum master at renowned companies such as Thompsons, Deloit, and The GAP, where they have been instrumental in delivering successful projects.
A proud alumnus of Duke University, Lydia pursued a degree in Computer Science, solidifying their academic foundation. At Duke, they gained a comprehensive understanding of computer systems, algorithms, and programming languages, which paved the way for their career in the ever-evolving field of software development.
As a technical author, Lydia remains committed to fostering knowledge sharing and promoting the growth of the computer science community. Their dedication to Python development, coupled with their expertise as a programmer and scrum master, positions them as a trusted source of guidance and insight. Through their publications and engagements, Lydia continues to inspire and empower fellow technologists, leaving an indelible mark on the world of scientific computer science.